数组最大的优点:快速查询。 数组最好应用于索引有语义的情况。 并非所有有语义的数字都可以作为数组的索引,例xaaacaacsaaacsaawcacc
String str="xaaacaacsaaacsaawcacc";
int[]counts=new int[26];
for (int i=0;i<str.length();i++){
char c=str.charAt(i);
counts[c-'a']++;
}
for (int item:counts){
System.out.println(item);
}
Map<Character,Integer>map=new HashMap<>();
for (int i=0;i<str.length();i++){
char c=str.charAt(i);
if(!map.containsKey(c)){
map.put(c,1);
}else{
map.put(c,map.get(c)+1);
}
System.out.print(c);
}
向数组中添加元素
向数组的尾部添加元素
public void addTail(T ele) {
add(this.size, ele);
}
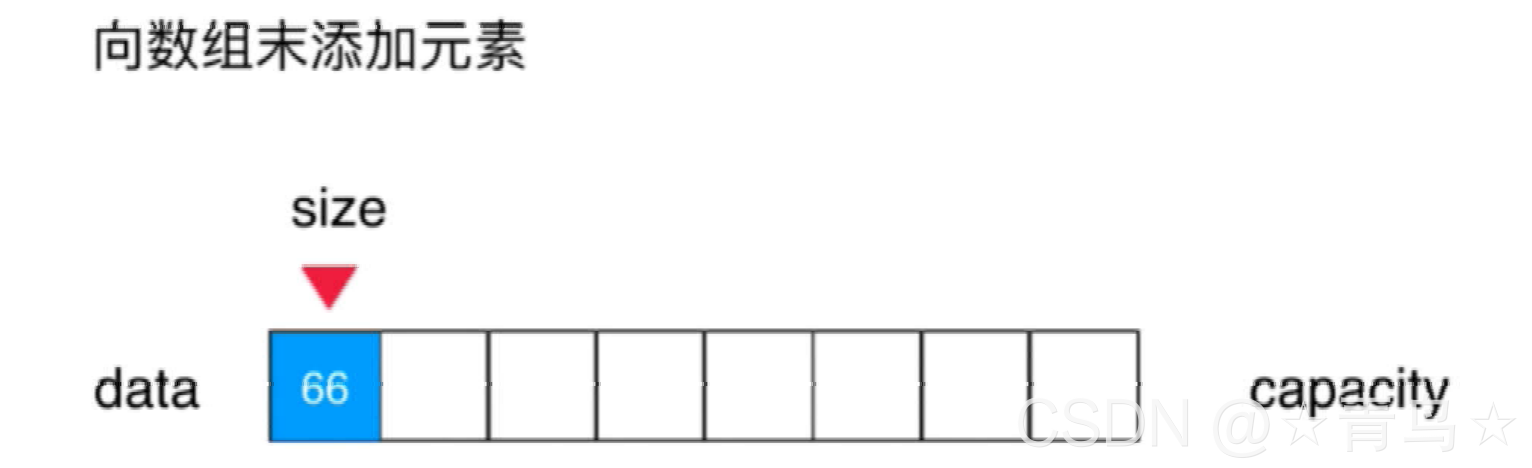
在数组的头部添加
public void addHead(T ele) {
add(0, ele);
}
在指定位置添加元素
public void add(int index, T ele) {
if (index < 0 || index > size) {
throw new IllegalArgumentException("index is error");
}
if (this.size == data.length) {
resize(2 * data.length);
}
for (int i = size - 1; i >= index; i--) {
data[i + 1] = data[i];
}
data[index] = ele;
this.size++;
}
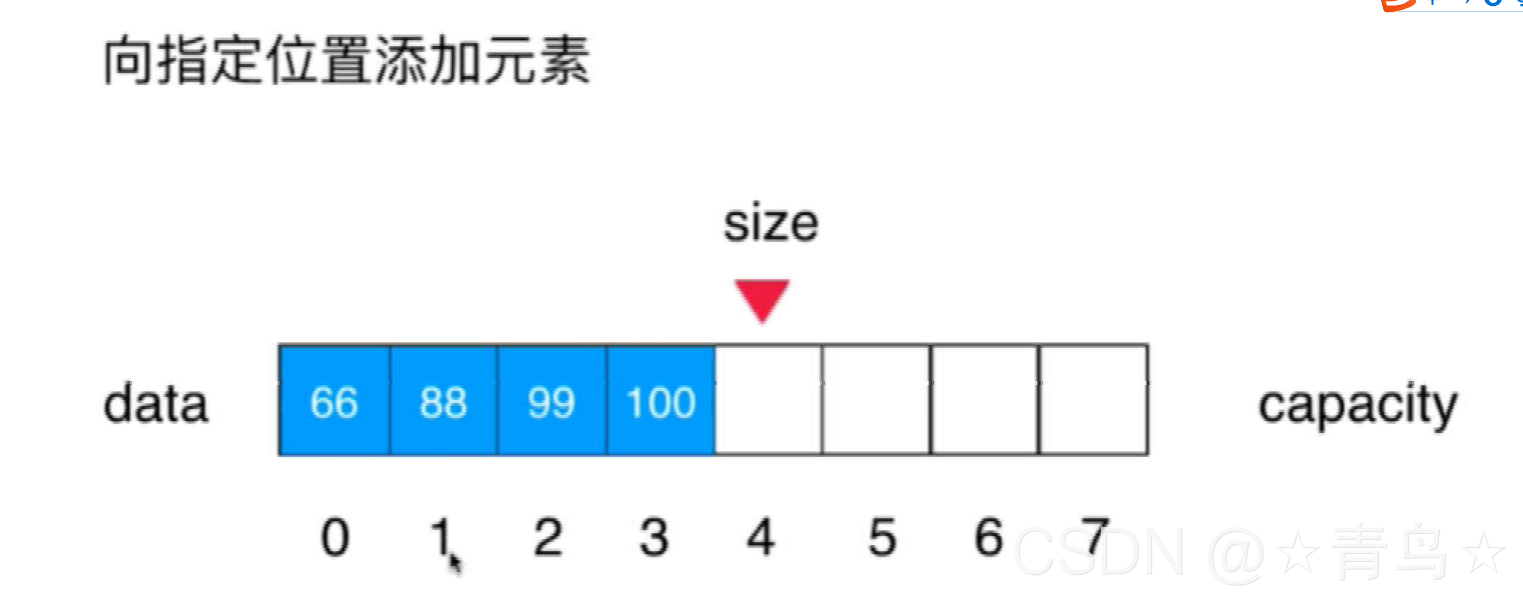 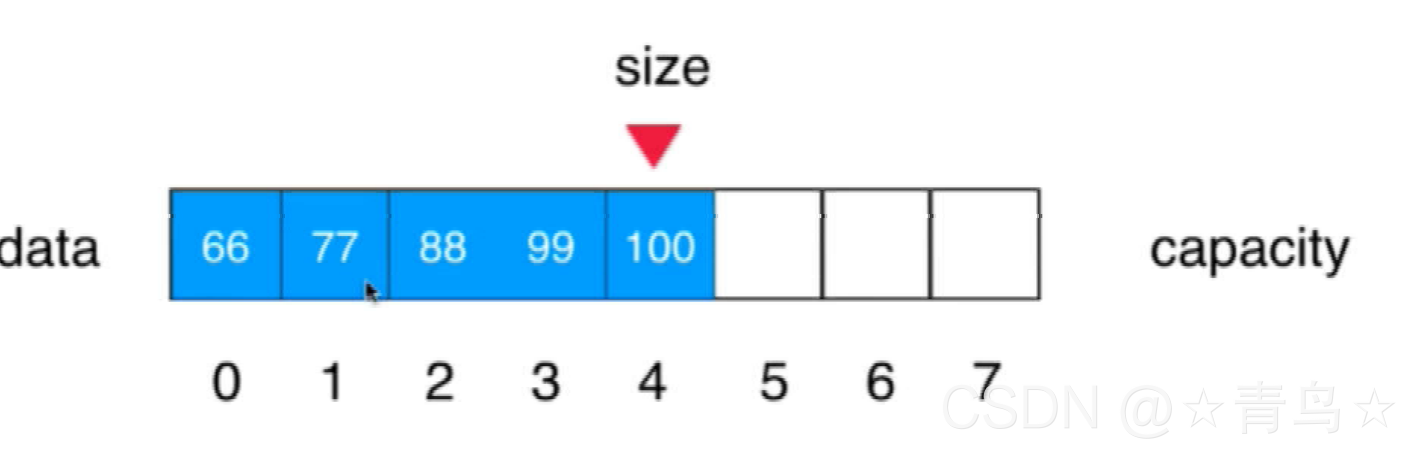
更改数组的容积
public void resize(int newCapacity) {
