一、集合的概述及和数组的缺点
集合、数组都是对多个数据进行存储操作的结构,简称Java容器
说明: 此时的存储,主要指的是内存层面的存储,不涉及持久化的存储(.txt,.jpg,.avi,数据库中)
数组在存储多个数据方面的特点:
?????????? 1.一旦初始化以后,其长度就确定了。
??????????? 2.数组一旦定义好,其元素的类型也就确定了。我们也就只能操作指定类型。
??????????? 比如:String[] arr,int[] arr1,Object[] arr2
数组在存储多个数据方面的缺点:
???? 1. 一旦初始化以后,其长度就不可修改
???? 2.数组中提供的方法非常有限,对于添加、删除、插入数据等操作,非常不便且效率不高
???? 3.获取数组中实际元素的个数的需求,数组没有现成的属性或方法可用
???? 4.数组存储数据的特点: 有序、可重复。对于无序、不可重复的需求,不能满足。
二、集合的框架
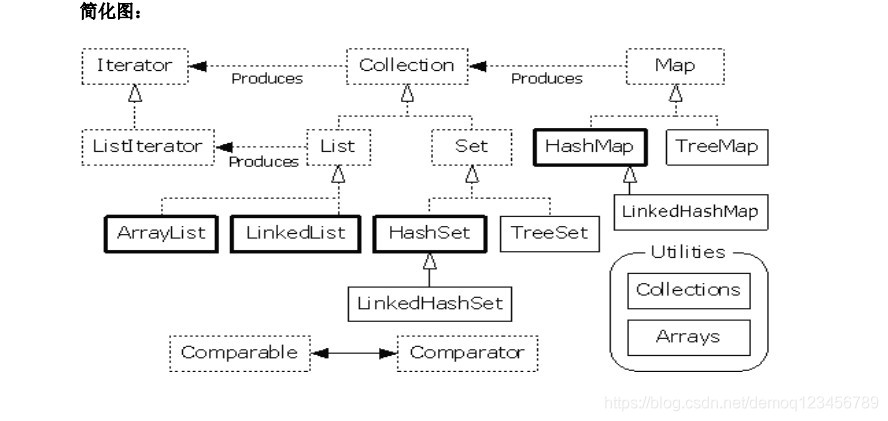
?
Collection 接口的接口 对象的集合(单列集合) ├——-List 接口:元素按进入先后有序保存,可重复 │—————-├ LinkedList 接口实现类, 链表, 插入删除, 没有同步, 线程不安全 │—————-├ ArrayList 接口实现类, 数组, 随机访问, 没有同步, 线程不安全 │—————-└ Vector 接口实现类 数组, 同步, 线程安全 │ ———————-└ Stack 是Vector类的实现类 └——-Set 接口: 仅接收一次,不可重复,并做内部排序 ├—————-└HashSet 使用hash表(数组)存储元素 │————————└ LinkedHashSet 链表维护元素的插入次序 └ —————-TreeSet 底层实现为二叉树,元素排好序
Map 接口 键值对的集合 (双列集合) ├———Hashtable 接口实现类, 同步, 线程安全 ├———HashMap 接口实现类 ,没有同步, 线程不安全- │—————–├ LinkedHashMap 双向链表和哈希表实现 │—————–└ WeakHashMap ├ ——–TreeMap 红黑树对所有的key进行排序 └———IdentifyHashMap
三、集合的常用方法?
Collection collection=new ArrayList();
//将元素添加到集合collection中
collection.add("AA");
collection.add("BB");
collection.add(123);
collection.add(new Date());
//获取集合的元素个数
System.out.println(collection.size());
//判断集合是否包含某个元素
boolean contains=collection.contains("AA");
System.out.println(contains);
//从collection中移除collection1的所有元素
Collection collection1= Arrays.asList(123,"AA");
collection.removeAll(collection1);
System.out.println(collection);
//清空集合元素
collection.clear();
//判断集合是否为空
System.out.println(collection.isEmpty());
List<String> list=new ArrayList<String>();
//将元素添加到集合collection中
list.add("AA");
list.add("BB");
list.add("123");
//第一种遍历方法使用 For-Each 遍历 List
for (String str : list) { //也可以改写 for(int i=0;i<list.size();i++) 这种形式
System.out.println(str);
}
//第二种遍历,把链表变为数组相关的内容进行遍历
String[] strArray=new String[list.size()];
list.toArray(strArray);
for(String str:strArray){
System.out.println(str);
}
//第三种遍历 使用迭代器进行相关遍历
Iterator iterator=list.iterator();
while (iterator.hasNext()){//判断下一个元素之后有值
System.out.println(iterator.next());
}
public static void main(String[] args) {
Map<String, String> map = new HashMap<String, String>();
map.put("1", "value1");
map.put("2", "value2");
map.put("3", "value3");
//第一种:普遍使用,二次取值
System.out.println("通过Map.keySet遍历key和value:");
for (String key : map.keySet()) {
System.out.println("key= "+ key + " and value= " + map.get(key));
}
//第二种
System.out.println("通过Map.entrySet使用iterator遍历key和value:");
Iterator<Map.Entry<String, String>> it = map.entrySet().iterator();
while (it.hasNext()) {
Map.Entry<String, String> entry = it.next();
System.out.println("key= " + entry.getKey() + " and value= " + entry.getValue());
}
//第三种:推荐,尤其是容量大时
System.out.println("通过Map.entrySet遍历key和value");
for (Map.Entry<String, String> entry : map.entrySet()) {
System.out.println("key= " + entry.getKey() + " and value= " + entry.getValue());
}
//第四种
System.out.println("通过Map.values()遍历所有的value,但不能遍历key");
for (String v : map.values()) {
System.out.println("value= " + v);
}
}
?
|