1. 排序概述
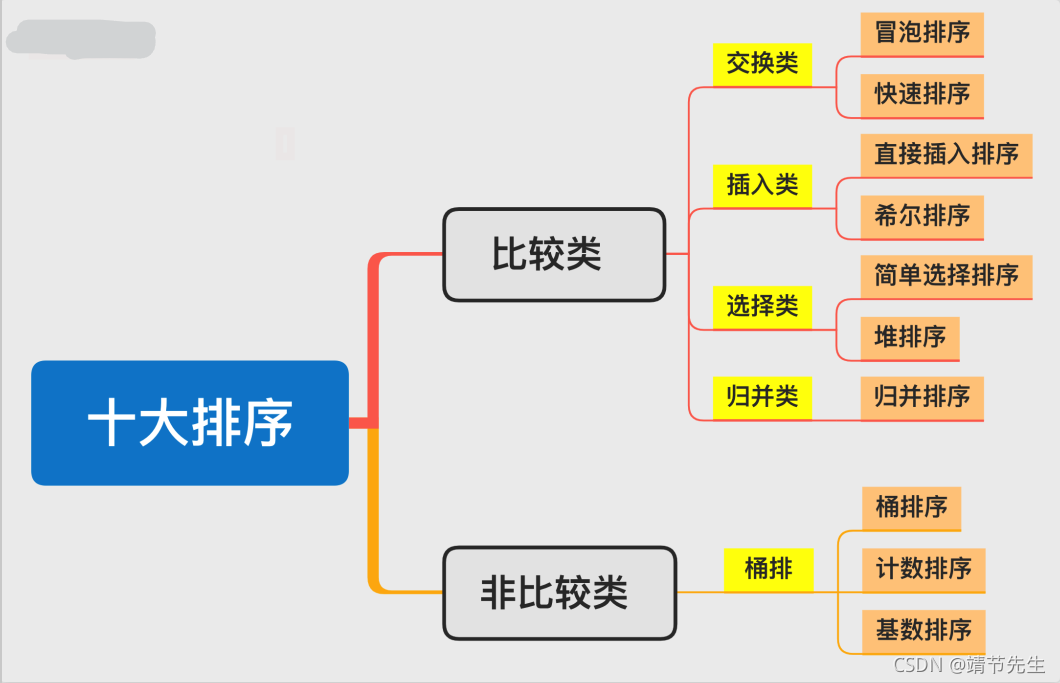
2. 排序实现
2.1 冒泡排序
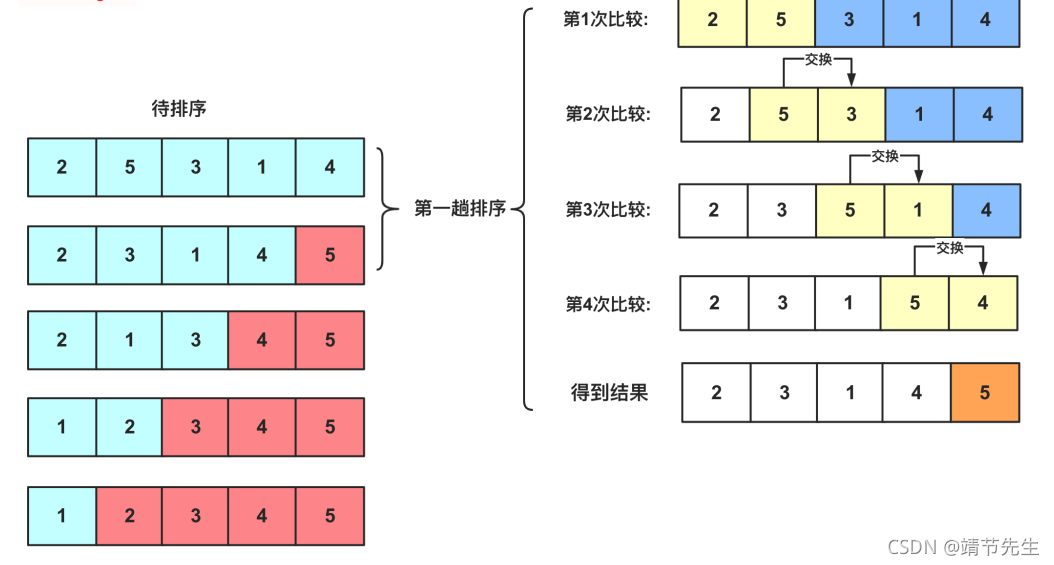
2.2 快速排序
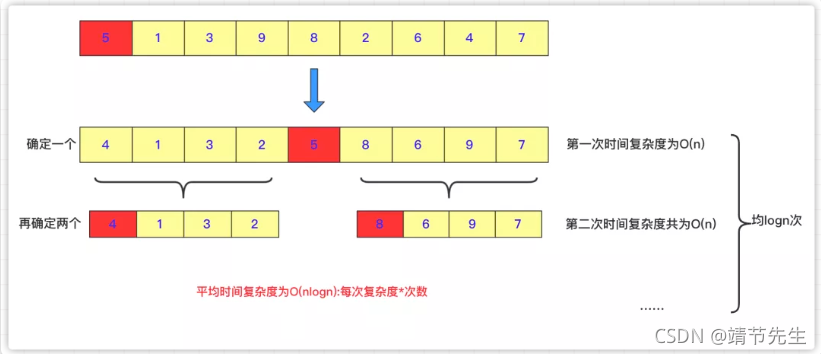
2.2 直接插入排序
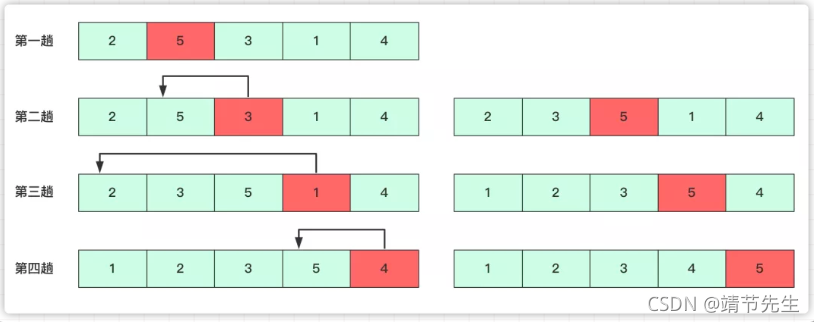
2.2 希尔排序
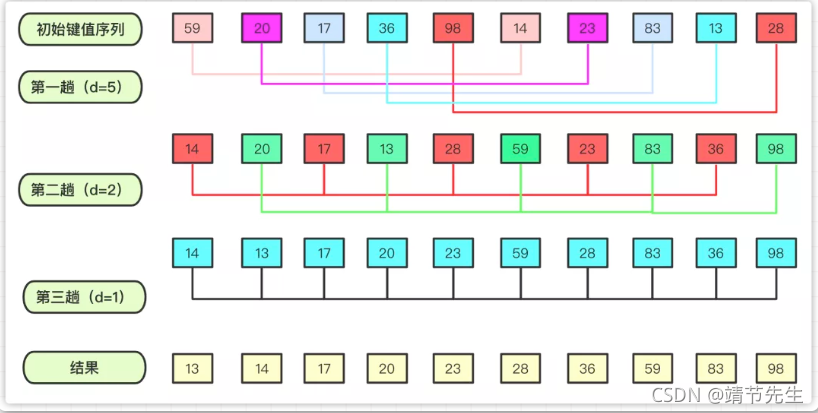
2.2 简单选择排序
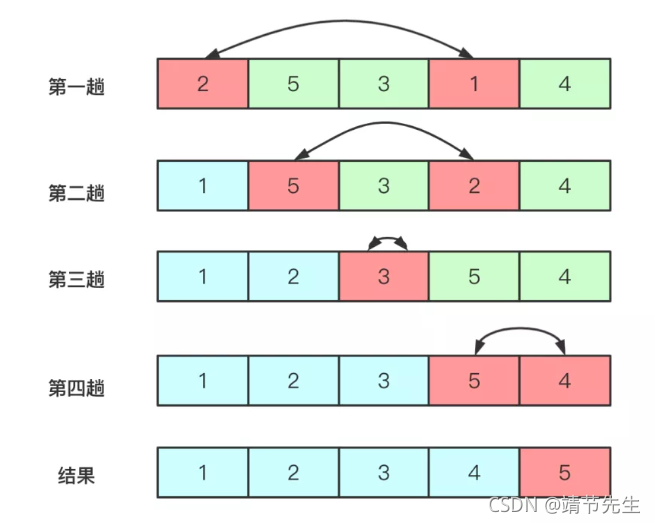
2.2 堆排序
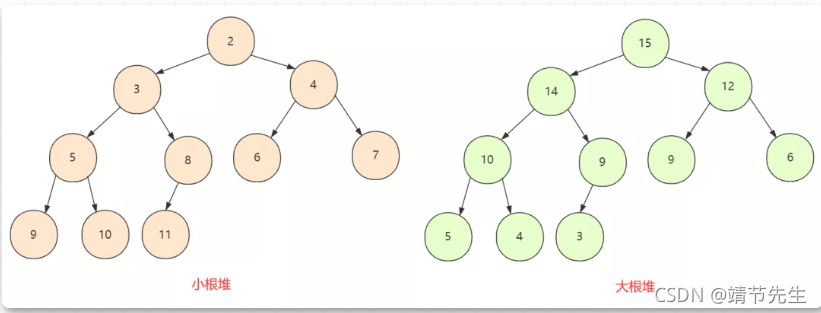 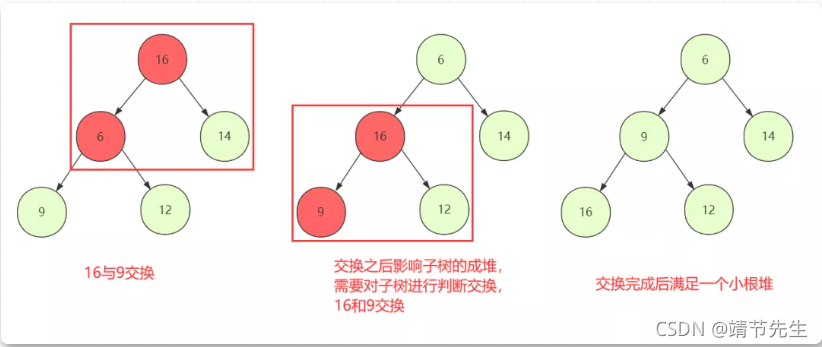
2.2 归并排序
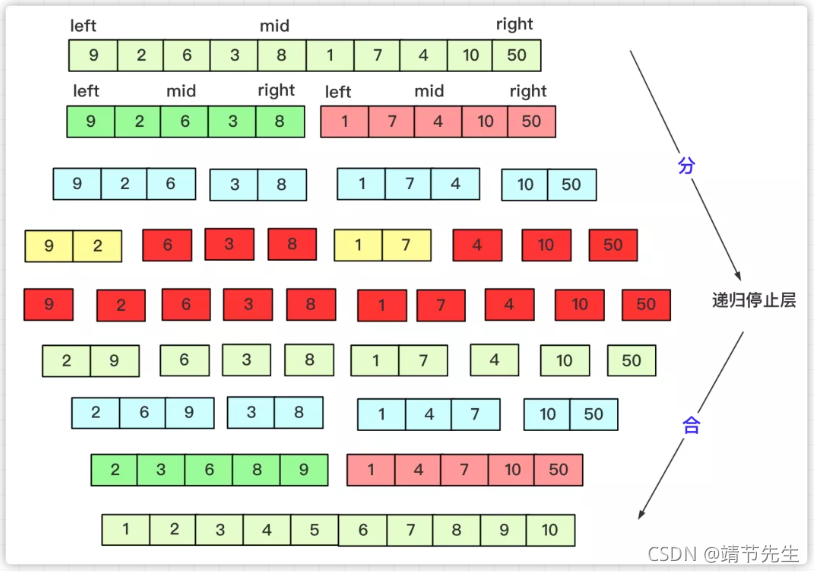
2. 代码实现
package com.zrj.algorithm.sort;
import com.alibaba.fastjson.JSON;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class SortData {
public static void main(String[] args) {
int[] array = {2, 5, 3, 1, 4};
bubbleSort( array );
insertSort( array );
hillSort( array );
selectSort( array );
quickSort( array, 0, array.length - 1 );
}
public static void bubbleSort(int[] array) {
for (int i = array.length - 1; i >= 0; i--) {
for (int j = 0; j < i; j++) {
if (array[j] > array[j + 1]) {
int variable = array[j];
array[j] = array[j + 1];
array[j + 1] = variable;
}
}
}
System.out.println( "冒泡排序:" + JSON.toJSONString( array ) );
}
public static void quickSort(int[] array, int left, int right) {
int low = left;
int high = right;
if (low > high) {
return;
}
int k = array[low];
while (low < high) {
while (low < high && array[high] >= k) {
high--;
}
array[low] = array[high];
while (low < high && array[low] <= k) {
low++;
}
array[high] = array[low];
}
array[low] = k;
