- K 个一组翻转链表
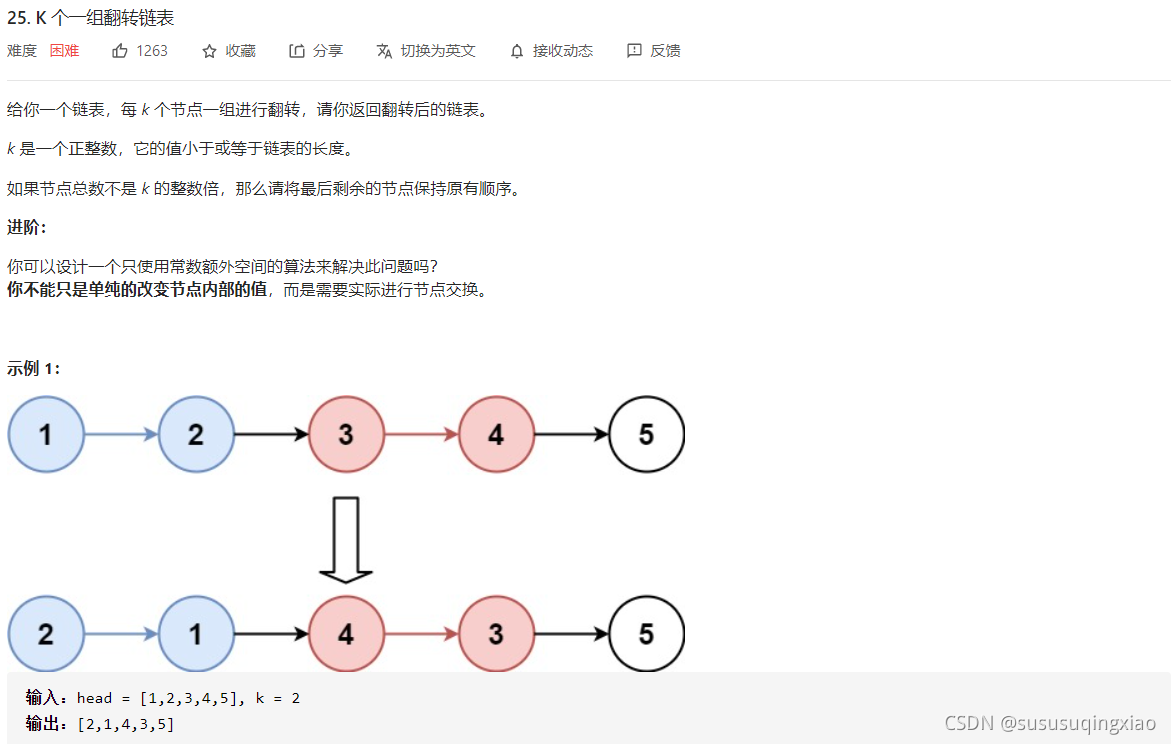
class Solution {
public:
pair<ListNode*, ListNode*> myReverse(ListNode* head, ListNode* tail) {
ListNode* prev = tail->next;
ListNode* cur = head;
while (prev != tail) {
ListNode* nex = cur->next;
cur->next = prev;
prev = cur;
cur = nex;
}
return {tail, head};
}
ListNode* reverseKGroup(ListNode* head, int k) {
ListNode* hair = new ListNode(0);
hair->next = head;
ListNode* pre = hair;
while (head) {
ListNode* tail = pre;
for (int i = 0; i < k; ++i)
{
tail = tail->next;
if (!tail) {
return hair->next;
}
}
ListNode* nex = tail->next;
tie(head, tail) = myReverse(head, tail);
pre->next = head;
tail->next = nex;
pre = tail;
head = tail->next;
}
return hair->next;
}
};
2、反转+递归
class Solution {
public:
ListNode* myReverse(ListNode* a, ListNode* b)
{
ListNode* pre = nullptr;
ListNode* cur = a;
ListNode* nex = a;
while (cur != b)
{
nex = cur->next;
cur->next = pre;
pre = cur;
cur = nex;
}
return pre;
}
ListNode* reverseKGroup(ListNode* head, int k)
{
if (head == nullptr)
return head;
ListNode* a = head;
ListNode* b = head;
for (int i = 0; i < k; ++i)
{
if (b == nullptr)
return head;
b = b->next;
}
ListNode* newHead = myReverse(a, b);
a->next = reverseKGroup(b, k);
return newHead;
}
};
|