记录手撸红黑树
今天得知学校提前返校,想到我整整一个暑假都在玩手机,有点内疚 ……一拍大腿,不行! 于是试着手撸红黑树
上网看了很多资料、博客,但还是一脸懵逼,幸好也做出来了一点。
学习数据结构的网站!!吹爆!!👇
https://www.cs.usfca.edu/~galles/visualization/Algorithms.html
手撸插入流程图
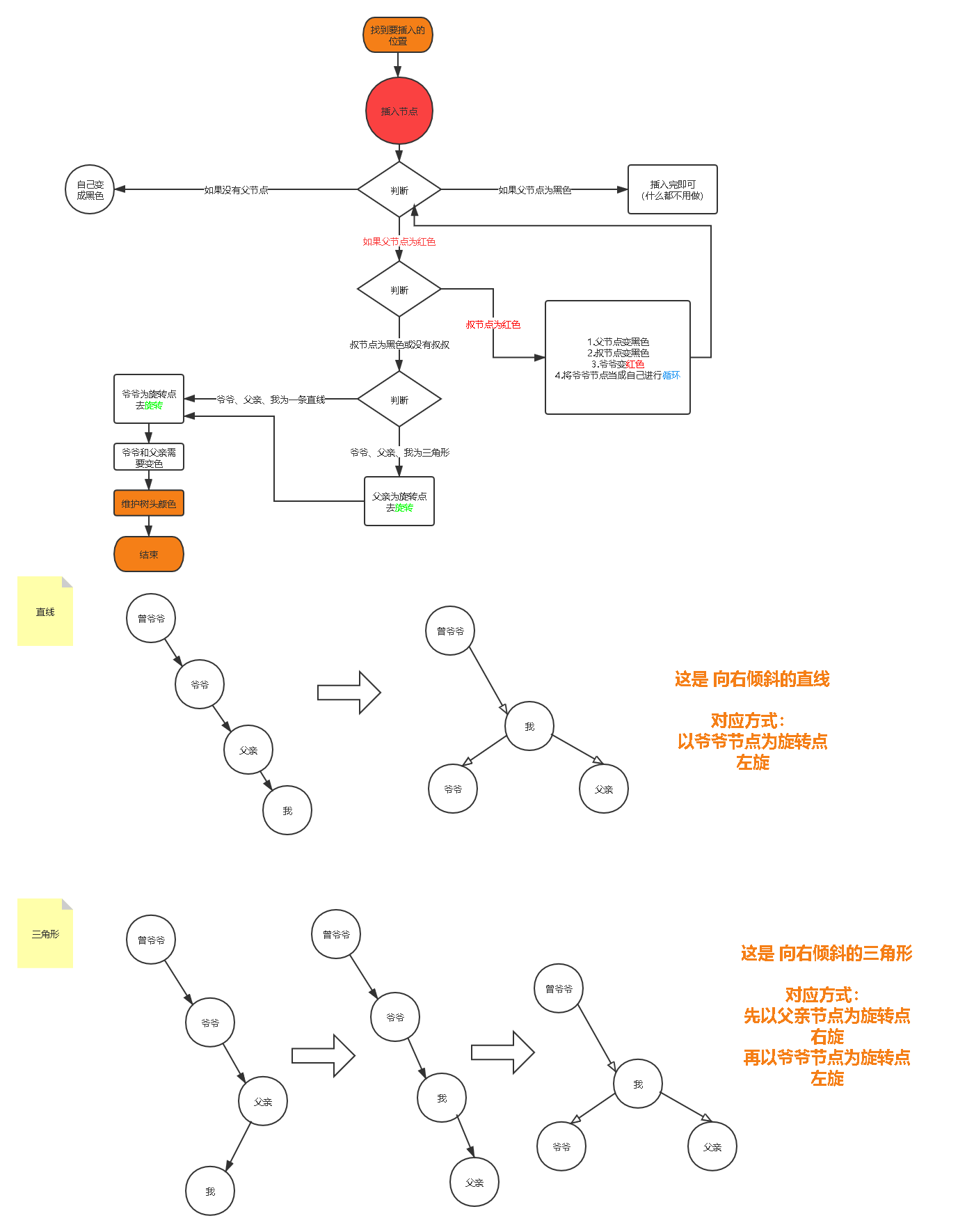
节点旋转
引用别人的动图
右旋
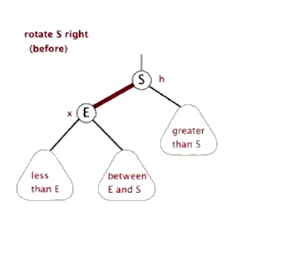
左旋
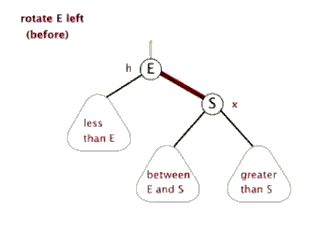
代码
红黑树代码
import java.util.LinkedList;
import java.util.Queue;
public class BlackRedTree {
static class Node{
int data;
Node father=null;
Node left=null;
Node right=null;
Node next=null;
boolean black=true;
Node(){}
Node(int data){
this.data=data;
}
}
private Node TreeHeadNode= new Node();
private void insert(Node NewNode){
NewNode.black=false;
if(this.TreeHeadNode.next==null){
this.TreeHeadNode.next=NewNode;
NewNode.father=TreeHeadNode;
return;
}
Node pMove=this.TreeHeadNode.next;
while(pMove!=null){
if(NewNode.data<pMove.data){
if(pMove.left==null){
pMove.left=NewNode;
NewNode.father=pMove;
return;
}
pMove=pMove.left;
}
else{
if(pMove.right==null){
pMove.right=NewNode;
NewNode.father=pMove;
return;
}
pMove=pMove.right;
}
}
}
public void put(int data) {
Node NewNode=new Node(data);
insert(NewNode);
while(true){
if(this.TreeHeadNode.next==NewNode)
{
NewNode.black=true;
return;
}
else{
Node Father=NewNode.father;
if(Father.black)
return;
else{
Node GrandFather=Father.father;
Node Uncle=GetUncle(GrandFather,Father);
if(Uncle==null||Uncle.black)
{
if(Shape(GrandFather,Father,NewNode))
{
if (RotationDirection(Father,NewNode))
RightRotation(GrandFather);
else {
LeftRotation(GrandFather);
}
GrandFather.black=!GrandFather.black;
Father.black=!Father.black;
UpdateTreeHeadColor();
return;
}
else
{
if (RotationDirection(GrandFather,Father))
{
LeftRotation(Father);
RightRotation(GrandFather);
}
else
{
RightRotation(Father);
LeftRotation(GrandFather);
}
GrandFather.black=!GrandFather.black;
Father.black=!Father.black;
UpdateTreeHeadColor();
}
}
else {
