相同的树
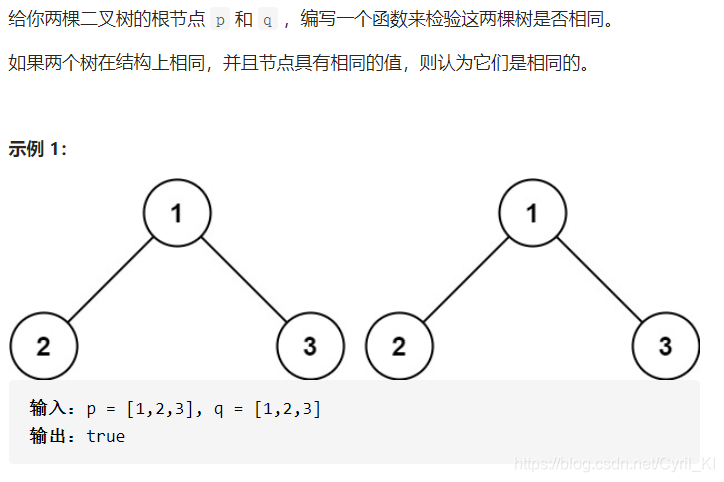 分析: ??递归判断。 代码:
class Solution {
public:
bool isSameTree(TreeNode* p, TreeNode* q) {
if(p == NULL && q == NULL) {
return true;
}else if(p == NULL || q == NULL) {
return false;
}else if(p->val != q->val) {
return false;
}else {
return isSameTree(p->left, q->left) && isSameTree(p->right, q->right);
}
}
};
反转字符串中的元音字母
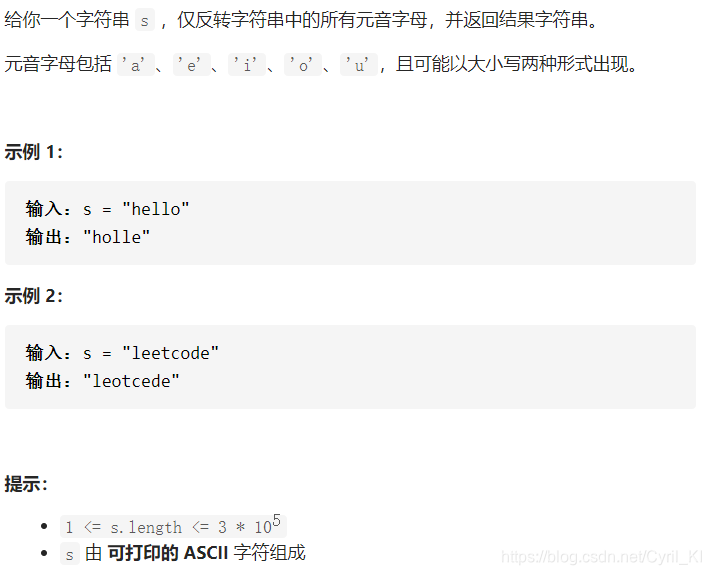 分析: ??双指针法:一个指针指向头,另一个指向尾,当两个指针指向的字符都为元音字母时交换两个字符,然后继续寻找。 代码:
class Solution {
public:
string reverseVowels(string s) {
int n = s.size();
int i = 0, j = n - 1;
string temp = "aeiouAEIOU";
while(i <= j) {
while(i < n && temp.find(s[i]) == -1) {
i++;
}
while(j >= 0 && temp.find(s[j]) == -1) {
j--;
}
if(i > j) {
break;
}
char t = s[i];
s[i] = s[j];
s[j] = t;
i++, j--;
}
return s;
}
};
有效的数独
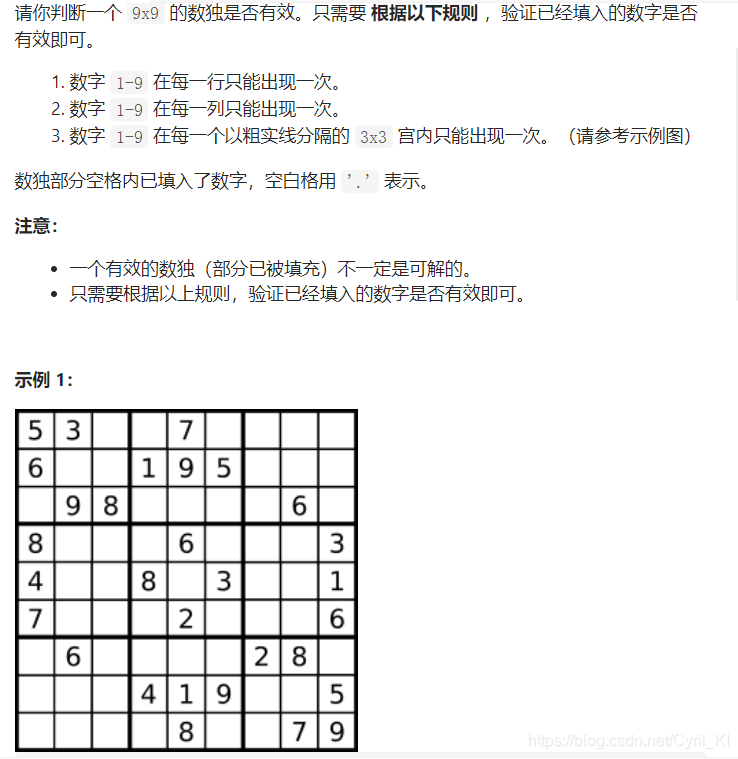 分析: ??根据题意判断即可。 代码:
class Solution {
public:
bool isValidSudoku(vector<vector<char>>& board) {
for(int i = 0, p = 0; i < 9, p < 9; i++, p++) {
vector<int> temp1(10, 0), temp2(10, 0);
for(int j = 0, q = 0; j < 9, q < 9; j++, q++) {
if(board[i][j] != '.') {
temp1[board[i][j] - '0']++;
if(temp1[board[i][j] - '0'] > 1) {
return false;
}
}
if(board[q][p] != '.') {
temp2[board[q][p] - '0']++;
if(temp2[board[q][p] - '0'] > 1) {
return false;
}
}
}
}
int dirs[9][2] = {{0, 0}, {0, 3}, {0, 6}, {3, 0}, {3, 3}, {3, 6}, {6, 0}, {6, 3}, {6, 6}};
for(int k = 0; k < 9; k++) {
vector<int> temp(10, 0);
int p = dirs[k][0], q = dirs[k][1];
for(int i = p; i < p + 3; i++) {
for(int j = q; j < q + 3; j++) {
if(board[i][j] != '.') {
temp[board[i][j] - '0']++;
if(temp[board[i][j] - '0'] > 1) {
return false;
}
}
}
}
}
return true;
}
};
|