#include<bits/stdc++.h>
using namespace std;
const int N=1e5+10;
int e[N],ne[N],head=-1,idx;
void add(int k,int x){
e[idx]=x;
ne[idx]=ne[k];
ne[k]=idx++;
}
void add_head(int x){
e[idx]=x;
ne[idx]=head;
head=idx++;
}
void remove(int k){
ne[k]=ne[ne[k]];
}
int main(){
int n;
scanf("%d",&n);
while(n--){
char c;
scanf(" %c",&c);
if(c=='I'){
int k,x;
scanf("%d%d",&k,&x);
add(k-1,x);
}
else if(c=='D'){
int k;
scanf("%d",&k);
if(!k) head=ne[head];
else remove(k-1);
}
else{
int x;
scanf("%d",&x);
add_head(x);
}
}
for(int i=head;i!=-1;i=ne[i]) cout<<e[i]<<' ';
return 0;
}
#include<iostream>
using namespace std;
const int N = 1e5 + 10;
int m;
int e[N], l[N], r[N];
int idx;
void init()
{
l[1] = 0, r[0] = 1;
idx = 2;
}
void add(int k, int x)
{
e[idx] = x;
r[idx] = r[k];
l[idx] = k;
l[r[k]] = idx;
r[k] = idx;
idx++;
}
void remove(int k)
{
r[l[k]] = r[k];
l[r[k]] = l[k];
}
int main(void)
{
ios::sync_with_stdio(false);
cin >> m;
init();
while(m--)
{
string op;
cin >> op;
int k, x;
if(op=="R")
{
cin >> x;
add(l[1], x);
}
else if(op=="L")
{
cin >> x;
add(0, x);
}
else if(op=="D")
{
cin >> k;
remove(k + 1);
}
else if(op=="IL")
{
cin >> k >> x;
add(l[k + 1], x);
}
else
{
cin >> k >> x;
add(k + 1, x);
}
}
for(int i = r[0]; i != 1; i = r[i]) cout << e[i] << ' ';
return 0;
}
#include<bits/stdc++.h>
using namespace std;
const int N=1e5+10;
char str[N];
int son[N][26],cnt[N],idx;
void insert(char str[]){
int p=0;
for(int i=0;str[i];i++){
int u=str[i]-'a';
if(!son[p][u]) son[p][u]=++idx;
p=son[p][u];
}
cnt[p]++;
}
int query(char str[]){
int p=0;
for(int i=0;str[i];i++){
int u=str[i]-'a';
if(!son[p][u]) return 0;
p=son[p][u];
}
return cnt[p];
}
int main(){
int n;
scanf("%d",&n);
while(n--){
char c[2];
scanf("%s%s",c,str);
if(c[0]=='I') insert(str);
else printf("%d\n",query(str));
}
return 0;
}
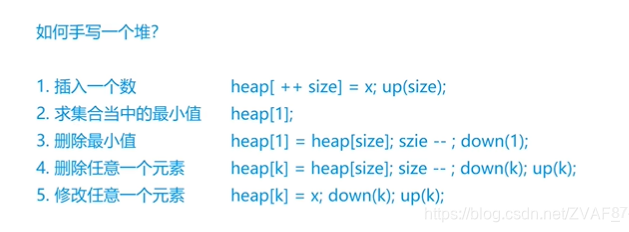
#include <iostream>
#include <algorithm>
#include <string.h>
using namespace std;
const int N = 100010;
int h[N], ph[N], hp[N], cnt;
void heap_swap(int a, int b)
{
swap(ph[hp[a]],ph[hp[b]]);
swap(hp[a], hp[b]);
swap(h[a], h[b]);
}
void down(int u)
{
int t = u;
if (u * 2 <= cnt && h[u * 2] < h[t]) t = u * 2;
if (u * 2 + 1 <= cnt && h[u * 2 + 1] < h[t]) t = u * 2 + 1;