Father.black=true;
Uncle.black=true;
GrandFather.black=false;
NewNode=GrandFather;
}
}
}
}
}
private void UpdateTreeHeadColor(){
this.TreeHeadNode.next.black=true;
}
private boolean RotationDirection(Node Father,Node me){
return Father.left==me?true:false;
}
private void LeftRotation(Node axis) {
Node Son=axis.right;
if(Son.left!=null) {
Node GrandSon=Son.left;
axis.right=GrandSon;
GrandSon.father=axis;
}
else
axis.right=null;
ConnectAxisFatherPoint(axis,Son);
Son.father=axis.father;
Son.left=axis;
axis.father=Son;
}
private void RightRotation(Node axis){
Node Son=axis.left;
if(Son.right!=null){
Node GrandSon=Son.right;
axis.left=GrandSon;
GrandSon.father=axis;
}
else
axis.left=null;
ConnectAxisFatherPoint(axis,Son);
Son.father=axis.father;
Son.right=axis;
axis.father=Son;
}
private void ConnectAxisFatherPoint(Node axis,Node Son){
Node Father=axis.father;
if(Father==TreeHeadNode)
TreeHeadNode.next=Son;
else if(Father.left==axis)
Father.left=Son;
else
Father.right=Son;
}
private boolean Shape(Node GrandFather,Node Father,Node me){
boolean line1=GrandFather.left==Father;
boolean line2=Father.left==me;
return line1==line2;
}
private Node GetUncle(Node GrandFather,Node Father){
return GrandFather.left==Father?GrandFather.right:GrandFather.left;
}
private void BFS(){
Queue<Node> queue=new LinkedList<>();
queue.add(this.TreeHeadNode.next);
while(!queue.isEmpty()){
Node TempNode=queue.poll();
System.out.println(TempNode.data);
if(TempNode.left!=null)
queue.add(TempNode.left);
if(TempNode.right!=null)
queue.add(TempNode.right);
}
}
}
测试main方法
public static void main(String[] args) {
BlackRedTree tree=new BlackRedTree();
for (int i = 1; i <=8; i++) {
tree.put(i);
}
tree.BFS();
}
测试点:1,2,3,4,5,6,7,8
这是红黑树一系列旋转的示意图 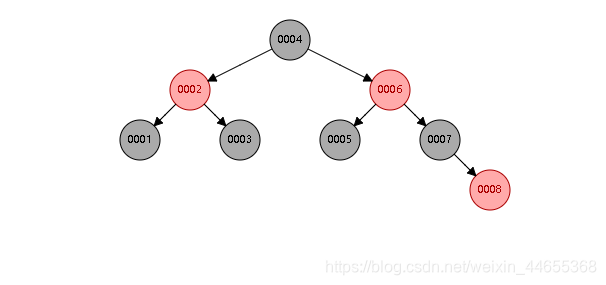 对应的BFS为:4,2,6,1,3,5,7,8
测试点:3,2,7,5,6
这是红黑树一系列旋转的示意图 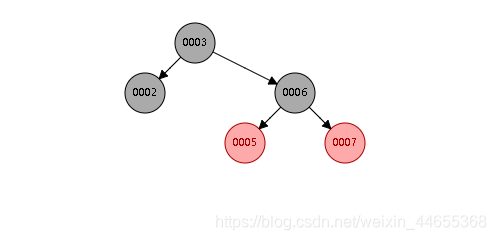
对应的BFS为:3,2,6,5,7
|