需求
排序前:{9,1,2,5,7,4,8,6,3,5} 排序后:{1,2,3,4,5,5,6,7,8,9}
一、希尔排序原理
希尔排序是插入排序算法的一种更高效的改良版本 1.选定一个增长量,按照增长量 h 作为数据分组的依据,对数据进行分组; 2.对分好组的每一组数据完成插入排序操作; 3.减小增长量,最小减为1,重复第二步操作; 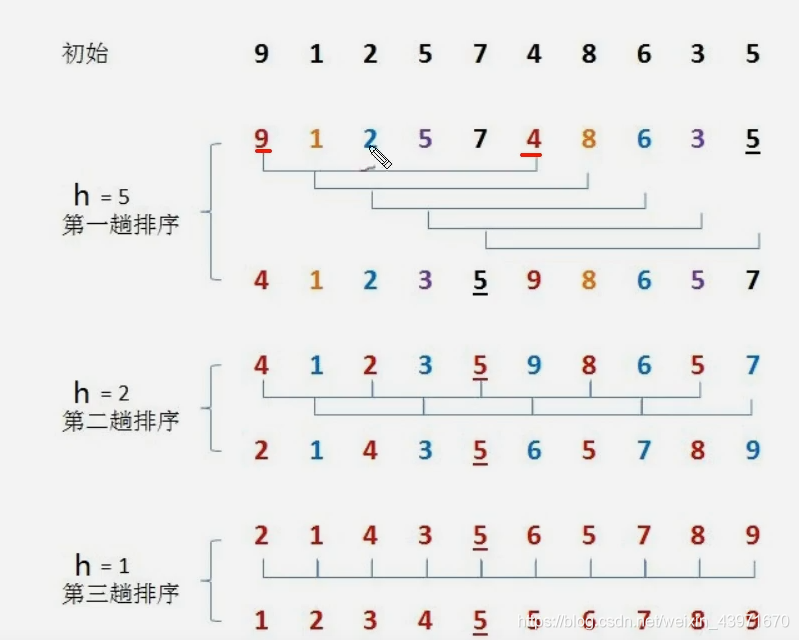
增长量 h 的确定: 增长量 h 的值没有固定的规则,我们这里采用以下规则:
int h = 1
while(h<5){
h = 2*h+1;
}
h的减小规则为:
h = h/2
二、具体步骤
1.希尔排序API设计:
类名 | Shell |
---|
构造方法 | Shell() : 创建Insertion对象 | 成员方法 | 1.public static void sort(Comparable[] a) : 对数组内的元素进行排序 2.public static boolean greater(Comparable v,Comparable w) : 判断v是否大于w 3. private static void exch(Comparable[] a,int i,int j) : 交换a数组中索引i和索引j处的值 |
2.希尔排序的代码实现:
代码如下:
Shell类:
package com.pcxtest.sort;
public class Shell {
public static void sort(Comparable[] a) {
int h = 1;
while (h < a.length/2){
h = 2*h+1;
}
while (h >= 1){
for (int i = h; i <= a.length-1; i++){
for (int j = i; j >= h; j-=h){
if (greater(a[j-h],a[j])){
exch(a,j-h,j);
}else{
break;
}
}
}
h = h/2;
}
}
public static boolean greater(Comparable v, Comparable w){
return v.compareTo(w) > 0;
}
public static void exch(Comparable[] a, int i, int j){
Comparable temp;
temp = a[i];
a[i] = a[j];
a[j] = temp;
}
}
测试类:
package com.pcxtest.test;
import com.pcxtest.sort.Shell;
import java.util.Arrays;
public class ShellTest {
public static void main(String[] args) {
Integer[] a = {9,1,2,5,7,4,8,6,3,5};
Shell.sort(a);
System.out.println(Arrays.toString(a));
}
}
本文基于此处学习
|