if (u != t)
{
heap_swap(u, t);
down(t);
}
}
void up(int u)
{
while (u / 2 && h[u] < h[u / 2])
{
heap_swap(u, u / 2);
u >>= 1;
}
}
int main()
{
int n, m = 0;
scanf("%d", &n);
while (n -- )
{
char op[5];
int k, x;
scanf("%s", op);
if (!strcmp(op, "I"))
{
scanf("%d", &x);
cnt ++ ;
m ++ ;
ph[m] = cnt, hp[cnt] = m;
h[cnt] = x;
up(cnt);
}
else if (!strcmp(op, "PM")) printf("%d\n", h[1]);
else if (!strcmp(op, "DM"))
{
heap_swap(1, cnt);
cnt -- ;
down(1);
}
else if (!strcmp(op, "D"))
{
scanf("%d", &k);
k = ph[k];
heap_swap(k, cnt);
cnt -- ;
up(k);
down(k);
}
else
{
scanf("%d%d", &k, &x);
k = ph[k];
h[k] = x;
up(k);
down(k);
}
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
const int N=1e5+10;
int stk[N],tt;
int main(){
int m;
cin>>m;
while(m--){
string s;
cin>>s;
if(s=="push"){
int x;
cin>>x;
stk[++tt]=x;
}
else if(s=="pop") tt--;
else if(s=="empty") cout<<(tt ? "NO":"YES")<<'\n';
else cout<<stk[tt]<<'\n';
}
return 0;
}
O(n),最常用应用找数左边(右边)第一个比它小(大)的数
#include<bits/stdc++.h>
using namespace std;
const int N=1e5+10;
int stk[N],tt;
int main(){
int n;
cin>>n;
while(n--){
int x;
cin>>x;
while(tt&&stk[tt]>=x) tt--;
if(tt) cout<<stk[tt]<<' ';
else cout<<-1<<' ';
stk[++tt]=x;
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
const int N=1e5+10;
int que[N],tt=-1,hh;
int main(){
int m;
cin>>m;
while(m--){
string s;
cin>>s;
if(s=="push"){
int x;
cin>>x;
que[++tt]=x;
}
else if(s=="empty") cout<<(hh<=tt ? "NO" : "YES")<<endl;
else if(s=="pop") hh++;
else cout<<que[hh]<<endl;
}
return 0;
}
我们从左到右扫描整个序列,用一个队列来维护最近 kk 个元素; 如果用暴力来做,就是每次都遍历一遍队列中的所有元素,找出最小值即可,但这样时间复杂度就变成 O(nk)O(nk) 了; 然后我们可以发现一个性质: 如果队列中存在两个元素,满足 a[i] >= a[j] 且 i < j,那么无论在什么时候我们都不会取 a[i] 作为最小值了,所以可以直接将 a[i] 删掉; 此时队列中剩下的元素严格单调递增,所以队头就是整个队列中的最小值,可以用 O(1)O(1) 的时间找到; 为了维护队列的这个性质,我们在往队尾插入元素之前,先将队尾大于等于当前数的元素全部弹出即可; 这样所有数均只进队一次,出队一次,所以时间复杂度是 O(n)O(n) 的。
#include<bits/stdc++.h>
using namespace std;
const int N=1e6+10;
int q[N],a[N];
int main(){
ios::sync_with_stdio(false);
cin.tie(0);cout.tie(0);
int n,k;
cin>>n>>k;
for(int i=0;i<n;i++) cin>>a[i];
int tt=-1,hh=0;
for(int i=0;i<n;i++){
if(hh<=tt&&i-q[hh]+1>k) hh++;
while(hh<=tt&&a[q[tt]]>=a[i]) tt--;
q[++tt]=i;
if(i>=k-1) cout<<a[q[hh]]<<' ';
}
cout<<'\n';
tt=-1,hh=0;
for(int i=0;i<n;i++){
