机器学习学习记录 1.线性回归
单变量线性回归
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
path = 'ex1data1.txt'
data = pd.read_csv(path, header=None, names=['Population', 'Profit'])
data.head()
data.describe()
其中**describe()**函数功能返回值如下:
| Population | Profit |
---|
count | 总数量 | | mean | 均值 | | std | 标准差 | | min | 最小值 | | 25% | 四分之一分位数 | | 50% | 二分之一分位数 | | 75% | 四分之三分位数 | | max | 最大值 | |
将数据可视化(画图)
data.plot(kind='scatter', x='Population', y='Profit', figsize=(12,8))
plt.show()
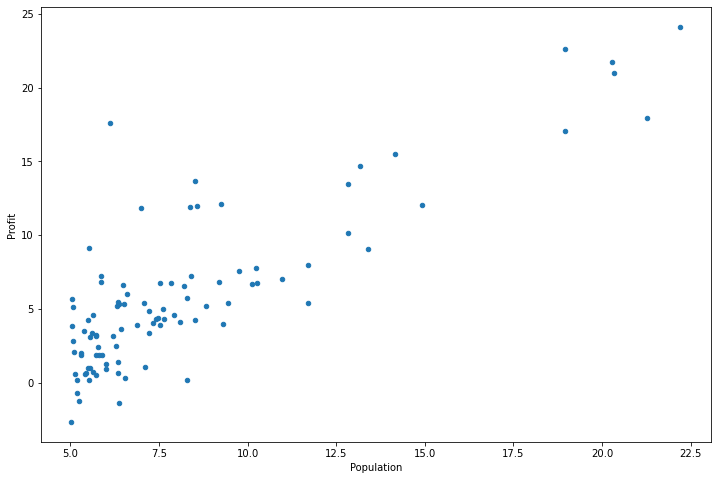
代价函数
创建一个以参数
θ
\theta
θ?为特征函数的代价函数
J
(
θ
)
=
1
2
m
∑
i
=
1
m
(
h
θ
(
x
(
i
)
)
?
y
(
i
)
)
2
J(\theta)=\frac{1}{2m}\sum_{i=1}^{m}(h_\theta(x^{(i)})-y^{(i)})^2
J(θ)=2m1?i=1∑m?(hθ?(x(i))?y(i))2 其中:
h
θ
(
x
)
=
θ
T
X
=
θ
0
x
0
+
θ
1
x
1
+
θ
2
x
2
+
.
.
.
+
θ
n
x
n
h_\theta(x)=\theta^TX=\theta_0x_0+\theta_1x_1+\theta_2x_2+...+\theta_nx_n
hθ?(x)=θTX=θ0?x0?+θ1?x1?+θ2?x2?+...+θn?xn? 创建代价函数
def computeCost(X, y, theta):
inner = np.power(((X * theta.T) - y), 2)
return np.sum(inner) / (2 * len(X))
让我们在训练集中添加一列,以便我们可以使用向量化的解决方案来计算代价和梯度。
data.insert(0, 'Ones', 1)
变量初始化
cols = data.shape[1]
X = data.iloc[:,0:cols-1]
y = data.iloc[:,cols-1:cols]
初始化theta同时将数值转换为矩阵进行计算
X = np.matrix(X.values)
y = np.matrix(y.values)
theta = np.matrix(np.array([0,0]))
此时可以计算代价函数(theta初始值为0)
computeCost(X, y, theta)
批量梯度下降
θ
j
:
=
θ
j
?
α
?
?
