Josepfu 约瑟夫问题实现
什么是约瑟夫问题:

问题分析 :
1,表示n个人环坐做在一起使用什么样子的数据结构, 数组?链表?
2.如何找到第K个结点,如何移动找到每隔m个要操作的结点
3. 最后将结点移动出去
在这次我选择数据结构为环形链表:因为形象直接,增加删除快速
分析图如下: 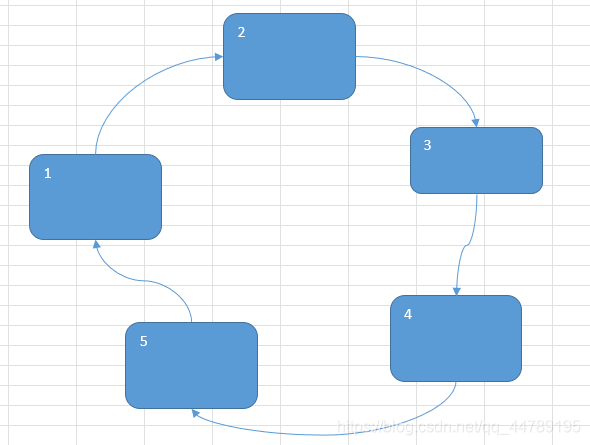
思路分析 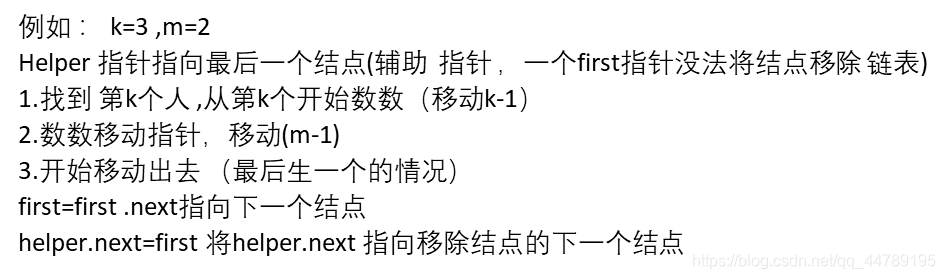 需要一个辅助结点helper指向first结点前一个元素 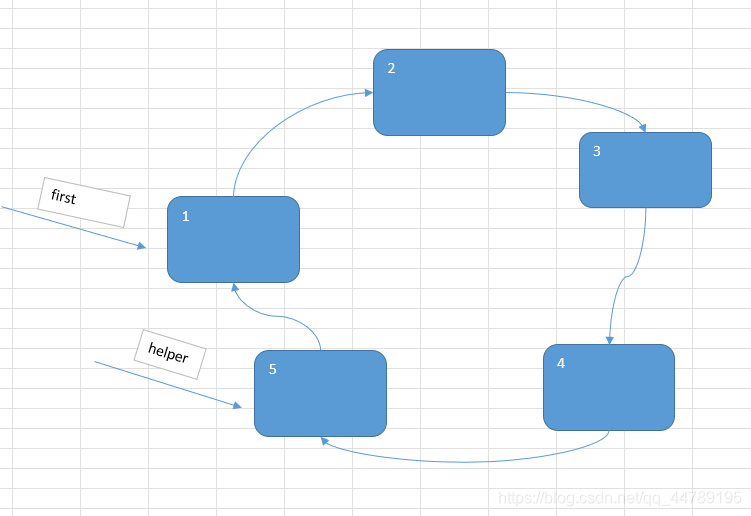 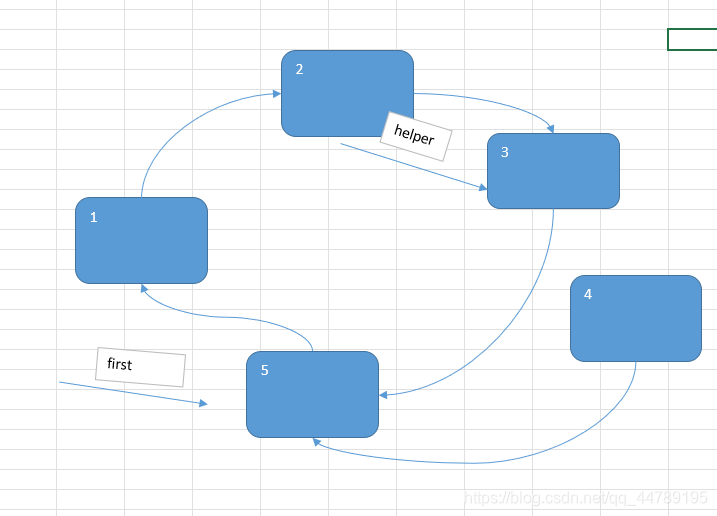 代码实现
孩子结点
public class BoyNode {
private int no;
private BoyNode next;
public BoyNode(int no){
this.no=no;
}
public int getNo() {
return no;
}
public void setNo(int no) {
this.no = no;
}
public BoyNode getNext() {
return next;
}
public void setNext(BoyNode next) {
this.next = next;
}
@Override
public String toString() {
return "BoyNode{" +
"no=" + no +
'}';
}
}
循环链表
public class CircleSingleLinkedList {
private BoyNode first = null;
private BoyNode cur = null;
public void add(int nums) {
if (nums < 1) {
throw new RuntimeException("num值不能小于1");
}
for (int num = 1; num <= nums; num++) {
BoyNode boy = new BoyNode(num);
if (first == null) {
first = boy;
boy.setNext(boy);
cur = boy;
} else {
cur.setNext(boy);
cur = boy;
cur.setNext(first);
}
}
}
public void josepfu(int k, int m) {
if (k < 1 || m < 0 || k > size()) {
throw new RuntimeException("k,m 不合理");
}
BoyNode helper = this.getLastNode();
for (int i = 0; i < k - 1; i++) {
first = first.getNext();
helper = helper.getNext();
}
while (true) {
if (first.getNext() == first) {
System.out.print("最后一个出列" + first);
return;
}
for (int j = 0; j < m - 1; j++) {
first = first.getNext();
helper = helper.getNext();
}
System.out.print(first + "出列了"+",\t");
first = first.getNext();
helper.setNext(first);
}
}
private BoyNode getLastNode() {
if (first == null) {
System.out.println("循环链表为空");
}
BoyNode end = first;
do {
end = end.getNext();
} while (end.getNext() != first);
return end;
}
public int size() {
int count = 0;
if (first == null) {
return count;
}
cur = first;
do {
count++;
cur = cur.getNext();
} while (cur != first);
cur = null;
return count;
}
public void printList() {
if (first == null) {
return;
}
cur = first;
do {
System.out.println(cur);
cur = cur.getNext();
} while (cur != first);
}
}
运行代码
public class CircleLinkedListTest {
public static void main(String[] args) {
CircleSingleLinkedList list = new CircleSingleLinkedList();
list.add(5);
list.josepfu(3,2);
}
结果显示 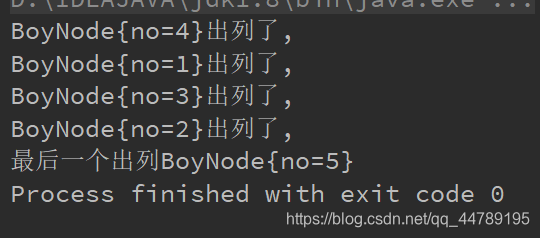
|