1.链表回文结构 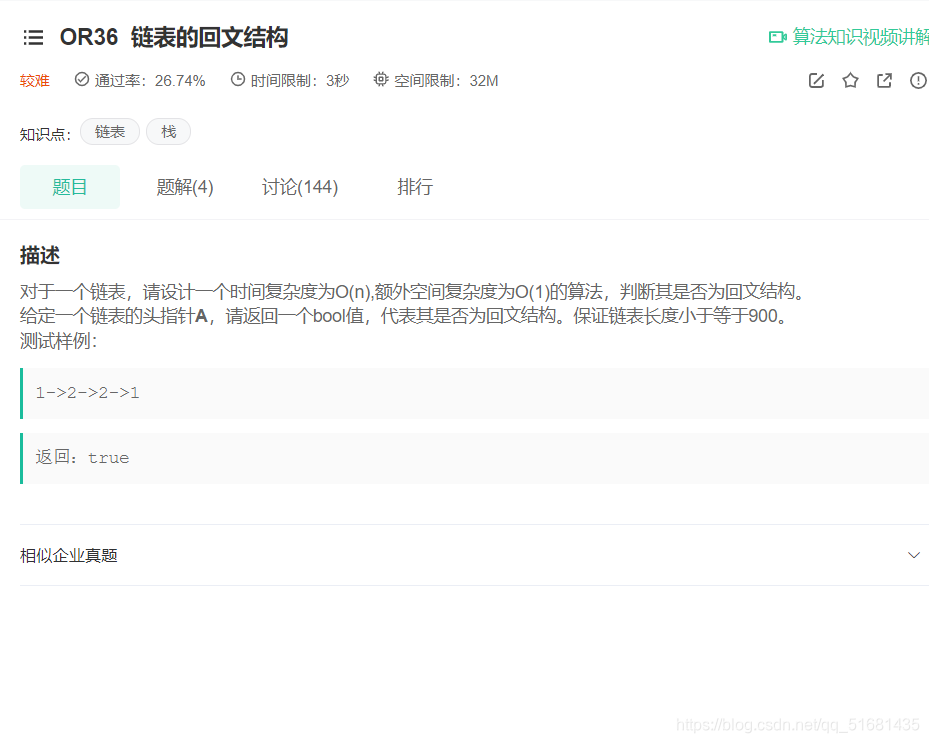
class PalindromeList {
public:
bool chkPalindrome(ListNode* A) {
if(A==nullptr&&A->next==nullptr)
return true;
ListNode* cur=A;
while(cur)
{
ListNode* next=cur->next;
s.push(cur->val);
q.push(cur->val);
cur=next;
}
while(!s.empty()&&!q.empty())
{
if(s.top()!=q.front())
{
return false;
}
else
{
return true;
}
s.pop();
q.pop();
}
return true;
}
stack<int> s;
queue<int> q;
};
2.求两个链表第一个公共节点 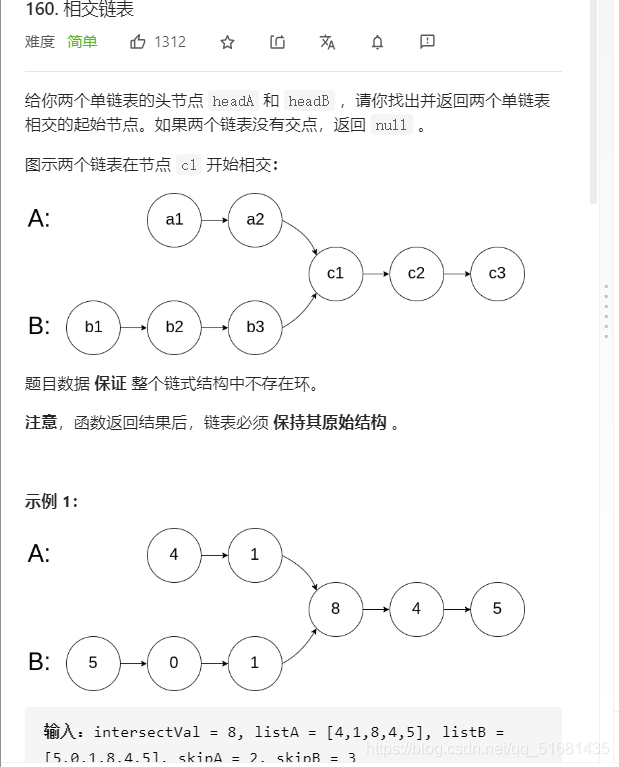
class Solution {
public:
ListNode *getIntersectionNode(ListNode *headA, ListNode *headB) {
ListNode *curA,*curB;
curA=headA;
curB=headB;
int lenA=0;
int lenB=0;
while(curA)
{
++lenA;
curA=curA->next;
}
while(curB)
{
++lenB;
curB=curB->next;
}
curA=headA;
curB=headB;
if(lenA>lenB)
{
int gapA=lenA-lenB;
while(gapA--)
{
