问题
给你单链表的头节点 head ,请你反转链表,并返回反转后的链表。 如 1->3->5->7->9,翻转之后:9->7->5->3->1
思路
因为不是数组结构,不能使用双指针,从两头向中遍历翻转(error); 所以我们只能通过改变next域的方向来翻转。 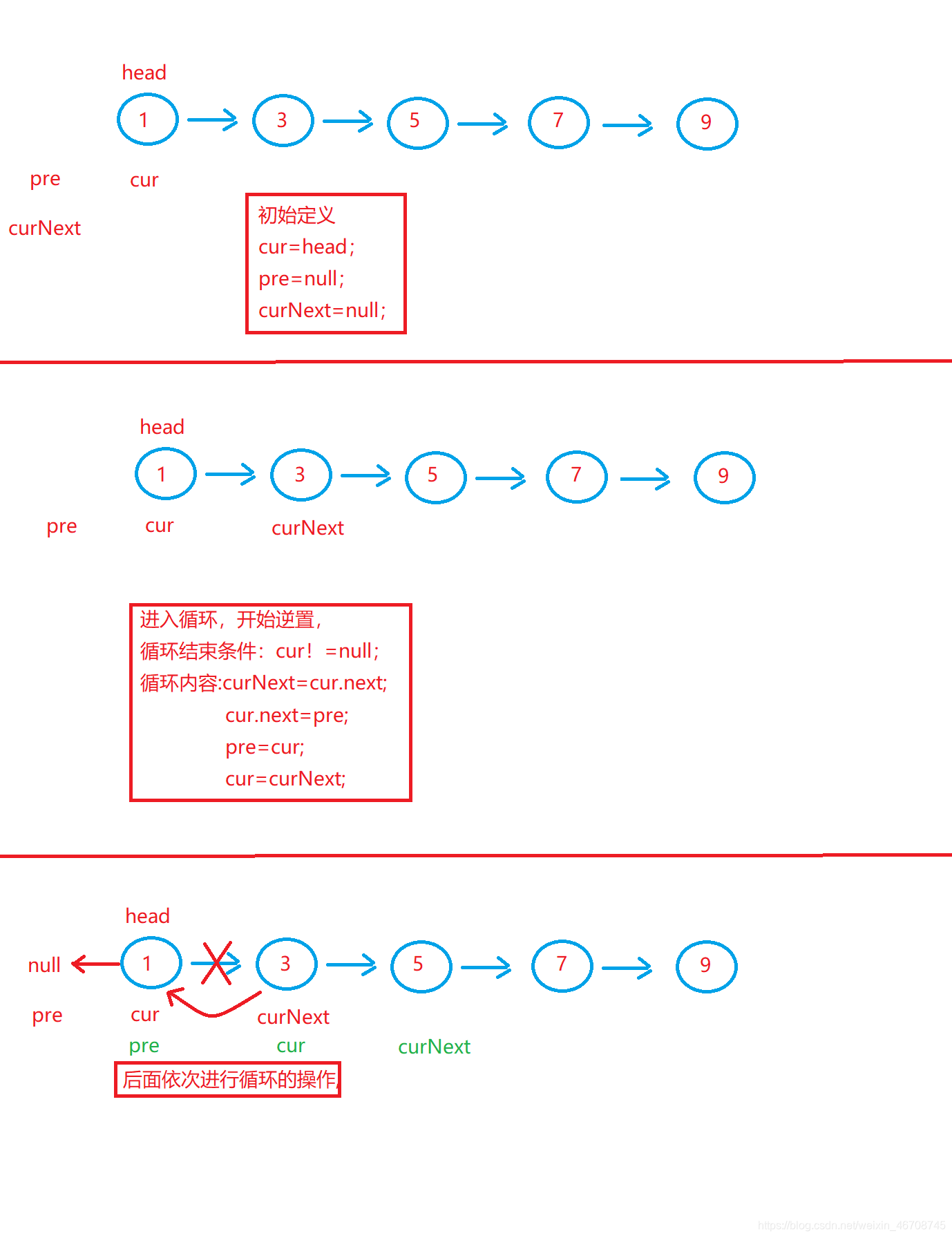
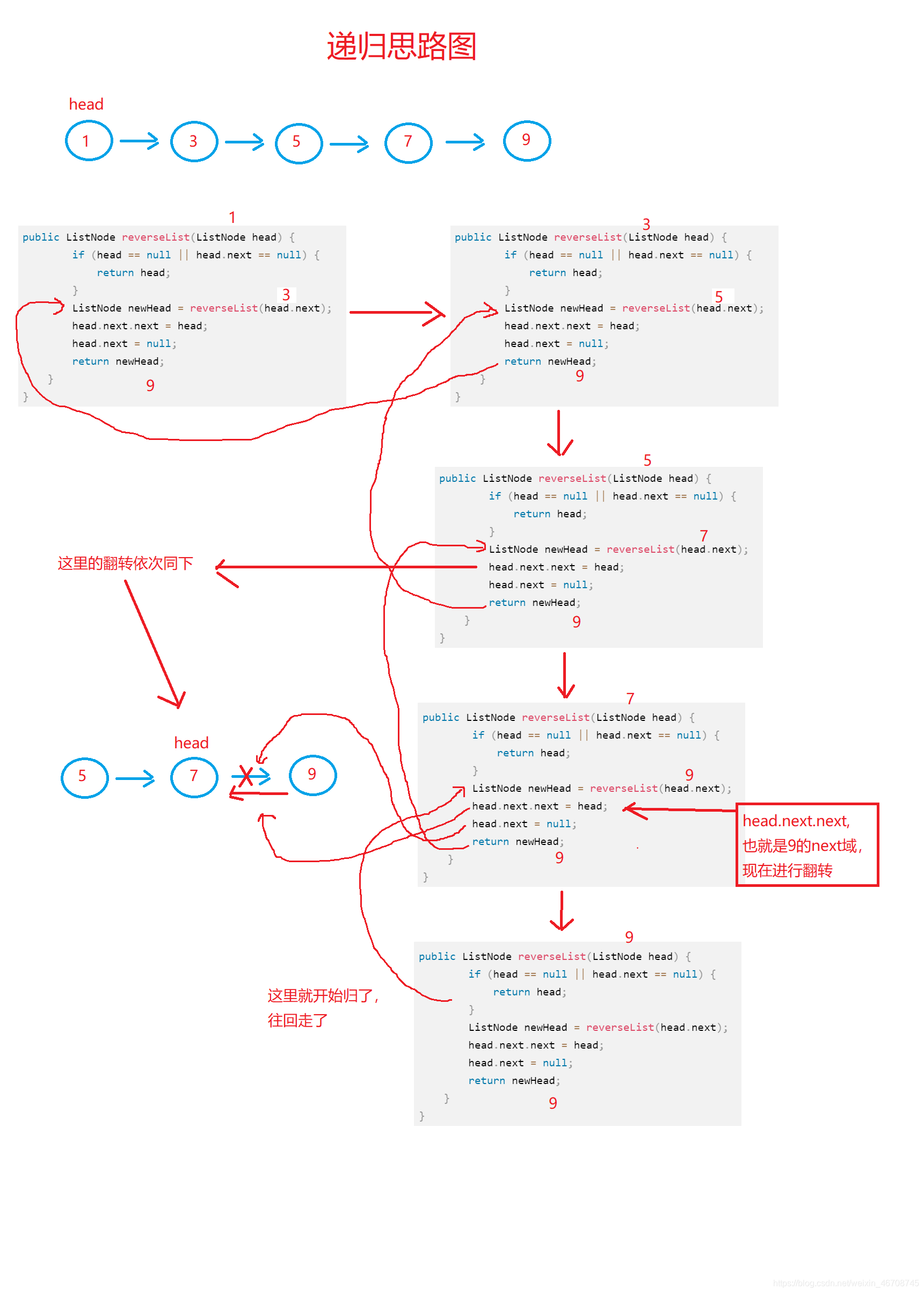
代码
解释都在注释中。
迭代
public ListNode reverseList(ListNode head) {
if(head==null){
return null;
}
ListNode cur=head;
ListNode pre=null;
ListNode curNext=null;
while(cur!=null){
curNext=cur.next;
cur.next=pre;
pre=cur;
cur=curNext;
}
return pre;
}
递归
递归等展开到最后,从后向前的翻转。可以多去理解上面的图片。
public ListNode reverseList(ListNode head) {
if (head == null || head.next == null) {
return head;
}
ListNode newHead = reverseList(head.next);
head.next.next = head;
head.next = null;
return newHead;
}
}
使用哪种方法还是得看长度,如果链表很长,使用递归容易出现StackOverflowError(栈溢出错误)。
|