php-策略模式实现
概述
策略模式(Strategy Pattern):定义一系列算法,将每一个算法封装起来,并让它们可以相互替换。策略模式让算法独立于使用它的客户而变化,也称为政策模式(Policy)。
模式结构
- MotorcycleProduce-摩托组装接口:建立摩托组装标准工艺
- MotocycleProduct -摩托车产品本身
- MotorcycleScooterProduce-踏板摩托车组装者:负责踏板摩托车的组装工作
- MotorcycleScooterProduceNew-踏板摩托车组装者:负责踏板摩托车的组装工作
- MotorScooter-策略类,用于指定踏板摩托车生产工艺
图例
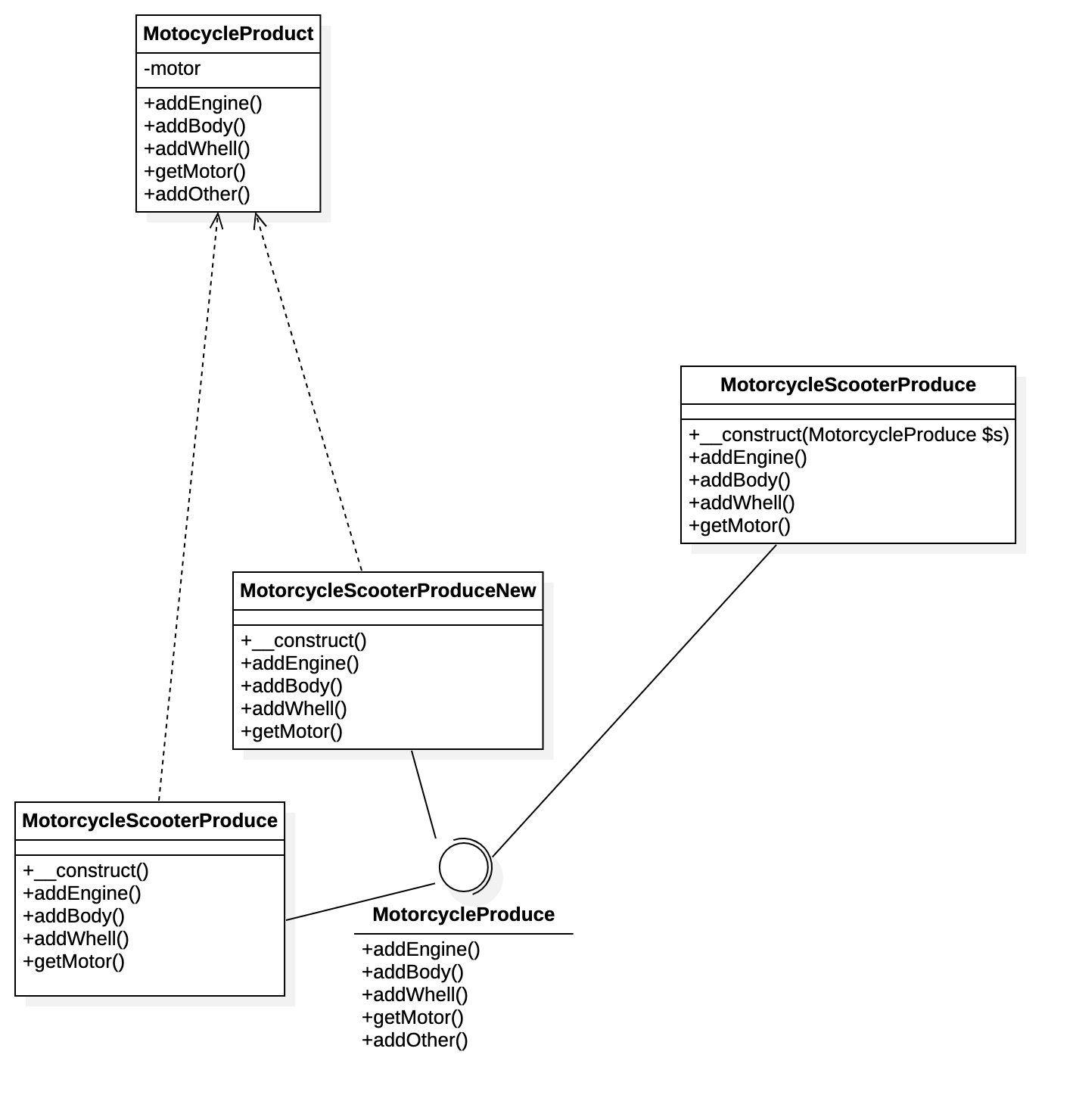
代码范例
<?php
interface MotorcycleProduce
{
public function addEngine();
public function addBody();
public function addWhell();
public function getMotor();
}
class MotocycleProduct{
private $motor = [
"engine"=>"",
"body"=>"",
"whell"=>"",
];
public function addEngine($engine){
$this->motor["engine"] = $engine;
}
public function addBody($body){
$this->motor["body"] = $body;
}
public function addWhell($whell){
$this->motor["whell"] = $whell;
}
public function getMotor(){
return $this->motor;
}
}
class MotorcycleScooterProduce implements MotorcycleProduce
{
public function __construct()
{
$this->motor = new MotocycleProduct();
}
public function addEngine()
{
$this->motor->addEngine("踏板摩托-发动机已装好");
}
public function addBody(){
$this->motor->addBody("踏板摩托-车身已装好");
}
public function addWhell(){
$this->motor->addWhell("踏板摩托-车轮已装好");
}
public function getMotor(){
return $this->motor->getMotor();
}
}
class MotorcycleScooterProduceNew implements MotorcycleProduce
{
public function __construct()
{
$this->motor = new MotocycleProduct();
