检验括号是否匹配
检验括号匹配的方法,就是对给定的字符串依次检验:若是左括号,入栈;若是右括 号,出栈一个左括号判断是否与之相匹配;是其它字符,不检验。检验到字符串尾,还要 检查栈是否空。只有栈空,整个字符串才匹配完。
括号(()、[]和{})匹配的检验
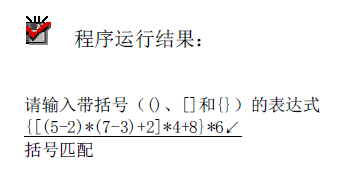 请输入带括号(()、[]和{})的表达式 {[(5-2)*(7-3)+2]*4+8}*6
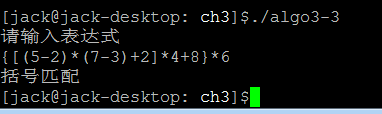
题目解析
(1)程序 提示 用户 ,手动输入 字符串
(2)对输入的字符串,逐个判断 如果是括号() [] {} ,包含 大括号,中括号,小括号 如果是左括号,就push到栈中, 如果是右括号,就从栈中pop出来。 看是否匹配,如果不匹配就报错。 (3) 在录入字符串时常使用到“gets”和“fgets”函数,但是两者都有一定的缺陷:
gets函数对录入的字符个数没有限制,容易造成越界 fgets函数会默认在字符串末尾加上"\n",影响数据的准确性
封装fgets函数,去掉其末尾的换行符"\n" https://blog.csdn.net/qq_43968080/article/details/84862198
首先写基本程序
#include<string.h>
#include<ctype.h>
#include<malloc.h>
#include<limits.h>
#include<stdio.h>
#include<stdlib.h>
#include <sys/io.h>
#include<math.h>
#include<pthread.h>
#define TRUE 1
#define FALSE 0
#define OK 1
#define ERROR 0
#define INFEASIBLE -1
typedef int Status;
typedef int Boolean;
typedef char ElemType;
#define STACK_INIT_SIZE 10
#define STACKINCREMENT 2
int main()
{
ElemType array[160];
int array_cnt = 0 ;
int i ;
ElemType ch ;
ElemType tmp_ch ;
ElemType contain[160];
int contain_index = 0 ;
printf("{1+()+(3+4+(5+6+6)+4+((((3+2)+3+(2+1)+((2+1+((+4+3)_+(22))))))))}\n");
printf("请输入 带括号的表达式:eg: {122 + (10/5)*(2x6)}\n");
memset(array,0,sizeof(array));
gets(array);
printf("输入内容为:%s\n",array);
array_cnt = strlen(array);
printf("输入长度为:%d\n",array_cnt);
for(i=0;i< array_cnt;i++){
ch = array[i];
switch(ch){
case '(':
case '[':
case '{':
contain[contain_index] = ch ;
contain_index++ ;
break;
case ')':
case ']':
case '}':
if(contain_index >0){
tmp_ch= contain[--contain_index];
if(tmp_ch == '(' && ch == ')'){
printf("小括号匹配\n");
}
else if(tmp_ch == '[' && ch == ']'){
printf("中括号匹配\n");
}
else if(tmp_ch == '{' && ch == '}'){
printf("大括号匹配\n");
}else{
printf("匹配错误\n");
return ERROR ;
}
}
break ;
}
}
return 0;
}
遇到问题
warning: the `gets’ function is dangerous and should not be used 解决办法是使用 fgets fgets()函数的基本用法为
fgets(char * s,int size,FILE * stream);
示例程序
#include <stdio.h>
int main ( ) {
char name[20];
printf("\n 输入任意字符 : ");
fgets(name, 20, stdin);
fputs(name, stdout);
return 0;
}
换成用栈的方式
#include<string.h>
#include<ctype.h>
#include<malloc.h>
#include<limits.h>
#include<stdio.h>
#include<stdlib.h>
#include <sys/io.h>
#include<math.h>
#include<pthread.h>
#define TRUE 1
#define FALSE 0
#define OK 1
#define ERROR 0
#define INFEASIBLE -1
typedef int Status;
typedef int Boolean;
typedef char ElemType;
#define STACK_INIT_SIZE 60
#define STACKINCREMENT 2
typedef struct sqStack{
ElemType *base ;
ElemType *top ;
int stack_size ;
}sqStack;
Status init_stack(sqStack * S)
{
S->base = (ElemType *)malloc(sizeof(ElemType)*STACK_INIT_SIZE);
S->top = S->base ;
S->stack_size = STACK_INIT_SIZE ;
return OK;
}
Status push_stack(sqStack *S, ElemType e)
{
if(S->top - S->base < S->stack_size){
*(S->top++) = e ;
return OK ;
}
return ERROR;
}
Boolean empty_stack(sqStack *S)
{
if(S->top == S->base){
return TRUE;
}
return FALSE ;
}
Status pop_stack(sqStack *S, ElemType *e)
{
if(!empty_stack(S)){
*e = *(--(S->top));
return OK;
}
return ERROR;
}
int main()
{
ElemType array[160];
int array_cnt = 0 ;
int i ;
ElemType ch ;
ElemType tmp_ch ;
sqStack S ;
printf("{1+()+(3+4+(5+6+6)+4+((((3+2)+3+(2+1)+((2+1+((+4+3)_+(22))))))))}\n");
printf("请输入 带括号的表达式:eg: {122 + (10/5)*(2x6)}\n");
memset(array,0,sizeof(array));
init_stack(&S);
fgets(array,sizeof(array), stdin);
char *find = strchr(array, '\n');
if(find)
*find = '\0';
printf("输入内容为:%s\n",array);
array_cnt = strlen(array);
printf("输入长度为:%d\n",array_cnt);
for(i=0;i< array_cnt;i++){
ch = array[i];
switch(ch){
case '(':
case '[':
case '{':
push_stack(&S,ch);
break;
case ')':
case ']':
case '}':
if(pop_stack(&S,&tmp_ch) == OK){
if(tmp_ch == '(' && ch == ')'){
printf("小括号匹配\n");
}
else if(tmp_ch == '[' && ch == ']'){
printf("中括号匹配\n");
}
else if(tmp_ch == '{' && ch == '}'){
printf("大括号匹配\n");
}else{
printf("匹配错误\n");
return ERROR ;
}
}
else{
printf("栈为空,匹配错误\n");
return ERROR ;
}
break ;
}
}
return 0;
}
|