9.5 Collections类与比较器
9.5.1 Collections类
Java的集合框架中还有一个重要的辅助工具类java.util.Collections, 它包含了各种有关集合操作的静态多态方法。此类不能实例化,用于对集合中的元素进行排序、查询以及线程安全等各种操作算法,为集合提供服务。Collections 类的方法有几十个,下表仅列出查找 替换、排序的常用方法,其余详细用法可参考Java API。 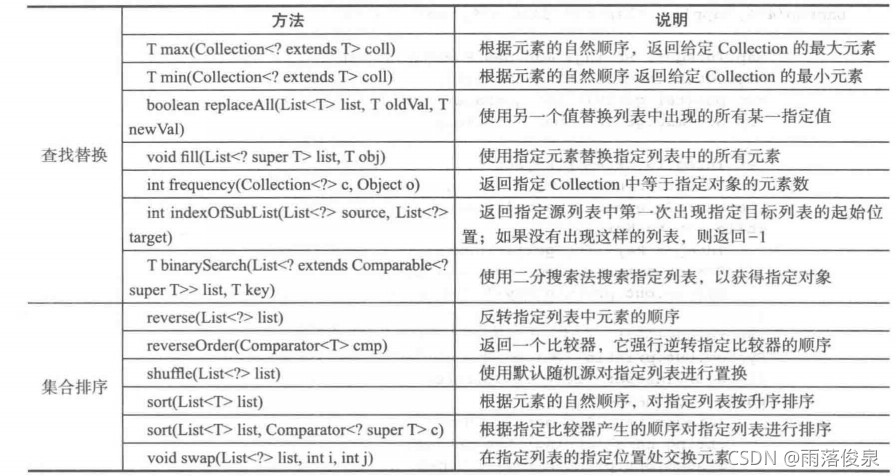 另外,Collections 类还提供了同步集合的功能,Collections 的synchronizedXXX系列方法(XXX表示集合类型)会返回同步化集合类,如synchronizedMap、synchronizedList、synchronizedSet 等,它们都是通过互斥机制来实现对集合操作的同步化。
示例 Collections 常用方法演示。
代码如下
public class CollectionsTest {
public static void main(String[] args) {
List<Double> list = new ArrayList<Double>();
double array[]={123,243,141.1,23,45};
for (int i = 0; i < array.length; i++) {
list.add(new Double(array[i]));
}
Collections.sort(list);
System.out.println("sorted number: ");
for (int i = 0; i <array.length ; i++) {
System.out.println(list.get(i));
}
Collections.reverse(list);
System.out.println("reversed number: ");
for (int i = 0; i <array.length ; i++) {
System.out.println(list.get(i));
}
System.out.println("min number is: "+Collections.min(list));
}
}
运行结果如下
sorted number:
23.0
45.0
123.0
141.1
243.0
reversed number:
243.0
141.1
123.0
45.0
23.0
min number is: 23.0
9.5.2 比较器
Java的集合框架里有两类比较器: Comparable 和Comparator,分别对应java.util.Comparable 接口和java.util.Comparator 接口,用于对集合对象进行排序。
Comparable属于排序接口,即实现它的具体类都支持排序功能。假如这些具体类的对象存放于某种集合或数组中,则该集合或数组可以调用Collections.sort()或Arrays.sort()来进行排序。另外,TreeSet 和TreeMap本身具有排序功能,不需要比较器。
Comparable接口只有一个方法: public int compareTo(T o) 。如果要比较对象a和b的大小,则a.compareTo(b)。返回值小于0,表示a小于b;返回值等于0,表示a等于b;返回值大于0,表示a大于b。
Comparator是比较器接口,如果某个类的对象需要排序,而该类本身没有实现Comparable接口,则可以通过此类实现Comparator接口来为其创建一个比较器比较对象大小,然后通过比较结果排序。这和Comparable的具体类不同,Comparable 的具体类属于自身具备排序功能的排序类。
Comparator接口有两个方法: int compare(T o1, T o2) ; boolean equals(Object obj) 。 若一个类要实现Comparator接口,它一定要实现compare(To1,To2)方法,但可以不实现equals(Object obj)方法,因为所有类都已经默认继承了java.lang.Object中的equals(Object obj) 。int compare(T o1, T o2) 可以比较o1和o2的大小。返回值小于0,表示o1小于o2小;返回值为0,表示o1 等于o2;返回值大于0,表示o1大于o2。
class ProductMe implements Comparable<ProductMe>{
int id;
String name;
public ProductMe(int id, String name) {
this.id = id;
this.name = name;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
@Override
public String toString() {
return name+"-"+id;
}
@Override
public int compareTo(ProductMe productMe) {
return name.compareTo(productMe.name);
}
}
class AscComparator implements Comparator<ProductMe>{
@Override
public int compare(ProductMe productMe, ProductMe t1) {
return productMe.getId()-t1.getId();
}
}
class DescComparator implements Comparator<ProductMe>{
@Override
public int compare(ProductMe productMe, ProductMe t1) {
return t1.getId()-productMe.getId();
}
}
public class CompareTest {
public static void main(String[] args) {
ArrayList<ProductMe>list = new ArrayList<>();
list.add(new ProductMe(12,"a"));
list.add(new ProductMe(10,"c"));
list.add(new ProductMe(22,"d"));
list.add(new ProductMe(11,"b"));
System.out.printf("original sort,list: %s\n",list);
Collections.sort(list);
System.out.printf("product name sorted list:%s\n",list);
Collections.sort(list,new AscComparator());
System.out.printf("asc id sorted,list:%s\n",list);
Collections.sort(list,new DescComparator());
System.out.printf("desc id sorted,list:%s\n",list);
}
}
运行结果如下
original sort,list: [a-12, c-10, d-22, b-11]
product name sorted list:[a-12, b-11, c-10, d-22]
asc id sorted,list:[c-10, b-11, a-12, d-22]
desc id sorted,list:[d-22, a-12, b-11, c-10]
|