T[] newData = (T[]) new Object[newCapacity];
for (int i = 0; i < this.size; i++) {
newData[i] = data[i];
}
this.data = newData;
}
获取指定位置的元素
public T getEleByIndex(int index) {
if (index < 0 || index >= size) {
throw new IllegalArgumentException("index is error");
}
return data[index];
}
修改指定位置的元素
public void updataByIndex(int index, T ele) {
if (index < 0 || index >= size) {
throw new IllegalArgumentException("index is error");
}
data[index] = ele;
}
是否包含指定的元素内容
public int isContains(T ele) {
for (int i = 0; i < this.size; i++) {
if (this.data[i].equals(ele)) {
return i;
}
}
return -1;
}
删除指定位置的元素
public T remove(int index) {
if (index < 0 || index >= size) {
throw new IllegalArgumentException("index is invalid!");
}
T result = data[index];
for (int i = index + 1; i < size; i++) {
data[i - 1] = data[i];
}
this.size--;
data[size] = null;
if (this.size == data.length / 4 && data.length / 4 > 0) {
resize(data.length / 2);
}
return result;
}
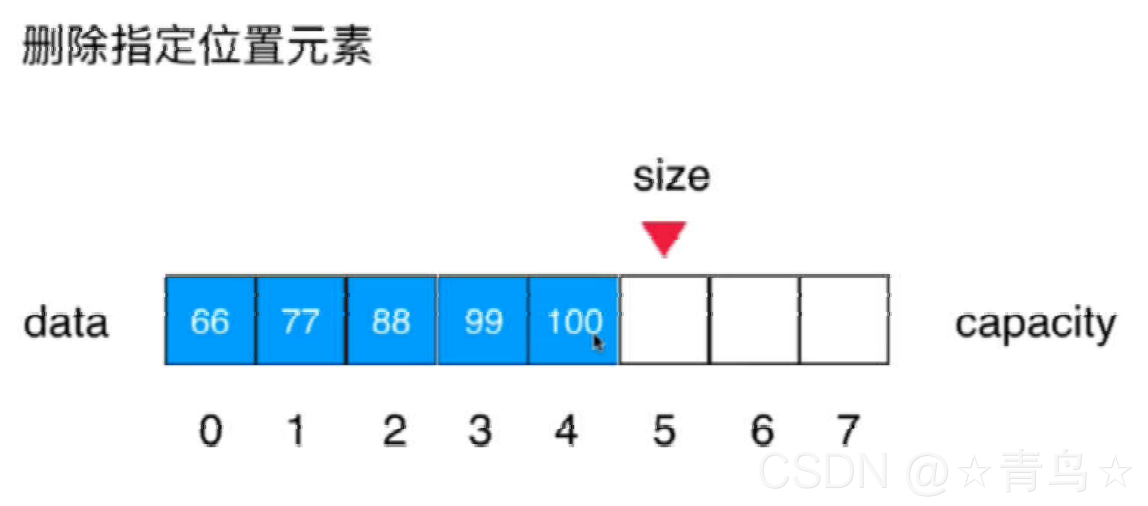
移除头部元素
public T removeHead() {
return remove(0);
}
移除尾部元素
public T removeTail() {
return remove(this.size - 1);
}
删除指定的元素
public int removeEle(T ele) {
int index = isContains(ele);
if (index != -1) {
remove(index);
}
return index;
}
public static void main(String[] args) {
MySelfArray<Integer> mySelfArray = new MySelfArray<>(10);
long startTime = System.nanoTime();
int count = 10;
Random random = new Random();
for (int i = 0; i < count; i++) {
mySelfArray.addTail(random.nextInt(Integer.MAX_VALUE));
}
System.out.println("数组:" + mySelfArray);
while (!mySelfArray.isEmpty()) {
mySelfArray.removeTail();
}
long endTime = System.nanoTime();
System.out.println("总耗时:" + (endTime - startTime) / 1000000000.0);
System.out.println("10是否存在:" + (mySelfArray.isContains(10) == -1 ? false : true));
MySelfArray<String> strArray = new MySelfArray<>(5);
strArray.addTail("apple");
strArray.addTail("pear");
strArray.addTail("banana");
strArray.addTail("pineapple");
strArray.addTail("watermelon");
System.out.println(strArray);
System.out.println(strArray.isContains("watermelon") == -1 ? false : true);
}
|