θ
J
(
θ
)
\theta_j:=\theta_j-\alpha\frac{\partial}{\partial\theta}J(\theta)
θj?:=θj??α?θ??J(θ)
def gradientDescent(X, y, theta, alpha, iters):
temp = np.matrix(np.zeros(theta.shape))
parameters = int(theta.ravel().shape[1])
cost = np.zeros(iters)
for i in range(iters):
error = (X * theta.T) - y
for j in range(parameters):
term = np.multiply(error, X[:,j])
temp[0,j] = theta[0,j] - ((alpha / len(X)) * np.sum(term))
theta = temp
cost[i] = computeCost(X, y, theta)
return theta, cost
执行梯度下降算法
alpha = 0.01
iters = 1000
g, cost = gradientDescent(X, y, theta, alpha, iters)
通过拟合后的参数计算计算训练模型的代价函数
computeCost(X, y, g)
将结果可视化绘制线性模型以及拟合数据(可由此参考Python画图的一般步骤)
x = np.linspace(data.Population.min(), data.Population.max(), 100)
f = g[0, 0] + (g[0, 1] * x)
fig, ax = plt.subplots(figsize=(12,8))
ax.plot(x, f, 'r', label='Prediction')
ax.scatter(data.Population, data.Profit, label='Traning Data')
ax.legend(loc=3)
ax.set_xlabel('Population')
ax.set_ylabel('Profit')
ax.set_title('Predicted Profit vs. Population Size')
plt.show()
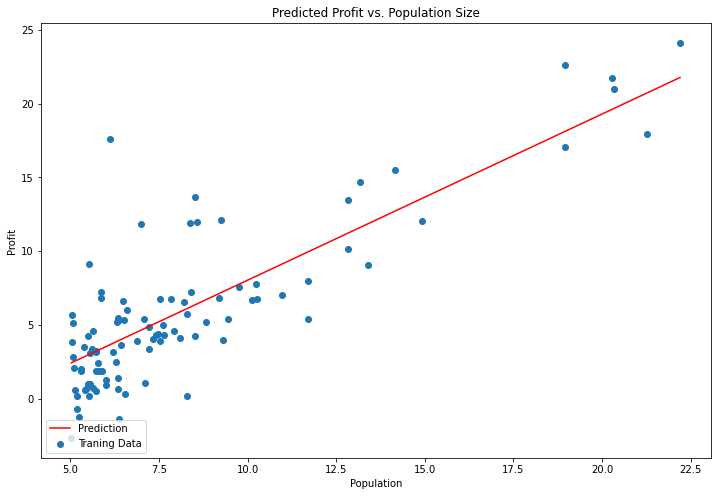
同样也可以绘制迭代次数和代价函数之间的关系
fig, ax = plt.subplots(figsize=(12,8))
ax.plot(np.arange(iters), cost, 'r')
ax.set_xlabel('Iterations')
ax.set_ylabel('Cost')
ax.set_title('Error vs. Training Epoch')
plt.show()
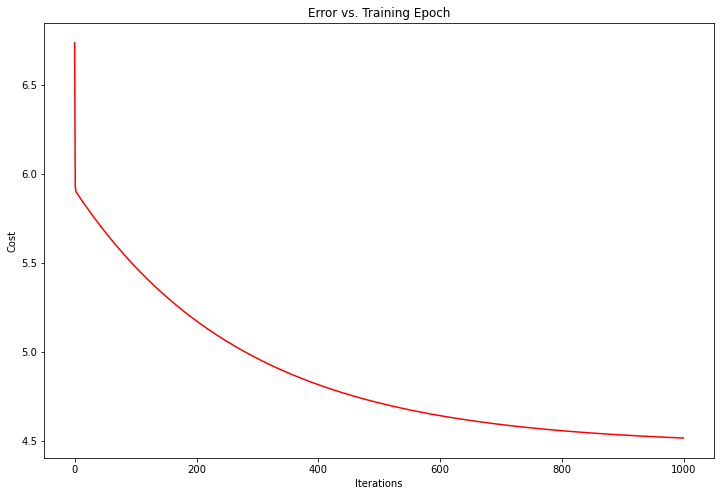
同样也可以使用scikit-learn的线性回归函数来直接应用于第一部分数据,而没有从头开始实现这些算法。
from sklearn import linear_model
model = linear_model.LinearRegression()
model.fit(X, y)
x = np.array(X[:, 1].A1)
f = model.predict(X).flatten()
fig, ax = plt.subplots(figsize=(12,8))
ax.plot(x, f, 'r', label='Prediction')
ax.scatter(data.Population, data.Profit, label='Traning Data')
ax.legend(loc=2)
