程序介绍:
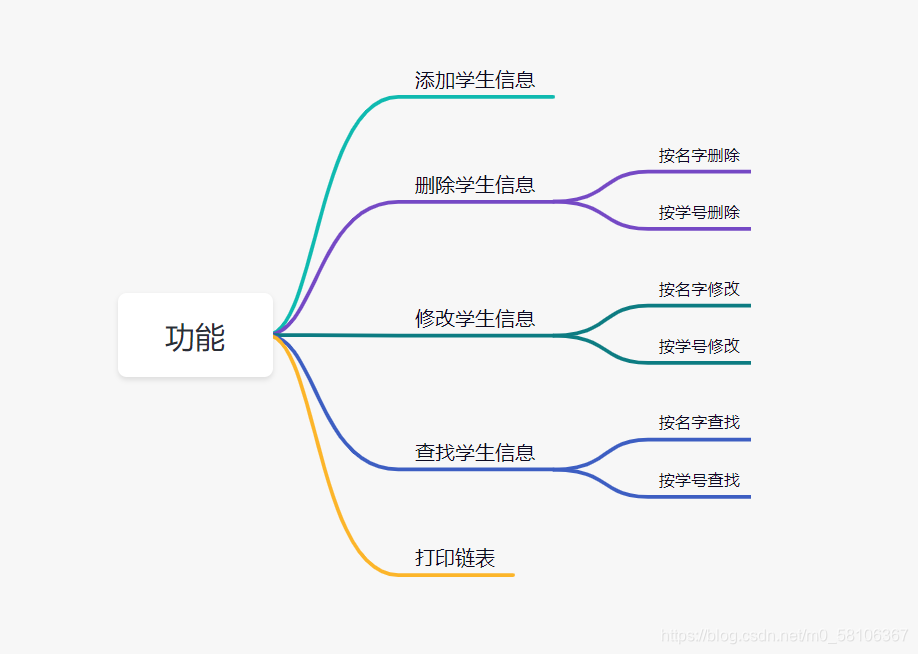
源代码:
#include <iostream>
#include<cstdlib>
using namespace std;
typedef struct Student
{
int score;
char id[124];
char name[124];
char sex[124];
char Class[124];
Student* next;
}Student;
void menu()
{
cout << "1.增加" << endl;
cout << "2.删除" << endl;
cout << "3.修改" << endl;
cout << "4.查找" << endl;
cout << "5.打印" << endl;
cout << "6.退出" << endl;
}
void print_node(Student* node)
{
cout << "该学生的名字是:" << node->name << ",性别是:" << node->sex << ",班级是:" << node->Class << ",学号是:" << node->id << ",分数是:" << node->score << endl;
}
void Add(Student** student)//尾插法
{
system("cls");
Student* node, * temp;
node = (Student*)malloc(sizeof(Student));
cout << "请输入该学生的名字:";
cin >> node->name;
cout << "请输入该学生的学号:";
cin >> node->id;
cout << "请输入该学生的性别:";
cin >> node->sex;
cout << "请输入该学生的班级:";
cin >> node->Class;
cout << "请输入该学生的成绩:";
cin >> node->score;
if (*student)
{
temp = *student;
while (temp->next)
{
temp = temp->next;
}
temp->next = node;
node->next = NULL;
}
else
{
*student = node;
node->next = NULL;
}
}
void Delete_name(Student** student)
{
system("cls");
cout << "请输入要删除的学生的名字:";
string get;
cin >> get;
if (*student == NULL)
{
cout << "很抱歉,链表为空!" << endl;
}
else
{
Student* left = *student;
Student* right = left->next;
if (left->name == get)
{
cout << "该生的信息如下:" << endl;
print_node(left);
left = left->next;
*student = left;
cout << "删除成功" << endl;
}
else
{
while (right)
{
if (right->name == get)
{
cout << "该生的信息如下:" << endl;
print_node(left);
left->next = right->next;
right = NULL;
cout << "删除成功" << endl;
return;
}
else
{
right = right->next;
left = left->next;
}
}
if (right == NULL)
{
cout << "很抱歉,没有找到该学生的数据!" << endl;
}
}
}
}
void Delete_id(Student** student)
{
system("cls");
cout << "请输入要删除的学生的学号:";
string get;
cin >> get;
if (*student == NULL)
{
cout << "很抱歉,链表为空!" << endl;
}
else
{
Student* left = *student;
Student* right = left->next;
if (left->id == get)
{
cout << "该生的信息如下:" << endl;
print_node(left);
left = left->next;
*student = left;
cout << "删除成功" << endl;
}
else
{
while (right)
{
if (right->id == get)
{
cout << "该生的信息如下:" << endl;
print_node(right);
left->next = right->next;
right = NULL;
cout << "删除成功" << endl;
return;
}
else
{
right = right->next;
left = left->next;
}
}
if (right == NULL)
{
cout << "很抱歉,没有找到该学生的数据!" << endl;
}
}
}
}
void Modify_name(Student** student)
{
system("cls");
cout << "请输入要修改的学生的姓名:";
string get;
cin >> get;
Student* temp;
temp = (Student*)malloc(sizeof(Student));
int count = 2;
if (*student == NULL)
{
cout << "很抱歉,链表为空!" << endl;
}
else
{
Student* left = *student;
Student* right = left->next;
if (left->name == get)
{
cout << "该学生在第" << "1个结点," << "该生的信息如下:" << endl;
print_node(left);
cout << "请输入修改以后的名字:";
cin >> temp->name;
cout << "请输入修改以后的性别:";
cin >> temp->sex;
cout << "请输入修改以后的班级:";
cin >> temp->Class;
cout << "请输入修改以后的学号:";
cin >> temp->id;
cout << "请输入修改以后的成绩:";
cin >> temp->score;
*student = temp;
temp->next = right;
right = NULL;
cout << "修改成功" << endl;
return;
}
else
{
while (right)
{
if (right->name == get)
{
cout << "该学生在第" << count << "个结点," << "该生的信息如下:" << endl;
print_node(right);
cout << "请输入修改以后的名字:";
cin >> temp->name;
cout << "请输入修改以后的性别:";
cin >> temp->sex;
cout << "请输入修改以后的班级:";
cin >> temp->Class;
cout << "请输入修改以后的学号:";
cin >> temp->id;
cout << "请输入修改以后的成绩:";
cin >> temp->score;
