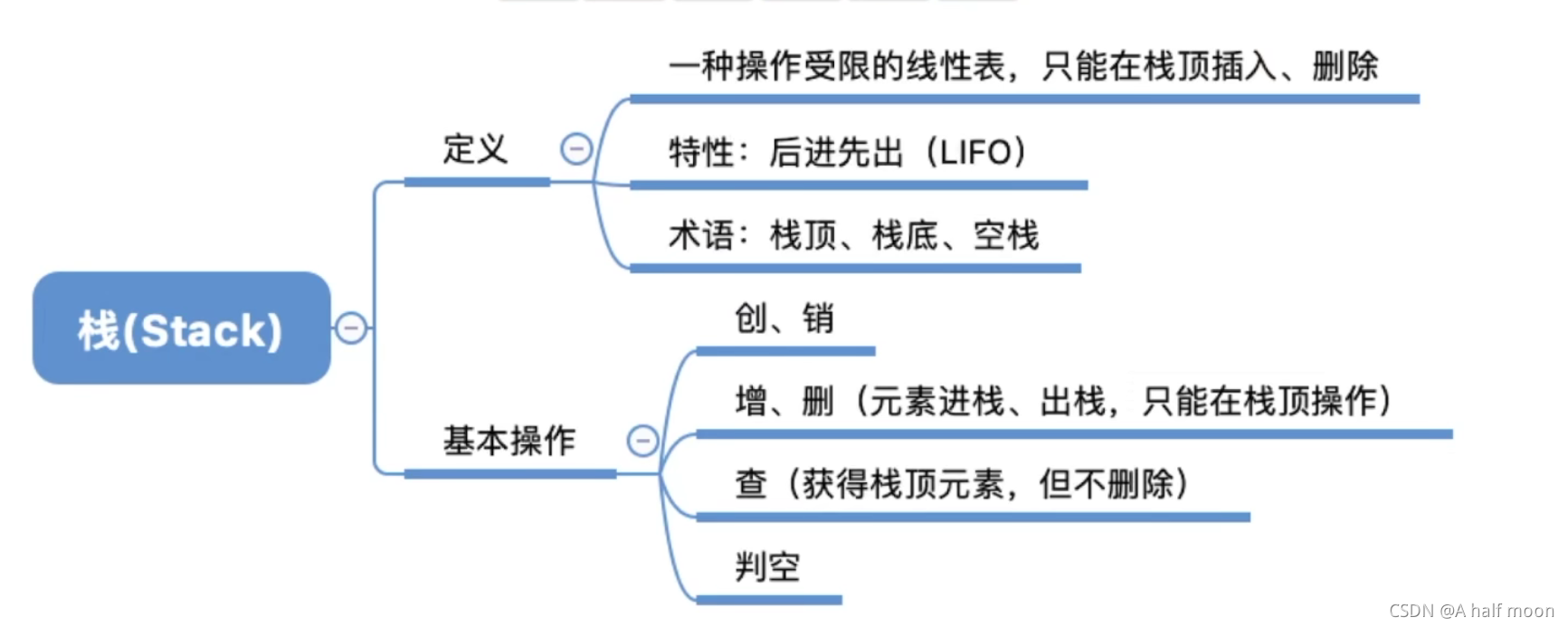 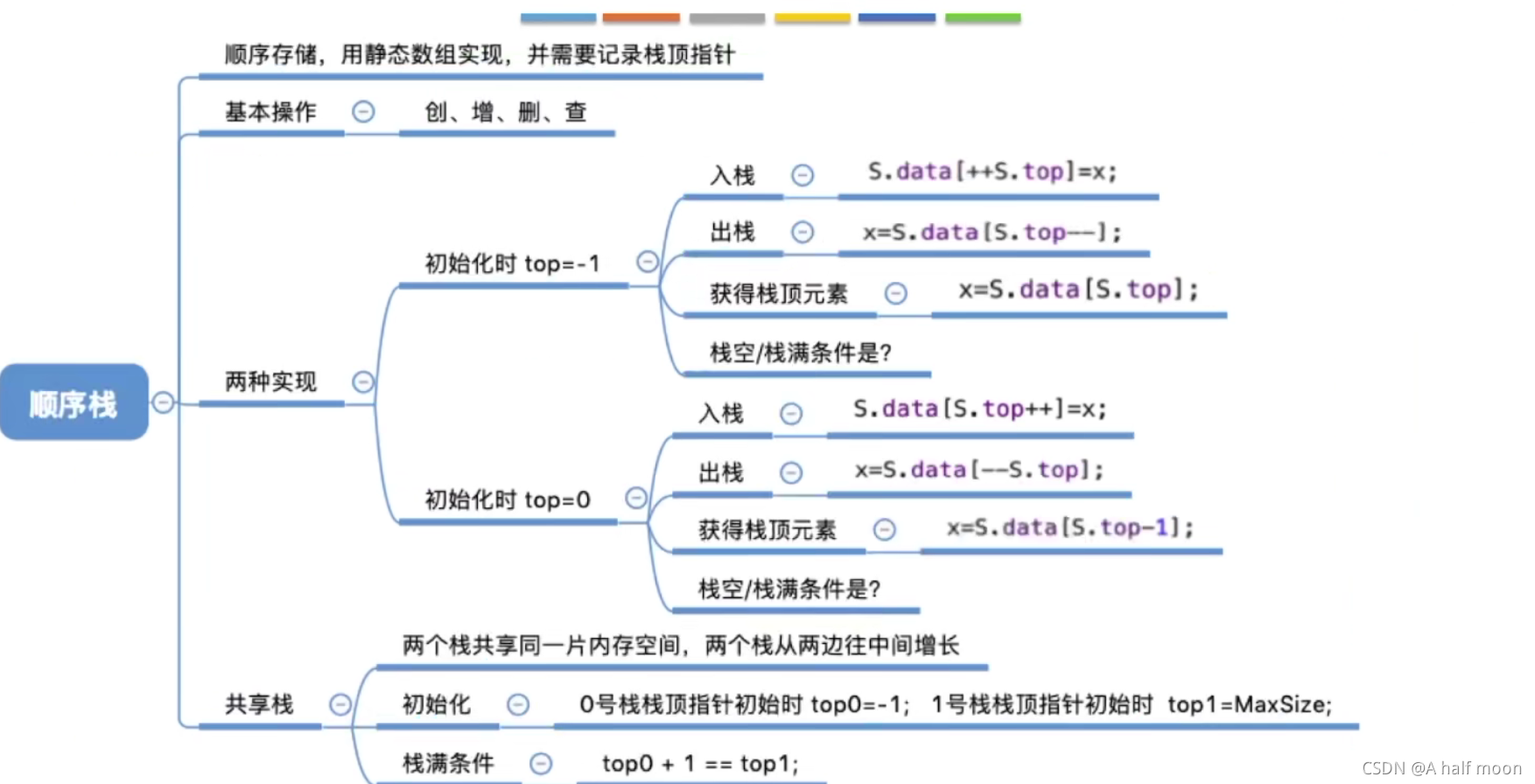 顺序存储方式实现栈代码(以s.top= -1为例)
#include<stdio.h>
#include<iostream>
#define Maxsize 10
typedef struct{
int data[Maxsize];
int top;
}SqStack;
void InitStack(SqStack &S)
{
S.top=-1;
}
bool StackEmpty(SqStack S)
{
if(S.top==-1)
return true;
else
return false;
}
bool Push(SqStack &S,int x)
{
if(S.top==Maxsize-1)
return false;
S.top=S.top+1;
S.data[S.top]=x;
}
bool Pop(SqStack &S, int &x)
{
if(S.top==-1)
return false;
x=S.data[S.top];
S.top=S.top-1;
return true;
}
bool GetTop(SqStack &S,int &x)
{
if(S.top==-1)
return false;
x=S.data[S.top];
return true;
}
void Show(SqStack S)
{
for(int i=0;i<S.top+1;i++)
{
printf("%d ",S.data[i]);
}
printf("\nLength: %d\n",S.top+1);
}
int main()
{
SqStack S;
InitStack(S);
Push(S,1);
Push(S,2);
Push(S,3);
Push(S,4);
Push(S,5);
Show(S);
int x=0;
Pop(S,x);
int top;