}
public function addEngine()
{
$this->motor->addEngine("新工艺-踏板摩托-发动机已装好");
}
public function addBody(){
$this->motor->addBody("新工艺-踏板摩托-车身已装好");
}
public function addWhell(){
$this->motor->addWhell("新工艺-踏板摩托-车轮已装好");
}
public function getMotor(){
return $this->motor->getMotor();
}
}
class MotorScooter{
private $strategy;
function __construct(MotorcycleProduce $s){
$this->strategy = $s;
}
function getMotor(){
$this->strategy->addEngine();
$this->strategy->addBody();
$this->strategy->addWhell();
return $this->strategy->getMotor();
}
}
$motorcycleScooterProduce = new MotorcycleScooterProduce();
$motorScooter = new MotorScooter($motorcycleScooterProduce);
$motor = $motorScooter->getMotor();
var_dump($motor);
echo "<br>";
$motorcycleScooterProduceNew = new MotorcycleScooterProduceNew();
$motorScooter = new MotorScooter($motorcycleScooterProduceNew);
$motor = $motorScooter->getMotor();
var_dump($motor);
模式分析
优缺点
策略模式的优点
- 策略模式提供了对“开闭原则”的完美支持,用户可以在不修改原有系统的基础上选择算法或行为,也可以灵活地增加新的算法或行为。
- 避免使用多重条件IF语句。提高系统的灵活性
- 替换继承关系的办法
- 灵活管理多实现方式
策略模式的缺点
- 客户端必须知道所有的策略类,并自行决定使用哪一个策略类。
- 策略模式将造成产生很多策略类,可以通过使用享元模式在一定程度上减少对象的数量。
适用环境
在以下情况下可以使用策略模式:
- 如果在一个系统里面有许多类,它们之间的区别仅在于它们的行为,那么使用策略模式可以动态地让一个对象在许多行为中选择一种行为。
- 如果一个对象有很多的行为,如果不用恰当的模式,这些行为就只好使用多重的条件选择语句来实现。
- 不希望客户端知道复杂的、与算法相关的数据结构,在具体策略类中封装算法和相关的数据结构,提高算法的保密性与安全性。
- 需要动态的对一个模块选择实现方式
获取更多学习资源请关注微信公众号:yuantanphp
|