quickSort( array, left, low - 1 );
quickSort( array, low + 1, right );
System.out.println( "快速排序:" + JSON.toJSONString( array ) );
}
public static void insertSort(int[] array) {
int variable;
for (int i = 0; i < array.length; i++) {
variable = array[i];
for (int j = i - 1; j >= 0; j--) {
if (array[j] > variable) {
array[j + 1] = array[j];
array[j] = variable;
} else {
break;
}
}
}
System.out.println( "直接插入排序:" + JSON.toJSONString( array ) );
}
public static void hillSort(int[] array) {
int d = array.length;
int variable;
for (; d >= 1; d /= 2) {
for (int i = d; i < array.length; i++) {
variable = array[i];
for (int j = i - d; j >= 0; j -= d) {
if (array[j] > variable) {
array[j + d] = array[j];
array[j] = variable;
} else {
break;
}
}
}
}
System.out.println( "希尔排序:" + JSON.toJSONString( array ) );
}
public static void selectSort(int[] arr) {
for (int i = 0; i < arr.length - 1; i++) {
int min = i;
for (int j = i + 1; j < arr.length; j++) {
if (arr[j] < arr[min]) {
min = j;
}
}
if (min != i) {
swap( arr, i, min );
}
}
System.out.println( "简单选择排序:" + JSON.toJSONString( arr ) );
}
private static void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
static void shiftDown(int arr[], int index, int len) {
int leftchild = index * 2 + 1;
int rightchild = index * 2 + 2;
if (leftchild >= len) {
return;
} else if (rightchild < len && arr[rightchild] < arr[index] && arr[rightchild] < arr[leftchild]) {
