二叉树的深度
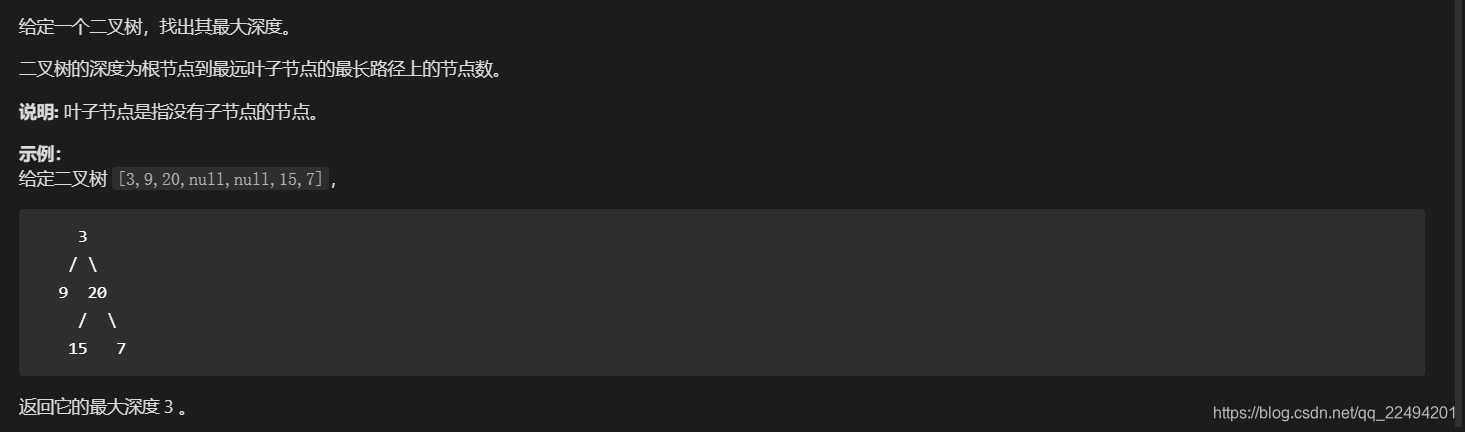 题解:
层序遍历计算深度
class Solution {
public:
int maxDepth(TreeNode* root) {
if(root == NULL){
return 0;
}
queue<TreeNode*> que;
que.push(root);
int deepth = 0;
while(!que.empty()){
int size = que.size();
deepth++;
for(int i =0;i<size;i++){
TreeNode *cur = que.front();
que.pop();
if(cur->left){
que.push(cur->left);
}
if(cur->right){
que.push(cur->right);
}
}
}
return deepth;
}
};
递归:
前序遍历:深度回溯求深度
递归三部曲: 1、确定递归函数的参数和返回值 无返回值,参数为该结点的深度以及树结点 2、确定终止条件 没有左右子树(叶节点)时即可终止 3、确定单层递归逻辑 左右子树最深叶节点的深度的最大值
class Solution {
public:
int result;
void getdepth(TreeNode * node,int depth){
result = result>depth?result:depth;
if(node->left){
depth++;
getdepth(node->left,depth);
depth--;
}
if(node->right){
depth++;
getdepth(node->right,depth);
depth--;
}
return;
}
int maxDepth(TreeNode* root) {
if(root == NULL){
return 0;
}
getdepth(root,1);
return result;
}
};
后序遍历
递归三部曲: 1、确定递归函数的参数和返回值 参数为树的根节点,返回这棵树的深度 2、确定终止条件 空结点返回0 3、确定单层递归逻辑 求左子树深度,再求右子树深度,取左右子树深度的最大值再加1
class Solution {
public:
int getdepth(TreeNode * node){
if(node==NULL){
return 0;
}
int dep1 = getdepth(node->left);
int dep2 = getdepth(node->right);
int result = 1+max(dep1,dep2);
return result;
}
int maxDepth(TreeNode* root) {
int result = getdepth(root);
return result;
}
};
n叉树的深度
层序遍历
大致逻辑与求二叉树深度一致 代码整体即为n叉树的层序遍历逻辑
class Solution {
public:
int maxDepth(Node* root) {
if(root == NULL){
return 0;
}
queue<Node*> que;
que.push(root);
int deepth = 0;
while(!que.empty()){
int size = que.size();
deepth++;
for(int i =0;i<size;i++){
Node *cur = que.front();
que.pop();
for(int j = 0;j<cur->children.size();j++){
que.push(cur->children[j]);
}
}
}
return deepth;
}
};
递归
递归三部曲: 1、确定递归函数的参数和返回值 参数为树的根节点,返回这棵树的深度 2、确定终止条件 空结点返回0 3、确定单层递归逻辑 求得孩子结点的最大深度,加1为树的深度
class Solution {
public:
int maxDepth(Node* root) {
if(root == NULL){
return 0;
}
int depth = 0;
for(int i = 0;i<root->children.size();i++){
depth = max(depth,maxDepth(root->children[i]));
}
return depth+1;
}
};
|