GetTop(S,top);
printf("已将栈顶元素%d出栈,出栈后的栈顶元素为%d\n",x,top);
Show(S);
return 0;
}
代码运行结果 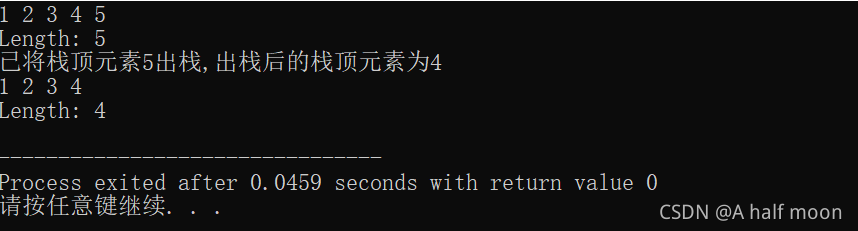 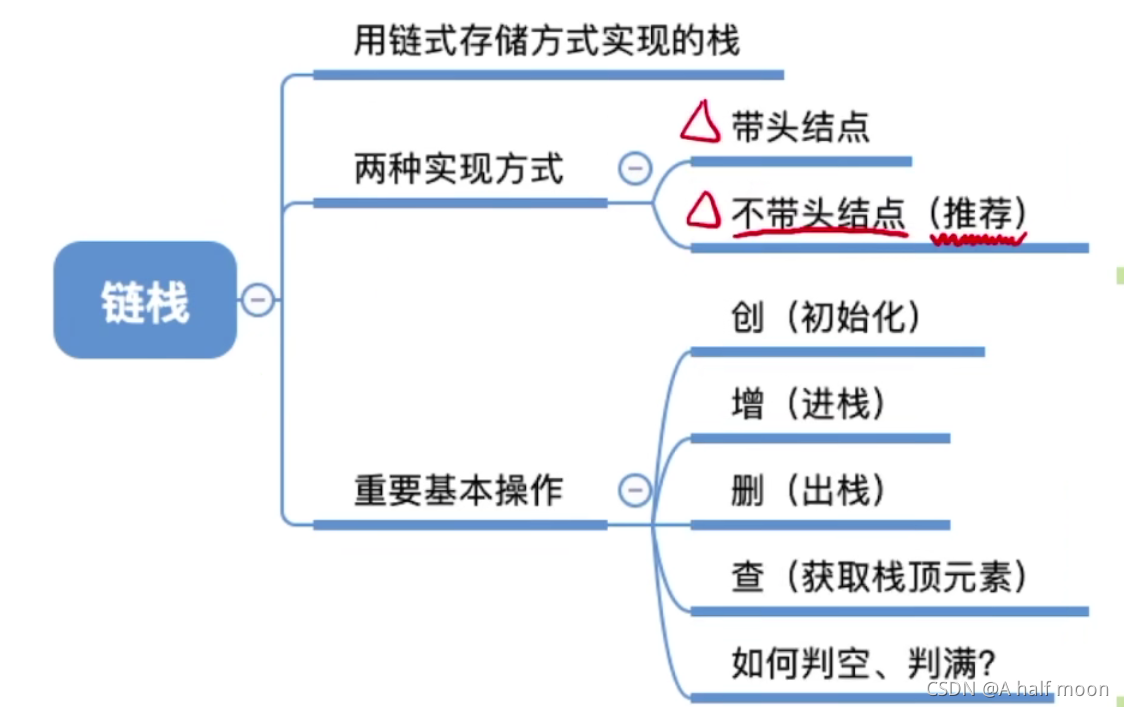 链式方式实现栈代码(相当单链表只在头结点插入与删除)
#include<stdio.h>
#include<cstdlib>
#include<iostream>
typedef struct LNode{
int data;
struct LNode *next;
}LNode,*LinkStack;
bool InitList(LinkStack &L)
{
L=(LNode*)malloc(sizeof(LNode));
if(L==NULL)
return false;
L->next=NULL;
return true;
}
bool Empty(LinkStack L){
if(L->next==NULL)
return true;
else
return false;
}
LNode * GetElem(LinkStack L,int i) {
if(i<0)
return NULL;
LNode *p;
int j=0;
p=L;
while(p!=NULL&&j<i)
{
p=p->next;
j++;
}
if(p==NULL)
return false;
return p;
}
LNode * LocateElem(LinkStack L ,int e)
{
LNode *p=L->next;
while(p!=NULL&&p->data!=e)
{
p=p->next;
}
return p;
}
int Length(LinkStack L)
{
int len=0;
LNode *p=L;
while(p->next!=NULL)
{
p=p->next;
len++;
}
return len;
