题目
题链:剑指 Offer 54. 二叉搜索树的第k大节点 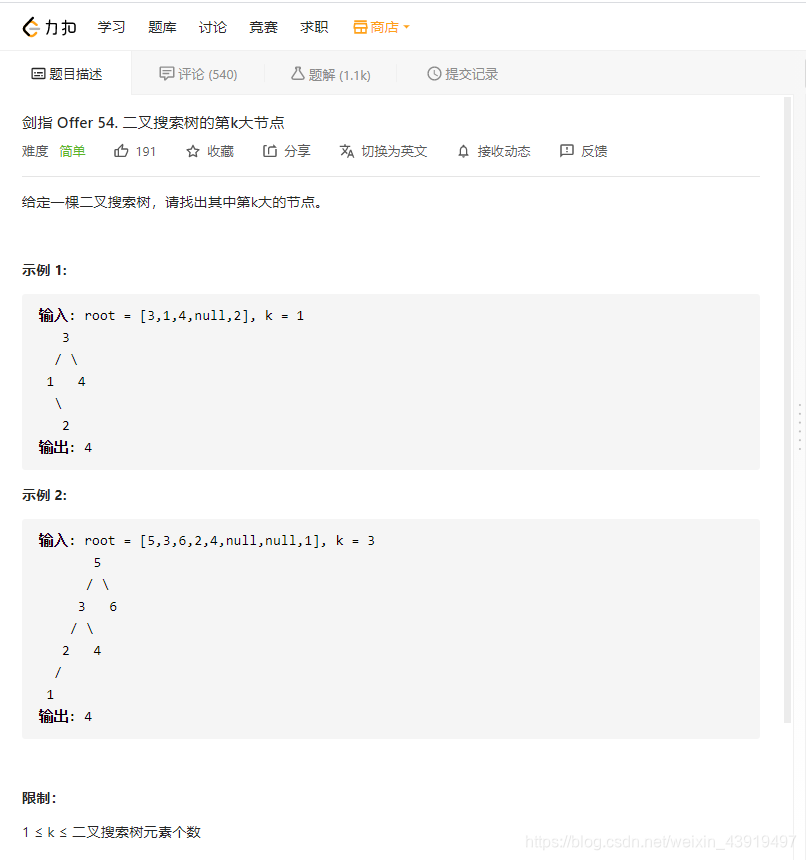
题解
很明显这题一看就会想到中序遍历、但这个是从大到小的遍历,所以遍历顺序是右 -> 中 -> 左 。 解法一:中序遍历+列表 自己先是使用了一个额外列表直接将遍历完的数字添加到列表中然后直接根据索引得出结果。这种时间负责度和空间复杂均为O(n)。
class Solution {
private ArrayList<Integer> res = new ArrayList<>();
public int kthLargest(TreeNode root, int k) {
inorderTraversal(root);
return res.get(k-1);
}
void inorderTraversal(TreeNode root){
if (root.left == null && root.right == null){
res.add(root.val);
return;
}
if (root.right != null){
inorderTraversal(root.right);
}
res.add(root.val);
if (root.left != null){
inorderTraversal(root.left);
}
}
}
解法二:中序遍历+剪枝 这种解法会遍历完树中所有的元素、其实只要遍历到第K个元素、然后其他的直接返回不需要继续遍历并且可以不用列表直接用一个变量接收到第k个元素即可。
class Solution {
private int r = 0, k = 0;
public int kthLargest(TreeNode root, int k) {
this.k = k;
inorderTraversal(root);
return r;
}
void inorderTraversal(TreeNode root) {
if (k == 0 || root == null) {
return;
}
inorderTraversal(root.right);
if (--k == 0) {
r = root.val;
return;
}
inorderTraversal(root.left);
}
}
|