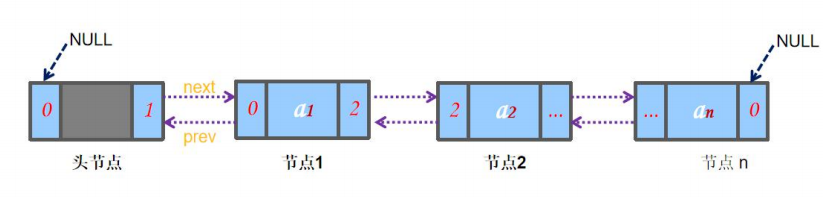
定义一个双向链表
typedef struct LinkNode {
int data;
LinkNode *next;
LinkNode *last;
}LinkNode,
LinkList;
初始化空的双向链表
bool List_Init(LinkList *&list) {
list = new LinkNode;
if (!list) return false;
list->next = NULL;
list->last = NULL;
return true;
}
前插法插入节点
bool List_Add_front(LinkList *&list, LinkNode *node) {
LinkNode *p = list;
if (!list || !node)return false;
if (list->next == NULL) {
node->next = NULL;
node->last = list;
list->next = node;
} else {
list->next->last = node;
node->next = list->next;
node->last = list;
list->next = node;
}
return true;
}
尾插法插入节点
bool List_Add_Back(LinkList *&list, LinkNode *node) {
LinkNode *last = NULL;
if (!list || !node) return false;
last = list;
while (last->next) {
last = last->next;
}
node->next = NULL;
node->last = last;
last->next = node;
return true;
}
指定位置插入节点
bool List_Insert(LinkList *&list, int i, int &e) {
if (!list || !list->next) return false;
if (i < 1) return false;
int j = 0;
LinkList *p = NULL, *s = NULL;
p = list;
while (p && j < i) {
p = p->next;
j++;
}
if (!p || j != i) {
cout << "要插入的节点不存在" << endl;
return false;
}
s = new LinkNode;
s->data = e;
p->last->next = s;
s->last = p->last;
s->next = p;
p->last = s;
return true;
}
获取指定节点的数据
bool List_GetElem(LinkList *&list, int i, int &e) {
LinkList *p = NULL;
int index;
if (!list || !list->next) return false;
p = list->next;
index = 1;
while (p && index < i) {
p = p->next;
index++;
}
if (!p || index > i) return false;
e = p->data;
return true;
}
删除指定位置的节点
bool List_Delete(LinkList *&list, int i) {
LinkNode *p = NULL;
int j = 0;
if (!list || !list->next) return false;
if (i < 1) return false;
p = list;
while (p && j < i) {
p = p->next;
j++;
}
if (!p) {
return false;
}
if (!p->next) {
p->last->next = NULL;
delete p;
return true;
}
p->last->next = p->next;
p->next->last = p->last;
delete p;
return true;
}
销毁双向链表
void List_Destroy(LinkList *&list) {
LinkList *p = list;
cout << "销毁链表!" << endl;
while (p) {
list = list->next;
delete p;
p = list;
}
}
输出双向链表
void List_Print(LinkList *&list) {
LinkNode *p = NULL;
if (!list) return;
p = list;
while (p->next) {
cout << p->next->data << "\t";
p = p->next;
}
cout << endl;
}
main函数
int main(void) {
LinkList *list = NULL;
LinkNode *node = NULL;
int n = 0;
List_Init(list);
cout << "请输入插入的数量: ";
cin >> n;
for (int i = 0; i < n; i++) {
node = new LinkNode;
node->data = i+1;
List_Add_front(list, node);
}
List_Print(list);
int n;
cout << "请输入要删除节点的位置: ";
cin >> n;
if (List_Delete(list, n)) {
cout << "删除第" << n << "个节点成功!" << endl;
} else {
cout << "删除第" << n << "个节点失败!" << endl;
}
List_Destroy(list);
List_Print(list);
system("pause");
return 0;
}
|