ax.set_xlabel('Population')
ax.set_ylabel('Profit')
ax.set_title('Predicted Profit vs. Population Size')
plt.show()
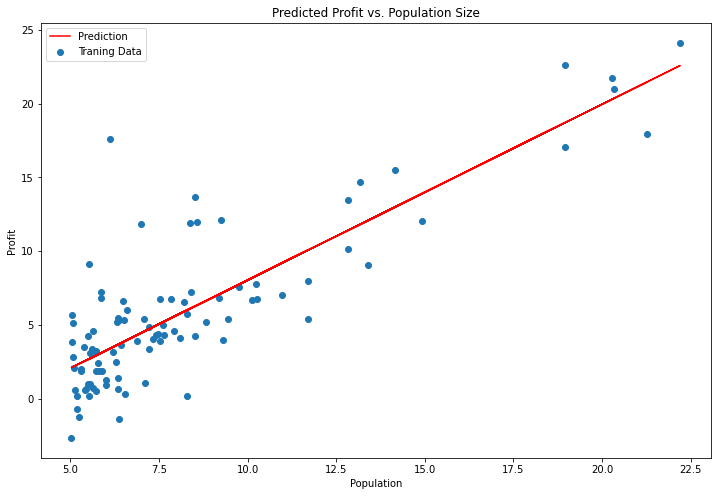
多变量线性回归
先读取数据(包括两个变量一个目标)
path = 'ex1data2.txt'
data2 = pd.read_csv(path, header=None, names=['Size', 'Bedrooms', 'Price'])
比之前多出一步:预处理特征归一化
data2 = (data2 - data2.mean()) / data2.std()
接下来重复之前的步骤
data2.insert(0, 'Ones', 1)
cols = data2.shape[1]
X2 = data2.iloc[:,0:cols-1]
y2 = data2.iloc[:,cols-1:cols]
X2 = np.matrix(X2.values)
y2 = np.matrix(y2.values)
theta2 = np.matrix(np.array([0,0,0]))
g2, cost2 = gradientDescent(X2, y2, theta2, alpha, iters)
computeCost(X2, y2, g2)
通过图像观察迭代次数与损失函数关系
fig, ax = plt.subplots(figsize=(12,8))
ax.plot(np.arange(iters), cost2, 'r')
ax.set_xlabel('Iterations')
ax.set_ylabel('Cost')
ax.set_title('Error vs. Training Epoch')
plt.show()
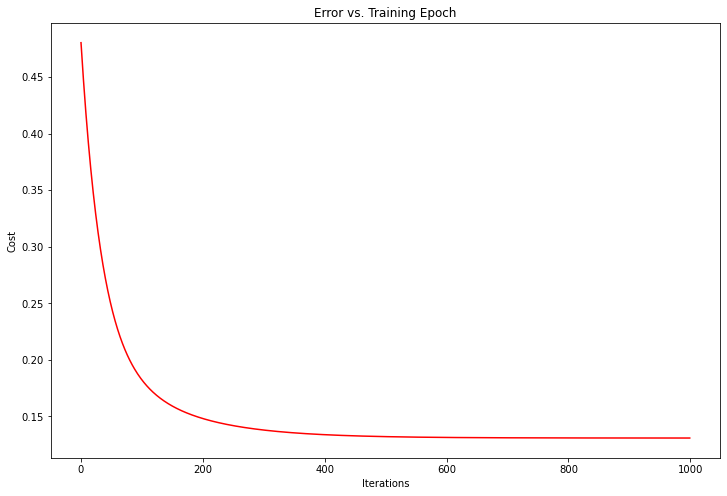
正规方程
通过求解
?
?
θ
j
J
(
θ
j
)
=
0
\frac{\partial}{\partial\theta_j}J(\theta_j)=0
?θj???J(θj?)=0 进而得到代价函数最小参数的过程称为正规方程法。
假设训练集特征矩阵为X(其中包含
x
0
=
1
x_0=1
x0?=1?)同时训练集的结果为向量
y
y
y?,则通过正规方程解得:
θ
=
(
X
T
X
)
?
1
X
T
y
\theta=(X^TX)^{-1}X^Ty
θ=(XTX)?1XTy 上标
T
T
T代表转置矩阵,上标
?
1
-1
?1代表矩阵的逆。设矩阵
A
=
X
T
X
A=X^TX
A=XTX???,则:
(
X
T
X
)
?
1
=
A
?
1
(X^{T}X)^-1=A^{-1}
(XTX)?1=A?1
def normalEqn(X, y):
theta = np.linalg.inv(X.T@X)@X.T@y
return theta
代入
X
X
X,
y
y
y,我们可以得到
final_theta2=normalEqn(X, y)
final_theta2
正规方程与梯度下降比较
梯度下降:需要选择学习率α,需要多次迭代,当特征数量n大时也能较好适用,适用于各种类型的模型
正规方程:不需要选择学习率α,一次计算得出,需要计算
(
X
T
X
)
?
1
(X^TX)^{-1}
(XTX)?1,如果特征数量n较大则运算代价大,因为矩阵逆的计算时间复杂度为
O
(
n
3
)
O(n^3)
O(n3)?,通常来说当
n
<
10000
n<10000
n<10000?时还是可以接受的,只适用于线性模型,不适合逻辑回归模型等其他模型。
备注:本次笔记是吴恩达老师的机器学习第一次课后编程题的python版本的笔记记录,欢迎大家学习交流。
|