curA=curA->next;
}
}
if(lenA<lenB)
{
int gapB=lenB-lenA;
while(gapB--)
{
curB=curB->next;
}
}
while(curA&&curB)
{
if(curA==curB)
{
return curA;
}
curA=curA->next;
curB=curB->next;
}
return nullptr;
}
};
3.判断链表是否有环 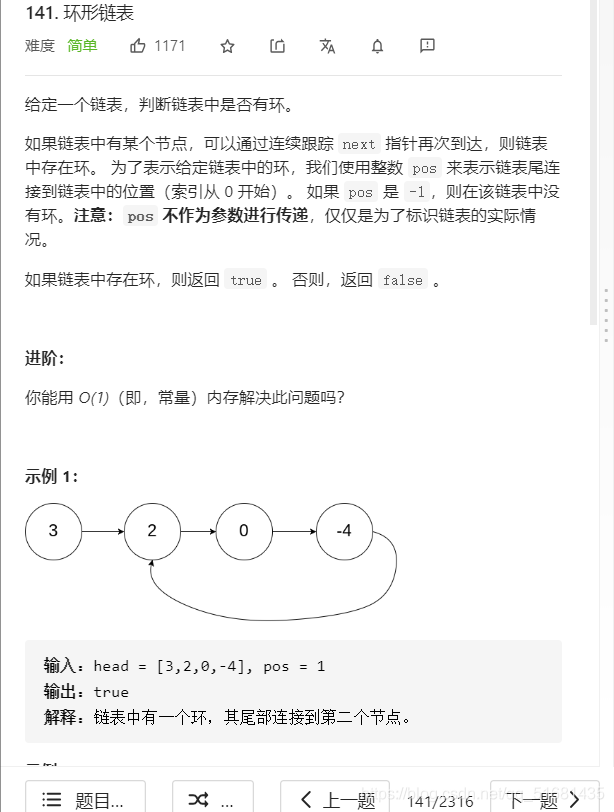
class Solution {
public:
bool hasCycle(ListNode *head) {
ListNode *fast,*slow;
fast=slow=head;
while(fast&&fast->next)
{
fast=fast->next->next;
slow=slow->next;
if(fast==slow)
{
return true;
}
}
return false;
}
};
4.环的入口位置 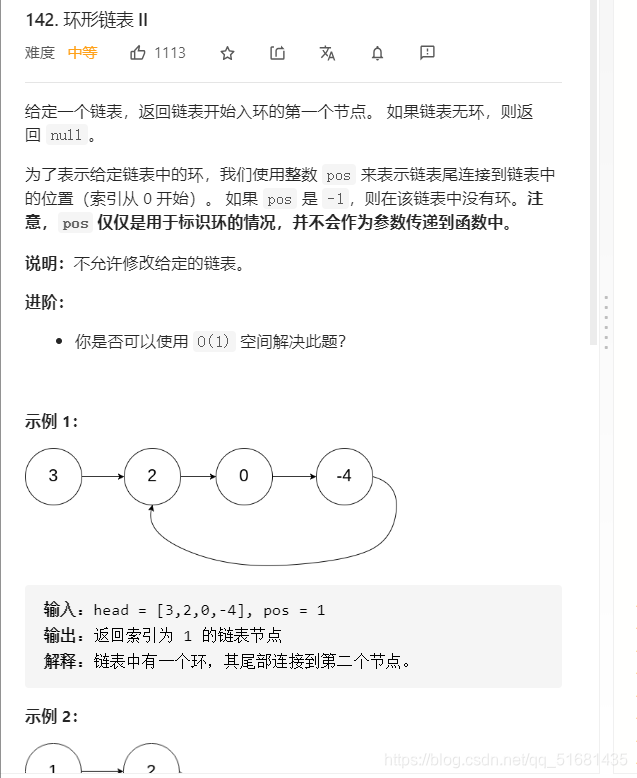
class Solution {
public:
ListNode* hascyle(ListNode* head)
{
ListNode* fast,*slow;
fast=slow=head;
while(fast&&fast->next)
{
fast=fast->next->next;
slow=slow->next;
if(fast==slow)
{
return fast;
}
}
return nullptr;
}
ListNode *detectCycle(ListNode *head) {
ListNode* cur=hascyle(head);
