双链表的定义
为什么要有双向链表? 因为单链表有自身局限性(只能向后跑,不能向前跑),所以当我们需要向前跑的时候,就有了双向链表。 双向链表是个啥? 和单链表相比,多了一个直接前驱指针。 示例: 单链表(假设地址为100 200 这些。。。) 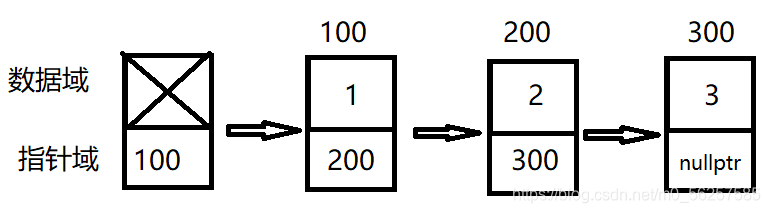
双向链表 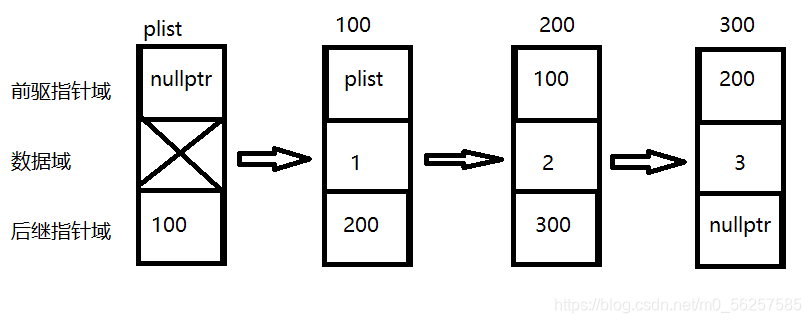
程序清单
Dlist.h
头文件中除了结构体的定义和单链表不同,其他操作基本一样。
#pragma once
typedef int ElemType;
typedef struct DNode
{
ElemType data;
struct DNode* next;
struct DNode* prior;
}DNode, * PDNode;
void Init_List(PDNode plist);
bool Insert_Head(PDNode plist, ElemType val);
bool Insert_Tail(PDNode plist, ElemType val);
bool Insert_Pos(PDNode plist, int pos, ElemType val);
bool Del_Pos(PDNode plist, int pos);
bool Del_Val(PDNode plist, ElemType val);
struct DNode* Search(PDNode plist, ElemType val);
PDNode Get_Prior(PDNode plist, ElemType val);
PDNode Get_Next(PDNode plist, ElemType val);
bool IsEmpty(PDNode plist);
int Get_Length(PDNode plist);
void Clear(PDNode plist);
void Destroy(PDNode plist);
void Destroy2(PDNode plist);
void Show(PDNode plist);
Dlist.cpp
#include<stdio.h>
#include <assert.h>
#include <stdlib.h>
#include<stdbool.h>
#include "dlist.h"
void Init_List(PDNode plist)
{
assert(plist != nullptr);
plist->next = nullptr;
plist->prior = nullptr;
}
bool Insert_Head(PDNode plist, ElemType val)
{
assert(plist != nullptr);
DNode* pnewnode = (DNode*)malloc(sizeof(DNode) * 1);
assert(pnewnode != nullptr);
pnewnode->data = val;
pnewnode->next = plist->next;
pnewnode->prior = plist;
if (plist->next != nullptr)
{
plist->next->prior = pnewnode;
}
plist->next = pnewnode;
return true;
}
bool Insert_Tail(PDNode plist, ElemType val)
{
assert(plist != nullptr);
DNode* pnewnode = (DNode*)malloc(sizeof(DNode) * 1);
assert(pnewnode != nullptr);
pnewnode->data = val;
DNode* p = plist;
for (p; p->next != nullptr; p = p->next);
pnewnode->next = p->next;
pnewnode->prior = p;
p->next = pnewnode;
return true;
}
bool Insert_Pos(PDNode plist, int pos, ElemType val)
{
assert(plist != nullptr && pos >= 0 && pos <= Get_Length(plist));
if (pos < 0 || pos > Get_Length(plist))
return false;
DNode* pnewnode = (DNode*)malloc(sizeof(DNode) * 1);
assert(pnewnode != nullptr);
pnewnode->data = val;
DNode* p = plist;
for (int i = 0; i < pos; i++)
{
p = p->next;
}
pnewnode->next = p->next;
pnewnode->prior = p;
if (p->next != nullptr)
{
p->next->prior = pnewnode;
}
p->next = pnewnode;
return true;
}
bool Del_Pos(PDNode plist, int pos)
{
assert(plist != nullptr && pos >= 0 && pos < Get_Length(plist));
if (pos < 0 || pos >= Get_Length(plist))
return false;
DNode* p = plist;
for (int i = 0; i <= pos; i++)
{
p = p->next;
}
if (p->next != nullptr)
{
p->next->prior = p->prior;
}
p->prior->next = p->next;
free(p);
p = nullptr;
return true;
}
bool Del_Val(PDNode plist, ElemType val)
{