if(hh<=tt&&i-k+1>q[hh]) hh++;
while(hh<=tt&&a[q[tt]]<=a[i]) tt--;
q[++tt]=i;
if(i>=k-1) cout<<a[q[hh]]<<' ';
}
return 0;
}
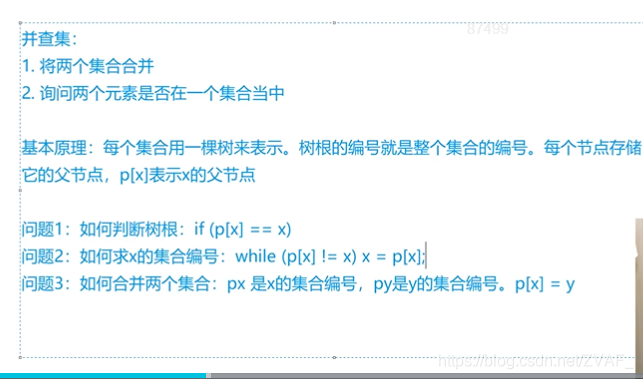
#include<bits/stdc++.h>
using namespace std;
const int N=100010;
int n,m;
int p[N],cnt[N];
int find(int x){
if(p[x]!=x) p[x]=find(p[x]);
return p[x];
}
int main(){
scanf("%d %d",&n,&m);
for(int i=1;i<=n;i++){
p[i]=i;
cnt[i]=1;
}
while(m--){
int a,b;
char op[5];
scanf("%s",op);
if(op[0]=='C'){
scanf("%d%d",&a,&b);
if(find(a)==find(b)) continue;
cnt[find(b)]+=cnt[find(a)];
p[find(a)]=find(b);
}
else if(op[1]=='1'){
scanf("%d%d",&a,&b);
if(find(a)==find(b)) puts("Yes");
else puts("No");
}
else{
scanf("%d",&a);
printf("%d\n",cnt[find(a)]);
}
}
return 0;
}
#include <iostream>
using namespace std;
const int N = 100010, M = 1000010;
int n, m;
int ne[N];
char s[M], p[N];
int main()
{
cin >> n >> p + 1 >> m >> s + 1;
for (int i = 2, j = 0; i <= n; i ++ )
{
while (j && p[i] != p[j + 1]) j = ne[j];
if (p[i] == p[j + 1]) j ++ ;
ne[i] = j;
}
for (int i = 1, j = 0; i <= m; i ++ )
{
while (j && s[i] != p[j + 1]) j = ne[j];
if (s[i] == p[j + 1]) j ++ ;
if (j == n)
{
printf("%d ", i - n);
j = ne[j];
}
}
return 0;
}
哈希表
840. 模拟散列表
#include<bits/stdc++.h>
using namespace std;
const int N=100003;
int h[N],e[N],ne[N],idx;
void insert(int x){
int k=(x%N+N)%N;
e[idx]=x;
ne[idx]=h[k];
h[k]=idx++;
}
bool find(int x){
int k=(x%N+N)%N;
for(int i=h[k];i!=-1;i=ne[i]){
if(e[i]==x) return true;
}
return false;
}
int main(){
int n;
cin>>n;
memset(h,-1,sizeof h);
while(n--){
char op[2];
int x;
scanf("%s%d",op,&x);
if(op[0]=='I') insert(x);
else{
if(find(x)) printf("Yes\n");
else puts("No");
}
}
return 0;
}
841. 字符串哈希
#include <iostream>
#include <algorithm>
using namespace std;
typedef unsigned long long ULL;
const int N = 100010, P = 131;
int n, m;
char str[N];
ULL h[N], p[N];
ULL get(int l, int r)
{
return h[r] - h[l - 1] * p[r - l + 1];
}
int main()
{
scanf("%d%d", &n, &m);
scanf("%s", str + 1);
p[0] = 1;
for (int i = 1; i <= n; i ++ )
{
h[i] = h[i - 1] * P + str[i];
p[i] = p[i - 1] * P;
}
while (m -- )
{
int l1, r1, l2, r2;
scanf("%d%d%d%d", &l1, &r1, &l2, &r2);
if (get(l1, r1) == get(l2, r2)) puts("Yes");
else puts("No");
}
return 0;
}
|