swap( arr, index, rightchild );
shiftDown( arr, rightchild, len );
} else if (arr[leftchild] < arr[index]) {
swap( arr, index, leftchild );
shiftDown( arr, leftchild, len );
}
}
static void creatHeap(int arr[]) {
for (int i = arr.length / 2; i >= 0; i--) {
shiftDown( arr, i, arr.length );
}
}
static void heapSort(int arr[]) {
System.out.println( "原始数组为:" + Arrays.toString( arr ) );
int val[] = new int[arr.length];
creatHeap( arr );
System.out.println( "建堆后的序列为:" + Arrays.toString( arr ) );
for (int i = 0; i < arr.length; i++) {
val[i] = arr[0];
arr[0] = arr[arr.length - 1 - i];
shiftDown( arr, 0, arr.length - i );
}
for (int i = 0; i < arr.length; i++) {
arr[i] = val[i];
}
System.out.println( "堆排序后的序列为:" + Arrays.toString( arr ) );
}
private static void mergesort(int[] array, int left, int right) {
int mid = (left + right) / 2;
if (left < right) {
mergesort( array, left, mid );
mergesort( array, mid + 1, right );
merge( array, left, mid, right );
}
}
private static void merge(int[] array, int l, int mid, int r) {
int lindex = l;
int rindex = mid + 1;
int team[] = new int[r - l + 1];
int teamindex = 0;
while (lindex <= mid && rindex <= r) {
if (array[lindex] <= array[rindex]) {
team[teamindex++] = array[lindex++];
} else {
team[teamindex++] = array[rindex++];
}
}
while (lindex <= mid) {
team[teamindex++] = array[lindex++];
}
while (rindex <= r) {
team[teamindex++] = array[rindex++];
}