assert(plist != nullptr);
DNode* p = Search(plist, val);
if (p == nullptr)
return false;
if (p->next != nullptr)
{
p->next->prior = p->prior;
}
p->prior->next = p->next;
free(p);
p = nullptr;
return true;
}
bool Del_Head(PDNode plist)
{
assert(plist != nullptr);
if (plist == nullptr || plist->next == nullptr)
return false;
DNode* p = plist->next;
if (p->next != nullptr)
{
p->next->prior = plist;
}
plist->next = p->next;
free(p);
p = nullptr;
return true;
}
bool Del_Tail(PDNode plist)
{
assert(plist != nullptr);
DNode* p = plist;
for (p; p->next != nullptr; p = p->next);
p->prior->next = nullptr;
free(p);
p = nullptr;
return true;
}
struct DNode* Search(PDNode plist, ElemType val)
{
assert(plist != nullptr);
DNode* p = plist->next;
for (p; p != nullptr; p = p->next)
{
if (p->data == val)
{
return p;
}
}
return nullptr;
}
PDNode Get_Prior(PDNode plist, ElemType val)
{
assert(plist != nullptr);
DNode* p = Search(plist, val);
return p == nullptr ? nullptr : p->prior;
}
PDNode Get_Next(PDNode plist, ElemType val)
{
assert(plist != nullptr);
DNode* p = Search(plist, val);
return p == nullptr ? nullptr : p->next;
}
bool IsEmpty(PDNode plist)
{
assert(plist != nullptr);
return plist->next == nullptr;
}
int Get_Length(PDNode plist)
{
int count = 0;
for (DNode* p = plist->next; p != nullptr; p = p->next)
{
count++;
}
return count;
}
void Clear(PDNode plist)
{
Destroy(plist);
}
void Destroy(PDNode plist)
{
assert(plist != nullptr);
while (plist->next != nullptr)
{
DNode* p = plist->next;
plist->next = p->next;
free(p);
}
}
void Destroy2(PDNode plist)
{
assert(plist != nullptr);
DNode* p = plist->next;
DNode* q = nullptr;
while (p != nullptr)
{
q = p->next;
free(p);
p = q;
}
plist->next = nullptr;
}
void Show(PDNode plist)
{
assert(plist != nullptr);
for (DNode* p = plist->next; p != nullptr; p = p->next)
{
printf("%d ", p->data);
}
printf("\n");
}
main.cpp
主函数的测试和单链表用相同的方法测试一下
#include<stdio.h>
#include"Dlist.h"
int main(void)
{
struct DNode list;
Init_List(&list);
for (int i = 0; i < 10; ++i)
{
Insert_Pos(&list, i, i + 1);
}
Show(&list);
Insert_Head(&list, 100);
Insert_Tail(&list, 100);
Show(&list);
printf("Length = %d\n", Get_Length(&list));
Del_Pos(&list, 3);
Del_Val(&list, 6);
Show(&list);
printf("Length = %d\n", Get_Length(&list));
Clear(&list);
Destroy(&list);
printf("Length = %d\n", Get_Length(&list));
return 0;
}
运行结果
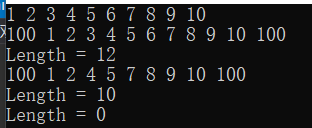
总结
容易出错的点
- 正常来说单链表需要处理2根线 而双向链表需要处理4根线
- 但是头插函数比较特殊,需要防止出现空链表导致少处理一根线
- 按位置删除函数也比较特殊,有可能删除的是最后一个节点
- 头删函数比较特殊 , 有可能只有一个有效节点需要删除
两个常用循环 如果需要前驱 ,一般用于插入和删除操作等等
for(DNode* p = plist; p->next != nullptr; p = p->next);
如果不需要前驱 , 一般用于查找和打印
for(DNode* p = plist->next; p! = nullptr; p = p->next);
|