数据结构与算法学习|REVIEW &1
Day 1
Part 1 Learned
数据结构——(以下仅为联想框架) 1.顺序表与链表(逻辑结构/物理结构) 2.栈 stack 3.队列 queue 4.标准库STL(1)—神仙库 stack queue vector list set map
Part 2 Check
1-1 (https://onlinejudge.u-aizu.ac.jp/problems/ALDS1_1_D)
Maximum Profit
Attention: (1)先判断最小的在哪里 (2)再在后面找最大的
#include<iostream>
#include<limits.h>
#include<time.h>
using namespace std;
const int N=2e5;
int p[N];
int main(){
int n;
cin>>n;
for(int i=0;i<n;i++){
cin>>p[i];
}
int minval=p[0];
int maxval=INT_MIN;
for(int i=1;i<n;i++){
maxval=max(maxval,p[i]-minval);
minval=min(minval,p[i]);
}
cout<<maxval<<endl;
return 0;
}
1-2 (https://onlinejudge.u-aizu.ac.jp/courses/lesson/1/ALDS1/3/ALDS1_3_C)
Doubly Linked List
Attention: (1)typedef的用法区分(代码区) (2)环形链表的结构体写法风格——过程要理解
#include<iostream>
#include<cstdio>
#include<cstring>
#include<cstdlib>
using namespace std;
struct Node{
int key;
Node *prev;
Node *next;
};
Node *head;
Node *listSearch(int key){
Node *cur=head->next;
while(cur!=head&&cur->key!=key){
cur= cur->next;
}
return cur;
}
void init(){
head=new Node;
head->prev=head;
head->next=head;
}
void printlist(){
Node *cur =head->next;
int isf=0;
while(1){
if(cur==head)break;
if(isf++ >0)printf(" ");
printf("%d",cur->key);
cur=cur->next;
}
printf("\n");
}
void insert(int key){
Node *x=new Node;