for (int i = 0; i < teamindex; i++) {
array[l + i] = team[i];
}
}
public static void bucketSort() {
int a[] = {1, 8, 7, 44, 42, 46, 38, 34, 33, 17, 15, 16, 27, 28, 24};
List[] buckets = new ArrayList[5];
for (int i = 0; i < buckets.length; i++) {
buckets[i] = new ArrayList<Integer>();
}
for (int i = 0; i < a.length; i++) {
int index = a[i] / 10;
buckets[index].add( a[i] );
}
for (int i = 0; i < buckets.length; i++) {
buckets[i].sort( null );
for (int j = 0; j < buckets[i].size(); j++)
{
System.out.print( buckets[i].get( j ) + " " );
}
}
}
public static void countSort(int[] a) {
int min = Integer.MAX_VALUE;
int max = Integer.MIN_VALUE;
for (int i = 0; i < a.length; i++) {
if (a[i] < min) {
min = a[i];
}
if (a[i] > max) {
max = a[i];
}
}
int[] count = new int[max - min + 1];
for (int i = 0; i < a.length; i++) {
count[a[i] - min]++;
}
int index = 0;
for (int i = 0; i < count.length; i++) {
while (count[i]-- > 0) {
a[index++] = i + min;
}
}
}
public static void radixSort(int[] arr) {
{
List<Integer>[] bucket = new ArrayList[10];
for (int i = 0; i < 10; i++) {
bucket[i] = new ArrayList<>();
}
int max = 0;
for (int i = 0; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
int divideNum = 1;
while (max > 0) {
for (int num : arr) {
bucket[(num / divideNum) % 10].add( num );
}
divideNum *= 10;
max /= 10;
int idx = 0;
for (List<Integer> list : bucket) {
for (int num : list) {
arr[idx++] = num;
}
list.clear();
}
}
}
}
}
|