题目的链接在这里:https://leetcode-cn.com/problems/binary-tree-postorder-traversal/
题目大意
给定一个二叉树,返回它的 后序 遍历。
一、示意图
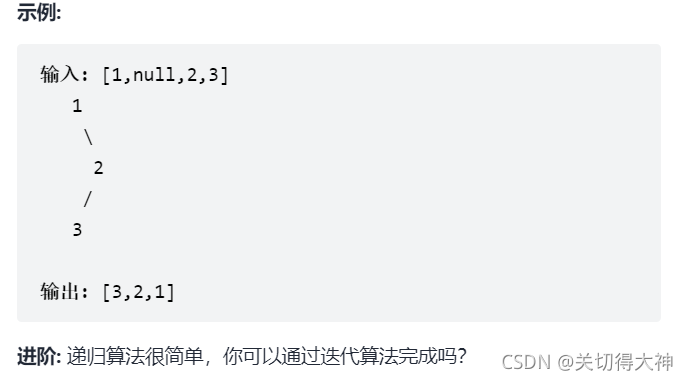
二、解题思路
递归和非递归
递归
代码如下:
class Solution {
List<Integer> list=new ArrayList<Integer>();
public List<Integer> postorderTraversal(TreeNode root) {
if(root==null)
return list;
postorder(root,list);
return list;
}
private void postorder(TreeNode root, List<Integer> list) {
if(root==null)
return;
else {
postorder(root.left,list);
postorder(root.right,list);
list.add(root.val);
}
}
}
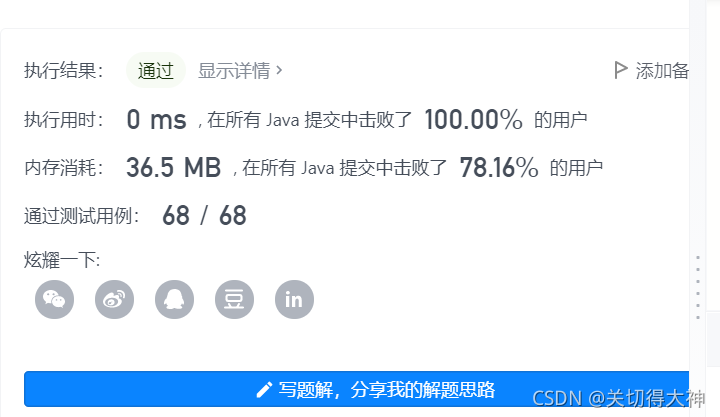
非递归
代码如下:
class Solution {
List<Integer> list=new ArrayList<Integer>();
public List<Integer> postorderTraversal(TreeNode root) {
if(root==null)
return list;
TreeNode cur,pre=null;
Stack<TreeNode> stack=new Stack<TreeNode>();
stack.push(root);
while (!stack.isEmpty()){
cur=stack.peek();
if((cur.left==null&&cur.right==null)||(pre!=null&&(pre==cur.left||pre==cur.right))){
list.add(cur.val);
stack.pop();
pre=cur;
}
else{
if(cur.right!=null){
stack.push(cur.right);
}
if(cur.left!=null){
stack.push(cur.left);
}
}
}
return list;
}
}
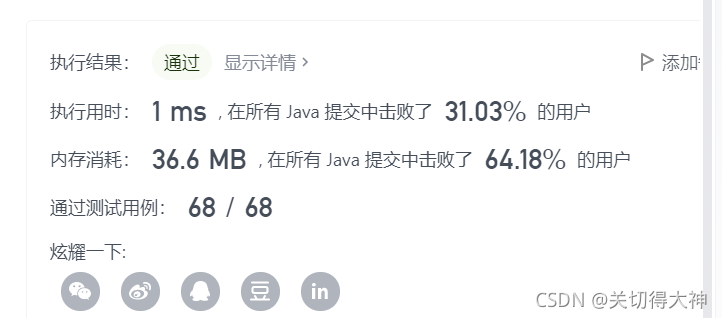
|