}
bool Push(LinkStack &L,int e)
{
LNode *s=(LNode *)malloc(sizeof(LNode));
if(s==NULL)
return false;
s->data=e;
s->next=L->next;
L->next=s;
return true;
}
int Pop(LinkStack &L)
{
int e;
if(Empty(L))
{
printf("栈空,出栈操作失败\n");
return false;
}
LNode *q=L->next;
e=q->data;
L->next=q->next;
free(q);
return e;
}
int GetTop(LinkStack &L)
{
if(Empty(L))
{
printf("栈空,读栈顶元素失败\n");
return false;
}
return L->next->data;
}
void Show(LinkStack L)
{
LNode *p=L->next;
while(p!=NULL)
{
printf("%d ",p->data);
p=p->next;
}
printf("\nLength: %d\n",Length(L));
}
int main()
{
LinkStack L;
InitList(L);
Push(L,1);
Push(L,2);
Push(L,3);
Push(L,4);
Push(L,5);
Show(L);
int e=Pop(L);
printf("已删除栈顶元素,删除元素值为 %d,删除后链表为:\n",e);
Show(L);
int t=GetTop(L);
printf("栈顶元素为: %d\n",t);
LNode *p1=GetElem(L,2);
printf("栈L中位置为2的元素为 %d\n",p1->data);
return 0;
}
代码运行结果 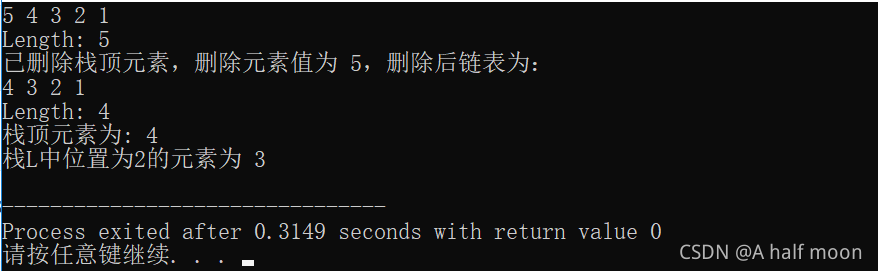
|