if(cur)
{
while(cur)
{
if(cur==head)
{
return cur;
}
cur=cur->next;
head=head->next;
}
}
return nullptr;
}
};
5.拷贝一个链表 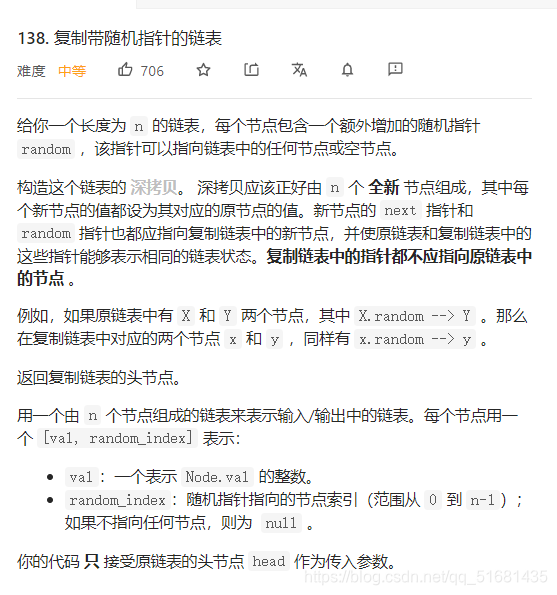 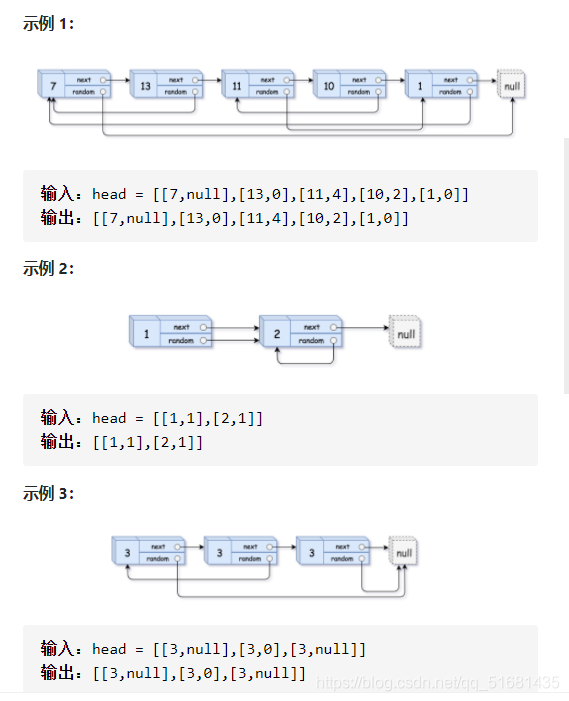
class Solution {
public:
Node* copyRandomList(Node* head) {
if(head==nullptr)
{
return head;
}
Node* cur=head;
while(cur)
{
Node* newnode=new Node(cur->val);
Node* next=cur->next;
newnode->next=next;
cur->next=newnode;
cur=newnode->next;
}
cur=head;
while(cur)
{
Node* copy=cur->next;
if(cur->random)
{
copy->random=cur->random->next;
}
else
{
copy->random=nullptr;
}
cur=copy->next;
}
Node* newhead=nullptr;
cur=head;
while(cur)
{
Node* copy=cur->next;
Node* next=copy->next;
cur->next=next;
if(newhead==nullptr)
{
newhead=copy;
}
if(next)
{
copy->next=next->next;
}
cur=next;
}
return newhead;
}
};
|