题目描述
请实现 copyRandomList 函数,复制一个复杂链表。在复杂链表中,每个节点除了有一个 next 指针指向下一个节点,还有一个 random 指针指向链表中的任意节点或者 null。
示例 1:
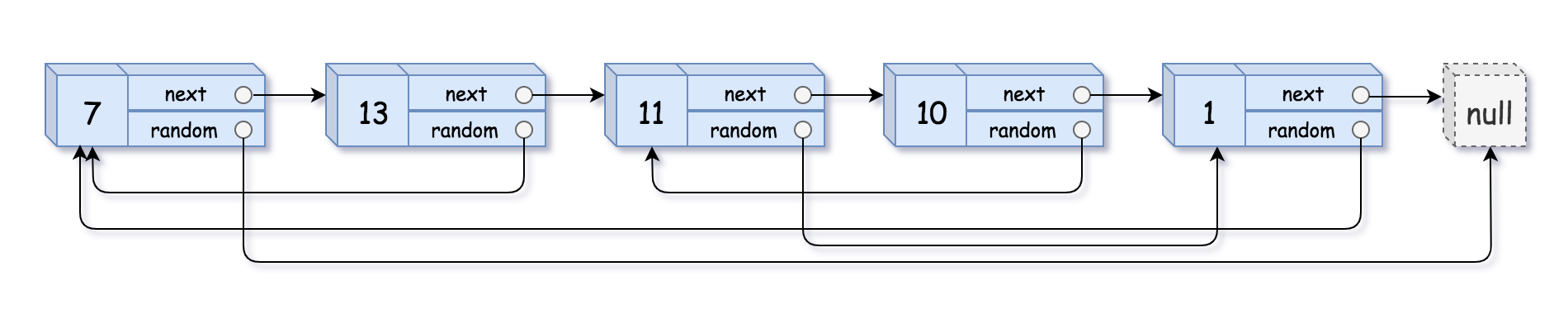
输入:head = [[7,null],[13,0],[11,4],[10,2],[1,0]] 输出:[[7,null],[13,0],[11,4],[10,2],[1,0]] 示例 2:

输入:head = [[1,1],[2,1]]
输出:[[1,1],[2,1]]
示例 3:

输入:head = [[3,null],[3,0],[3,null]] 输出:[[3,null],[3,0],[3,null]] 示例 4:
输入:head = [] 输出:[] 解释:给定的链表为空(空指针),因此返回 null。
提示:
-10000 <= Node.val <= 10000 Node.random?为空(null)或指向链表中的节点。 节点数目不超过 1000 。
?复制节点+拆分链表
/*
// Definition for a Node.
class Node {
int val;
Node next;
Node random;
public Node(int val) {
this.val = val;
this.next = null;
this.random = null;
}
}
*/
class Solution {
public Node copyRandomList(Node head) {
if(head==null)
return head;
//复制各节点,构造拼接链表
Node p=head;
while(p!=null)
{
Node newNode=new Node(p.val);
newNode.next=p.next;
p.next=newNode;
p=p.next.next;
}
//构建各复制节点的random指向
p=head;
while(p!=null)
{
if(p.random!=null)
p.next.random=p.random.next;
p=p.next.next;
}
//拆分两链表:原节点与复制节点同时拆分
p=head;
Node result=head.next;
while(p!=null&&p.next!=null)
{
Node now=p.next;
p.next=p.next.next;
if(now.next!=null)
now.next=now.next.next;
else
now.next=null;
p=p.next;
}
return result;
}
}
|