数据结构学习(C语言)–单链表的基本操作
#include<stdio.h>
#include<malloc.h>
typedef struct Node {
int data;
struct Node * pNext;
}*pNode, Node;
pNode createList();
void ergodicList(pNode);
bool isEmpty(pNode);
int getListLength(pNode);
bool insertByIndex(pNode, int, int);
bool deleteByIndex(pNode, int, int*);
bool deleteByValue(pNode, int);
bool serchByIndex(pNode, int);
bool serchByValue(pNode, int);
void selectSort(pNode);
pNode reverseOrder(pNode);
int main(void) {
pNode pHead = NULL;
int val;
int len;
pHead = createList();
ergodicList(pHead);
isEmpty(pHead);
len = getListLength(pHead);
printf_s("链表的长度为:%d\n", len);
insertByIndex(pHead, 3, 7);
ergodicList(pHead);
printf_s("此时链表长度为:%d\n", getListLength(pHead));
deleteByIndex(pHead, 3, &val);
ergodicList(pHead);
deleteByValue(pHead, 7);
ergodicList(pHead);
serchByIndex(pHead, 3);
serchByValue(pHead, 12);
selectSort(pHead);
ergodicList(pHead);
ergodicList(reverseOrder(pHead));
return 0;
}
pNode createList() {
int len;
int val;
int i;
pNode pHead;
pHead = (pNode)malloc(sizeof(Node));
pNode pTail = pHead;
pTail->pNext = NULL;
printf_s("请输入要创建链表的长度:");
scanf_s("%d", &len);
for (i = 0; i < len; i++) {
printf_s("请输入第%d个结点的值:", i + 1);
scanf_s("%d", &val);
pNode pNew = (pNode)malloc(sizeof(Node));
pNew->data = val;
pTail->pNext = pNew;
pNew->pNext = NULL;
pTail = pNew;
}
return pHead;
}
void ergodicList(pNode pHead) {
pNode p = pHead->pNext;
printf_s("链表中元素如下:\n");
while (p != NULL) {
printf_s("%d ", p->data);
p = p->pNext;
}
putchar('\n');
return;
}
bool isEmpty(pNode pHead) {
if (pHead->pNext == NULL) {
printf_s("链表为空!\n");
return true;
}
else {
printf_s("链表不为空!\n");
return false;
}
}
int getListLength(pNode pHead) {
pNode p = pHead->pNext;
int len = 0;
while (p != NULL) {
len++;
p = p->pNext;
}
return len;
}
bool insertByIndex(pNode pHead, int pos, int val) {
pNode p = pHead;
int i = 0;
if (pos<1 || pos>getListLength(pHead)) {
printf_s("您选择的插入位置不合法!\n");
return false;
}
while (i < pos) {
p = p->pNext;
i++;
}
pNode pNew = (pNode)malloc(sizeof(Node));
pNew->data = val;
pNew->pNext = p->pNext;