x->key=key;
x->next=head->next;
head->next->prev=x;
head->next=x;
x->prev=head;
}
void deleteNode(Node *t){
if(t==head)return;
t->prev->next=t->next;
t->next->prev=t->prev;
free(t);
}
void deleteFirst(){
deleteNode(head->next);
}
void deleteLast(){
deleteNode(head->prev);
}
void deletekey(int key){
deleteNode(listSearch(key));
}
int main(){
int key,n,i;
char com[20];
scanf("%d",&n);
init();
for(i=0;i<n;i++){
scanf("%s%d",com,&key);
if(com[0]=='i'){insert(key);}
else if(com[0]=='d'){
if(strlen(com)>6){
if(com[6]=='F')deleteFirst();
else if(com[6]=='L')deleteLast();
}
else{
deletekey(key);
}
}
}
printlist();
return 0;
}
1-3 (https://onlinejudge.u-aizu.ac.jp/courses/lesson/1/ALDS1/3/ALDS1_3_A)
逆波兰数-stack
Attention: (1)stack的STL函数库的调用 (2)注意多位数字,char不太行得通,走string,但是也要注意string转换int型。-> "sstream"函数
#include<iostream>
#include<stack>
#include<sstream>
using namespace std;
bool isDegital(string str) {
for (int i = 0;i < str.size();i++) {
if (str.at(i) == '-' && str.size() > 1)
continue;
if (str.at(i) > '9' || str.at(i) < '0')
return false;
}
return true;
}
int main(){
stack<int> s;
string c;
int a,b;
int p;
while(cin>>c){
if(isDegital(c)){
stringstream ss;
ss<<c;
int temp;
ss>>temp;
s.push(temp);
}
if(c=="+"){
a=s.top();
s.pop();
b=s.top();
