题目:
将两个升序链表合并为一个新的 升序 链表并返回。新链表是通过拼接给定的两个链表的所有节点组成的。 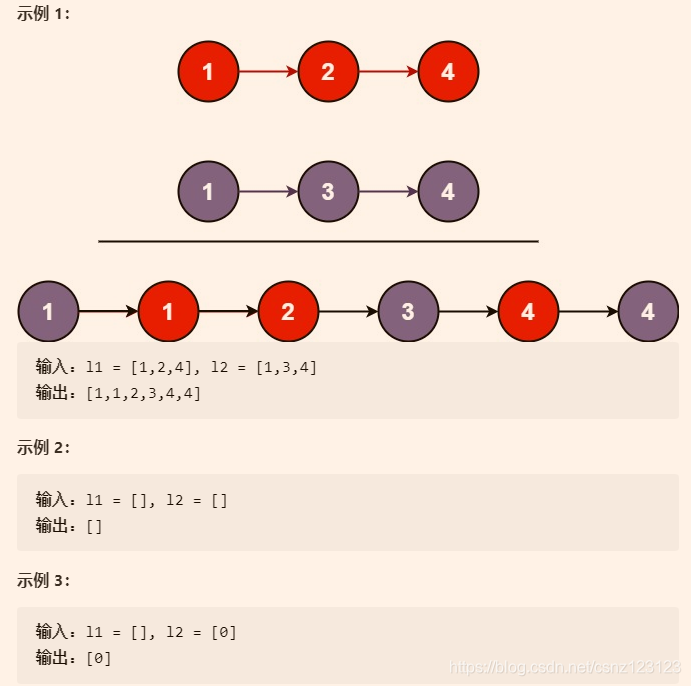
思路代码
public class ListNode {
int val;
ListNode next;
ListNode() {}
ListNode(int val) {
this.val = val;
}
ListNode(int val, ListNode next) {
this.val = val;
this.next = next;
}
}
class Solution {
public static ListNode mergeTwoLists(ListNode l1, ListNode l2) {
ListNode node = new ListNode();
ListNode p =node;
while(l1!=null && l2!=null) {
if(l1.val<=l2.val) {
p.next = l1;
l1 = l1.next;
}else {
p.next = l2;
l2 = l2.next;
}
p = p.next;
}
if(l1!=null) {
p.next = l1;
}
if(l2!=null) {
p.next = l2;
}
return node.next;
}
}
测试
public static void main(String[] args) {
ListNode node1 = new ListNode(1);
node1.next = new ListNode(2);
node1.next.next = new ListNode(4);
ListNode node2 = new ListNode(1);
node2.next = new ListNode(3);
node2.next.next = new ListNode(4);
ListNode list = mergeTwoLists(node1,node2);
while(list!=null) {
System.out.print(list.val+"\t");
list = list.next;
}
}
结果:  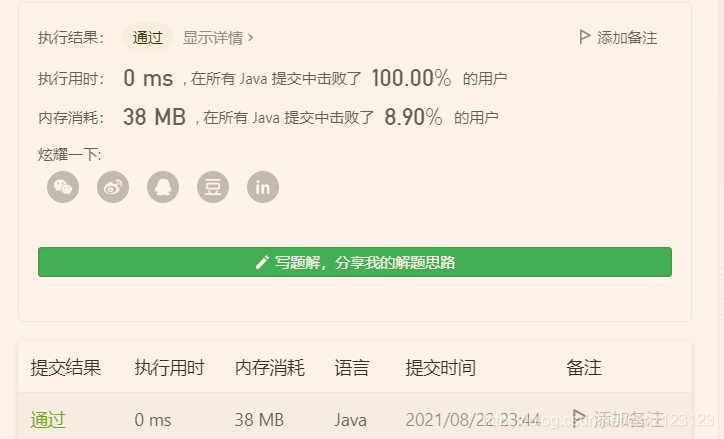
|