left->next = temp;
temp->next = right->next;
right = NULL;
cout << "修改成功" << endl;
return;
}
else
{
right = right->next;
left = left->next;
count++;
}
}
if (right == NULL)
{
cout << "很抱歉,没有找到该学生的数据!" << endl;
}
}
}
}
void Modify_id(Student** student)
{
system("cls");
cout << "请输入要修改的学生的学号:";
string get;
cin >> get;
Student* temp;
temp = (Student*)malloc(sizeof(Student));
int count = 2;
if (*student == NULL)
{
cout << "很抱歉,链表为空!" << endl;
}
else
{
Student* left = *student;
Student* right = left->next;
if (left->id == get)
{
cout << "该学生在第" << "1个结点," << "该生的信息如下:" << endl;
print_node(left);
cout << "请输入修改以后的名字:";
cin >> temp->name;
cout << "请输入修改以后的性别:";
cin >> temp->sex;
cout << "请输入修改以后的班级:";
cin >> temp->Class;
cout << "请输入修改以后的学号:";
cin >> temp->id;
cout << "请输入修改以后的成绩:";
cin >> temp->score;
*student = temp;
temp->next = right;
right = NULL;
cout << "修改成功" << endl;
return;
}
else
{
while (right)
{
if (right->id == get)
{
cout << "该学生在第" << count << "个结点," << "该生的信息如下:" << endl;
print_node(right);
cout << "请输入修改以后的名字:";
cin >> temp->name;
cout << "请输入修改以后的性别:";
cin >> temp->sex;
cout << "请输入修改以后的班级:";
cin >> temp->Class;
cout << "请输入修改以后的学号:";
cin >> temp->id;
cout << "请输入修改以后的成绩:";
cin >> temp->score;
left->next = temp;
temp->next = right->next;
right = NULL;
cout << "修改成功" << endl;
return;
}
else
{
right = right->next;
left = left->next;
count++;
}
}
if (right == NULL)
{
cout << "很抱歉,没有找到该学生的数据!" << endl;
}
}
}
}
void Search_name(Student* student)
{
system("cls");
cout << "请输入要查找的学生的名字:";
string get;
cin >> get;
int count = 1;
Student* node = student;
if (student == NULL)
{
cout << "很抱歉,链表为空!" << endl;
}
else
{
while (node)
{
if (node->name == get)
{
cout << "该学生在第" << count << "个结点" << endl;
print_node(node);
break;
}
count++;
node = node->next;
}
if (node == NULL)
{
cout << "很抱歉,未找到该学生!" << endl;
}
}
}
void Search_id(Student* student)
{
system("cls");
cout << "请输入要查找的学生的学号:";
string get;
cin >> get;
int count = 1;
Student* node = student;
if (student == NULL)
{
cout << "很抱歉,链表为空!" << endl;
}
else
{
while (node)
{
if (node->id == get)
{
cout << "该学生在第" << count << "个结点" << endl;
print_node(node);
break;
}
count++;
node = node->next;
}
if (node == NULL)
{
cout << "很抱歉,未找到该学生!" << endl;
}
}
}
void Print(Student* student)
{
system("cls");
Student* node = student;
int count = 1;
if (student == NULL)
{
cout << "很抱歉,链表为空!" << endl;
}
else
{
while (node)
{
print_node(node);
node = node->next;
count++;
}
}
}
int main()
{
Student* student = NULL;
while (1)
{
menu();
int ch;
cout << "请输入:";
cin >> ch;
if (ch == 1)
{
Add(&student);
}
else if (ch == 2)
{
cout << "1.按名字删除" << endl;
cout << "2.按学号删除" << endl;
cout << "请选择你要删除的方式:";
int get;
cin >> get;
if (get == 1)
{
Delete_name(&student);
}
else if(get==2)
{
Delete_id(&student);
}
}
else if (ch == 3)
{
cout << "1.按名字修改" << endl;
cout << "2.按学号修改" << endl;
cout << "请选择你要修改的方式:";
int get;
cin >> get;
if (get == 1)
{
Modify_name(&student);
}
else if (get == 2)
{
Modify_id(&student);
}
}
else if (ch == 4)
{
cout << "1.按名字查找" << endl;
cout << "2.按学号查找" << endl;
cout << "请选择你要查找的方式:";
int get;
cin >> get;
if (get == 1)
{
Search_name(student);
}
else if (get == 2)
{
Search_id(student);
}
}
else if (ch == 5)
{
Print(student);
}
else if (ch == 6)
{
return 0;
}
else
{
cout << "你的输入有误,请重新输入!" << endl;
}
}
return 0;
}
【注】:本程序全部由本人编写,未借鉴任何资料,若有雷同,纯属巧合
|