单链表的初始化 插入 打印
初始化一个单链表
#include <stdio.h>
#include <stdlib.h>
struct Node{
int data;
struct Node *next;
};
数据域存放数据 指针域存放的是下一个数据的地址
创建带头结点
struct Node *createList(){
struct Node *headNode;
headNode = (struct Node*)malloc(sizeof(struct Node));
headNode -> next = NULL;
return headNode;
}
创建结点
struct Node *createNode(int data){
struct Node *newNode = (struct Node*)malloc(sizeof(struct Node));
newNode -> data = data;
newNode -> next = NULL;
return newNode;
}
插入函数:向结点插入数据
void insertNodeByHead(struct Node *headNode,int data){
struct Node *newNode = createNode(data);
newNode->next = headNode->next;
headNode->next = newNode;
}
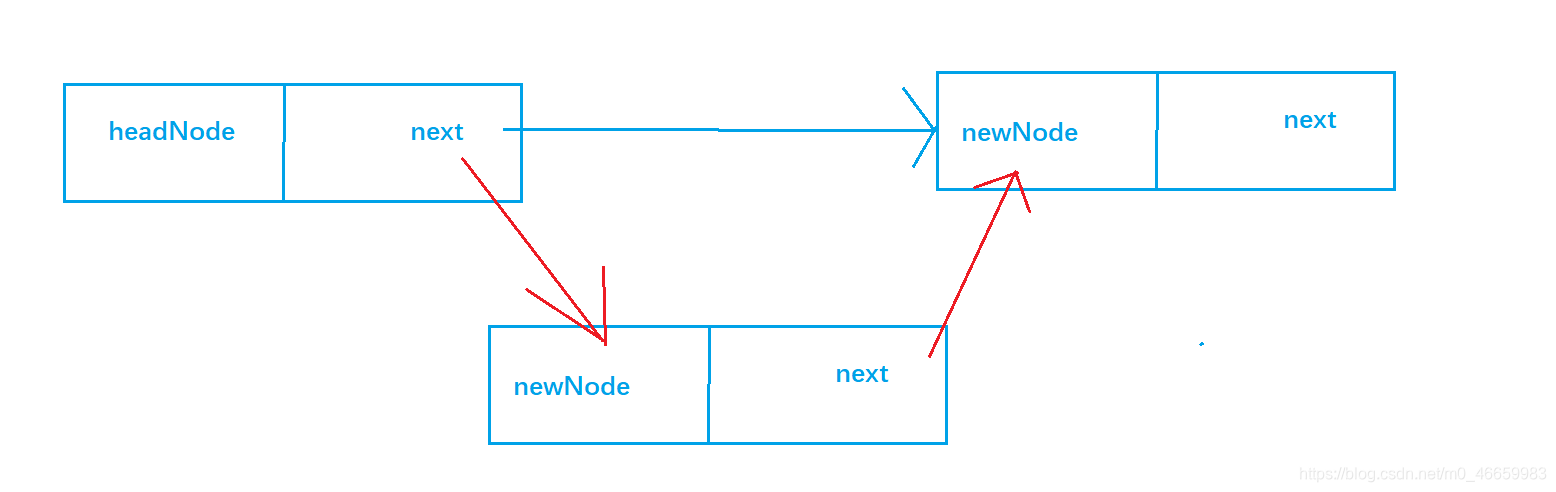
打印单链表
void printList(struct Node *headNode){
struct Node *pMove = headNode->next;
while(pMove){
printf("%d",pMove->data);
pMove = pMove->next;
}
printf("\n");
}
主函数
int main (){
struct Node *List = createList();
insertNodeByHead(List,1);
insertNodeByHead(List,2);
insertNodeByHead(List,3);
printList(List);
system("pause");
return 0;
}
|