字符串轮转。给定两个字符串s1和s2,请编写代码检查s2是否为s1旋转而成(比如,waterbottle是erbottlewat旋转后的字符串)。
开始打算遍历两个字符串, 越做越麻烦,后来看题解知道应该这么做:“ 首先判断两个字符串长度是否相等,再判断把两个s1连起来能不能包含s2”
package mianshi;
import java.util.Arrays;
public class IsFlipedString {
public boolean isFlipedString(String s1, String s2) {
if(s1 == null || s2 == null){
return false;
}
if(s1.length() != s2.length()){
return false;
}
String s = s1 + s1;
int res = kmpIndex(s, s2);
System.out.println(res);
return res != -1;
}
private int kmpIndex(String s, String t){
int[] next = getNext(t);
int i = 0, j = 0;
while (i < s.length() && j < t.length()){
if(j == -1 || s.charAt(i)==t.charAt(j)){
i++;
j++;
}
else {
j = next[j];
}
}
if(j >= t.length()){
return (i-t.length());
}
else {
return -1;
}
}
private int[] getNext(String s){
int[] next = new int[s.length()];
int j, k;
j = 0; k = -1;
next[0] = -1;
while (j < s.length()-1){
if(k == -1 || s.charAt(j) == s.charAt(k)){
j++; k++;
if (s.charAt(j)!=s.charAt(k)) {
next[j] = k;
}
else {
next[j] = next[k];
}
}else{
k = next[k];
}
}
return next;
}
public static void main(String[] args) {
String s1 = "waterbottle";
String s2 = "erbottlewat";
boolean res = new IsFlipedString().isFlipedString(s1, s2);
System.out.println("Res is "+res);
String s3 = "aaaaab";
int[] next = new IsFlipedString().getNext(s3);
System.out.println("Res is "+res);
}
}
使用kmp来做, kmp:KMP算法的作用是在一个已知字符串中查找子串的位置,也叫做串的模式匹配。
求next的方法: 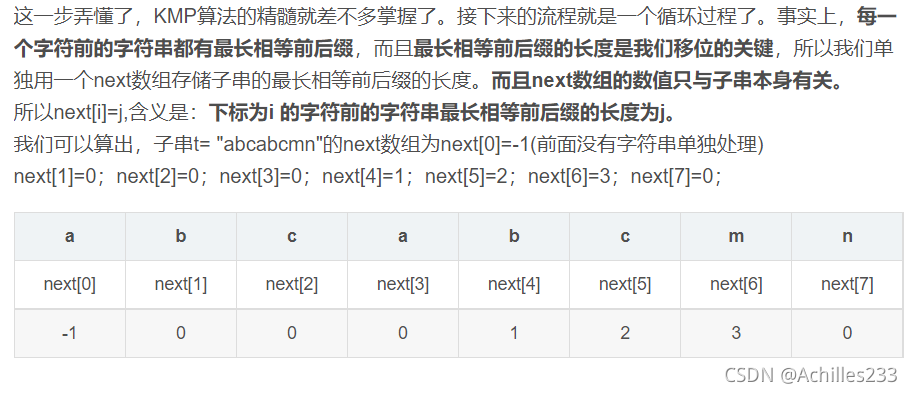 优化next的原理:
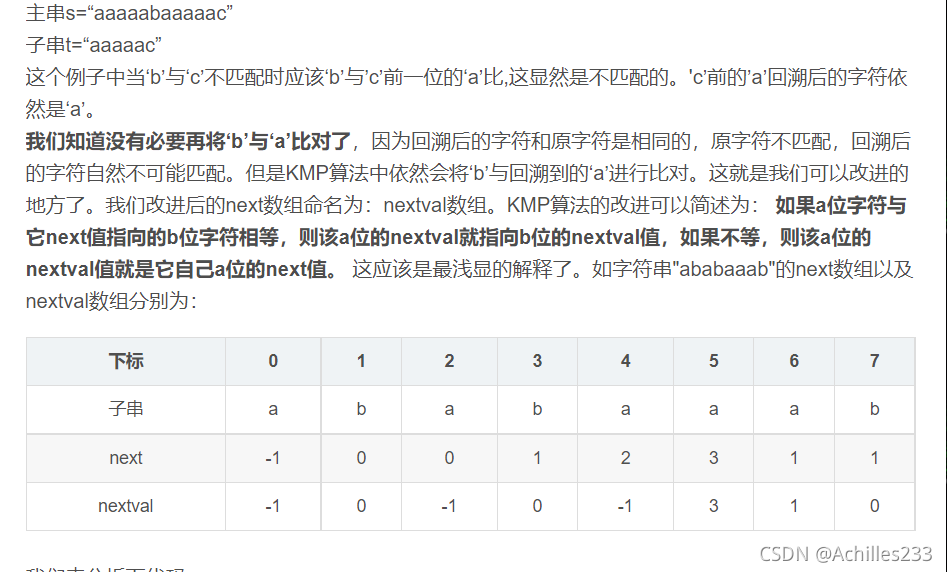
kmp算法的学习来源是: https://blog.csdn.net/weixin_46007276/article/details/104372119?ops_request_misc=%257B%2522request%255Fid%2522%253A%2522163090574116780261967375%2522%252C%2522scm%2522%253A%252220140713.130102334…%2522%257D&request_id=163090574116780261967375&biz_id=0&utm_medium=distribute.pc_search_result.none-task-blog-2alltop_positive~default-1-104372119.pc_search_ecpm_flag&utm_term=kmp&spm=1018.2226.3001.4187
|