难度:中等
给你二叉树的根节点 root 和一个整数目标和 targetSum ,找出所有 从根节点到叶子节点 路径总和等于给定目标和的路径。
叶子节点 是指没有子节点的节点。
示例 1:
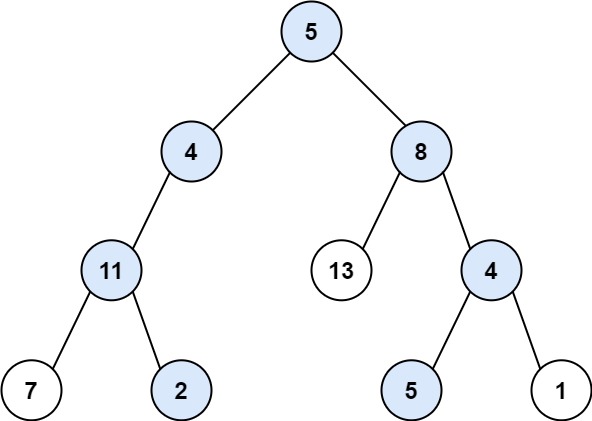
输入:root = [5,4,8,11,null,13,4,7,2,null,null,5,1], targetSum = 22
输出:[[5,4,11,2],[5,8,4,5]]
示例 2:
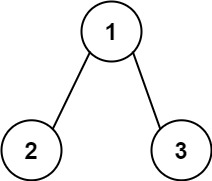
输入:root = [1,2,3], targetSum = 5
输出:[]
示例 3:
输入:root = [1,2], targetSum = 0
输出:[]
提示:
- 树中节点总数在范围
[0, 5000] 内 -1000 <= Node.val <= 1000 -1000 <= targetSum <= 1000
自解
class Solution:
def pathSum(self, root: TreeNode, targetSum: int) -> List[List[int]]:
if not root:
return []
def func(node,temp_result,value):
if not node.left and not node.right:
if value == targetSum:
result.append(temp_result)
return
if node.left:
func(node.left,temp_result + [node.left.val],value + node.left.val)
if node.right:
func(node.right,temp_result + [node.right.val],value + node.right.val)
temp_result = []
result = []
func(root,temp_result + [root.val],root.val)
return result
官解
class Solution:
def pathSum(self, root: TreeNode, targetSum: int) -> List[List[int]]:
result = []
temp_result = []
def func(root,value):
if not root:
return
temp_result.append(root.val)
value += root.val
if not root.left and not root.right and value == targetSum:
result.append(temp_result[:])
func(root.left,value)
func(root.right,value)
temp_result.pop()
func(root,0)
return result
|