核心代码
struct Test* insertFromBehind(struct Test* phead,struct Test* new)
{
struct Test* p=phead;
if(phead==NULL)
{
phead=new;
return phead
}
while(p->next!=NULL)
{
p=p->next;
}
p->next=new;
return phead;
}
struct Test* createLink2(struct Test* phead)
{
struct Test* new=NULL;
printf("Please input total node number:\n");
int i=0;
scanf("%d",&i);
while(1)
{
if(i!=0)
{
new=(struct Test*)malloc(sizeof(struct Test));
printf("Please input node:\n");
scan("%d",&(new->data));
}
if(i==0)
{
printf("qiut!\n");
return phead;
}
i--;
phead=insertFromBehind(phead,new);
}
}
示例
#include <stdio.h>
#include <stdlib.h>
struct Test
{
int data;
struct Test* next;
};
void printLink(struct Test* phead)
{
struct Test* point=phead;
while(point!=NULL)
{
printf("%d ",point->data);
point=point->next;
}
putchar('\n');
}
struct Test *insertFromHead(struct Test* phead,struct Test* new)
{
if(phead==NULL)
{
phead=new;
return phead;
}
else
{
new->next=phead;
phead=new;
return phead;
}
}
struct Test* createLink(struct Test* phead)
{
struct Test* new=NULL;
printf("Please input node:\n");
int i=0;
scanf("%d",&i);
while(1)
{
if(i!=0)
{
new=(struct Test*)malloc(sizeof(struct Test));
printf("Please input Node:\n");
scanf("%d",&(new->data));
}
if(i==0)
{
printf("quit!\n");
return phead;
}
i--;
phead=insertFromHead(phead,new);
}
}
struct Test* insertFromBehind(struct Test* phead,struct Test* new)
{
struct Test* p=phead;
if(p==NULL)
{
phead=new;
return phead;
}
while(p->next!=NULL)
{
p=p->next;
}
p->next=new;
return phead;
}
struct Test* createLink2(struct Test* phead)
{
struct Test* new=NULL;
printf("Please input total node number:\n");
int i=0;
scanf("%d",&i);
while(1)
{
if(i!=0)
{
new=(struct Test*)malloc(sizeof(struct Test));
printf("Please input node:\n");
scanf("%d",&(new->data));
}
if(i==0)
{
printf("quit!\n");
return phead;
}
i--;
phead=insertFromBehind(phead,new);
}
}
int main()
{
struct Test* head=NULL;
head=createLink2(head);
struct Test t1={100,NULL};
head=insertFromHead(head,&t1);
printLink(head);
struct Test t2={200,NULL};
head=insertFromBehind(head,&t2);
printLink(head);
return 0;
}
运行结果
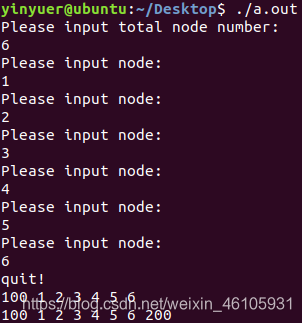
|