s.pop();
p=a+b;
s.push(p);
}
if(c=="-"){
a=s.top();
s.pop();
b=s.top();
s.pop();
p=b-a;
s.push(p);
}
if(c=="*"){
a=s.top();
s.pop();
b=s.top();
s.pop();
p=b*a;
s.push(p);
}
if(cin.get()=='\n') break;
}
cout<<s.top()<<endl;
return 0;
}
1-4(https://onlinejudge.u-aizu.ac.jp/courses/lesson/1/ALDS1/3/ALDS1_3_B)
单处理机,时间片轮转法——queue
(感觉有点意思的一道题)
Attention: (1)单片机的处理过程。 (2)队列FIFO,指针的指向过程,注意最后带序号输出。
时间轮转法理解part:

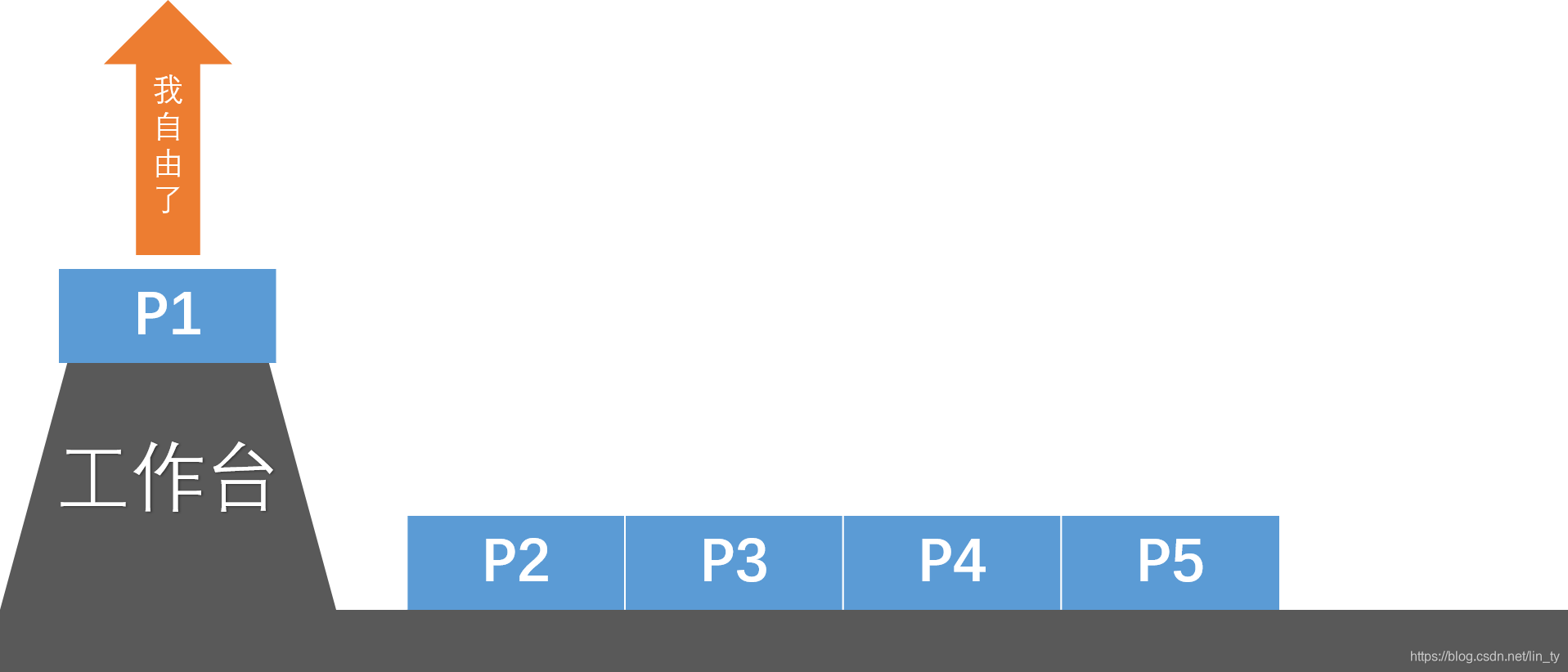 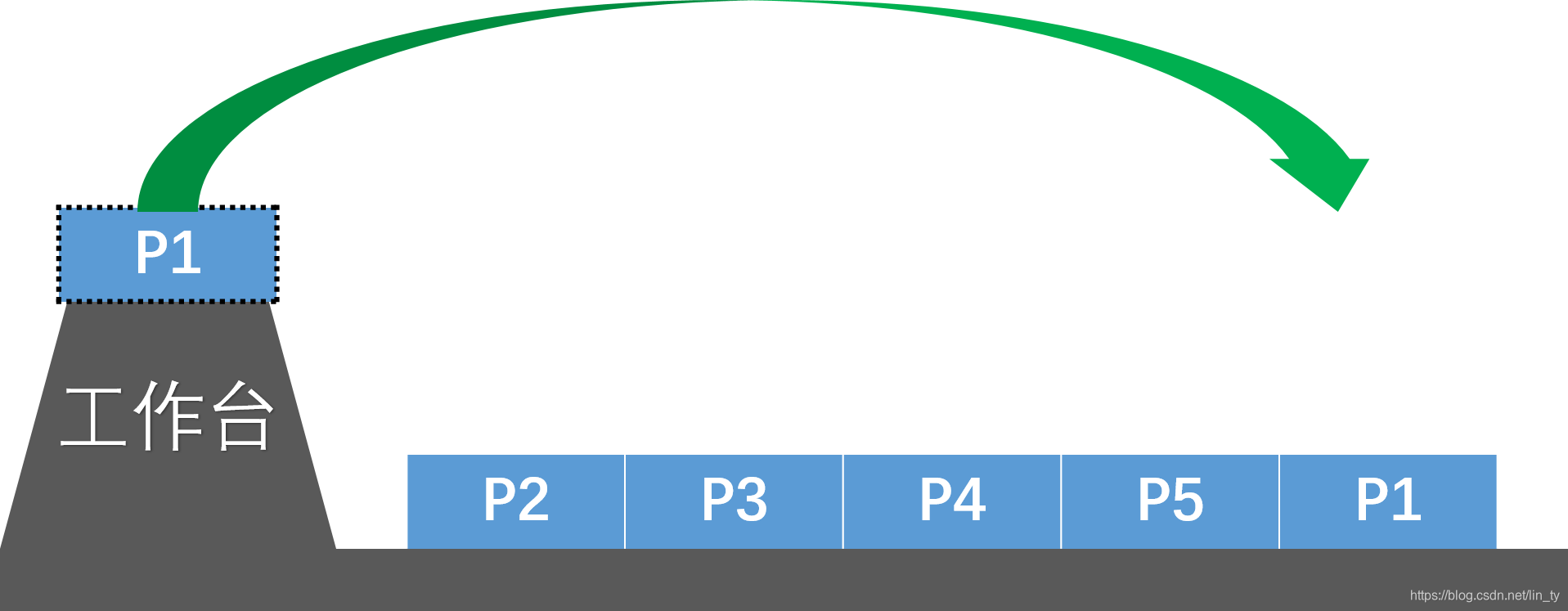
#include<iostream>
using namespace std;
struct Point{
string name;
int time;
};
const int maxn=100000+10;
Point a[maxn];
int head;
int tail;
void enqueue(Point x){
a[tail]=x;
tail=(tail+1)%maxn;
}
Point dequeue(){
Point x=a[head];
head=(head+1)%maxn;
return x;
}
int main(){
int N,T;
cin>>N>>T;
for(int i=0;i<N;i++){
cin>>a[i].name>>a[i].time;
}
head=0;
tail=N;
Point temp;
int c;
int sum=0;
while(head!=tail){
temp=dequeue();
c=min(T,temp.time);
temp.time-=c;
sum+=c;
if(temp.time>0){
enqueue(temp);
}
else
{ cout<<temp.name<<" "<<sum<<endl;
}
}
return 0;
}
Part 3 else
1.struct的基本结构
struct (名字){
变量类型 变量名;
...
}
2.typedef的用法辨析
struct Node{
int key;
Node *prev;
Node *next;
};
Node *head;
3.书籍参考《大话数据结构》
4.算法的时间复杂度
我们主要关心的是最坏的情况,因此,在多层嵌套中,要以最内层的语句作为判断的指标。 eg.两层for的嵌套影响
|