p->pNext = pNew;
printf_s("已将新结点插入到第%d个结点之后!\n", pos);
return true;
}
bool deleteByIndex(pNode pHead, int pos, int *val) {
int i = 0;
pNode p = pHead;
if (pos<1 || pos>getListLength(pHead)) {
printf_s("您选择的删除位置不合法!\n");
return false;
}
while (i < pos - 1) {
p = p->pNext;
i++;
}
pNode q = p->pNext;
*val = q->data;
p->pNext = q->pNext;
free(q);
q->pNext = NULL;
printf_s("已将第%d个值为%d结点删除!\n", pos, *val);
return true;
}
bool deleteByValue(pNode pHead, int val) {
int pos = 0;
int i = 0;
pNode p = pHead;
while (p->data != val) {
p = p->pNext;
pos++;
}
if (pos<1 || pos>getListLength(pHead)) {
printf_s("没有您要删除的数据\n");
return false;
}
p = pHead;
while (i < pos - 1) {
p = p->pNext;
i++;
}
pNode q = p->pNext;
p->pNext = q->pNext;
free(q);
q->pNext = NULL;
printf_s("已将值为%d的结点删除,其为第%d个结点\n", val, pos);
return true;
}
bool serchByIndex(pNode pHead, int pos) {
int i = 0;
if (pos<1 || pos>getListLength(pHead)) {
printf_s("查找位置不合法!\n");
return false;
}
pNode p = pHead;
for (i; i < pos; i++) {
p = p->pNext;
}
printf_s("第%d个结点的值是:%d\n", pos, p->data);
return true;
}
bool serchByValue(pNode pHead, int val) {
int pos = 0;
pNode p = pHead;
while (p->data != val) {
p = p->pNext;
pos++;
}
if (pos<1 || pos>getListLength(pHead)) {
printf_s("没有要查找的值!\n");
return false;
}
printf_s("第一个值为%d的结点为第%d个结点!\n", val, pos);
return true;
}
void selectSort(pNode pHead) {
pNode p;
pNode q;
int t;
int i, j;
for (i = 1, p = pHead->pNext; i < getListLength(pHead); i++, p = p->pNext) {
for (j = i + 1, q = p->pNext; j < getListLength(pHead) + 1; j++, q = q->pNext) {
if (p->data > q->data) {
t = p->data;
p->data = q->data;
q->data = t;
}
}
}
printf_s("已完成排序!\n");
return;
}
pNode reverseOrder(pNode pHead) {
if (pHead == NULL || pHead->pNext == NULL)
return pHead;
pNode top = NULL;
pNode mid = pHead->pNext;
pNode end = mid->pNext;
while (1) {
mid->pNext = top;
if (end == NULL) {
break;
}
top = mid;
mid = end;
end = end->pNext;
}
pHead->pNext = mid;
return pHead;
}
运行结果: 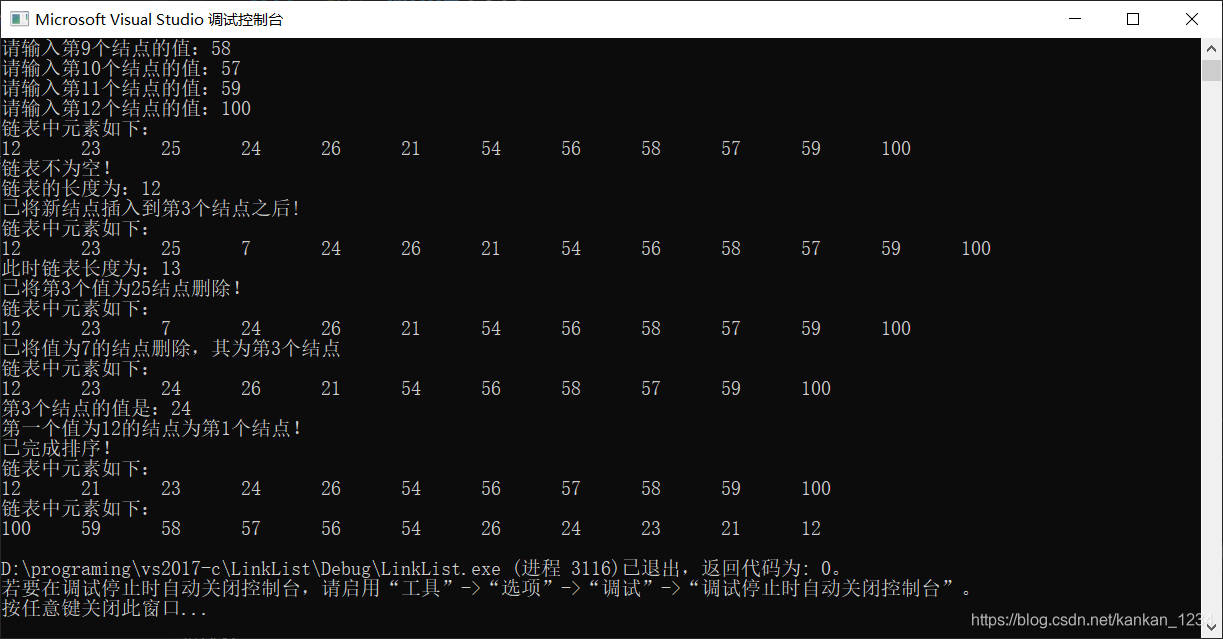 关于倒置(倒序输出)功能的说明: 倒序输出函数的方法可以参考文章单链表反转详解(4种算法实现)
|