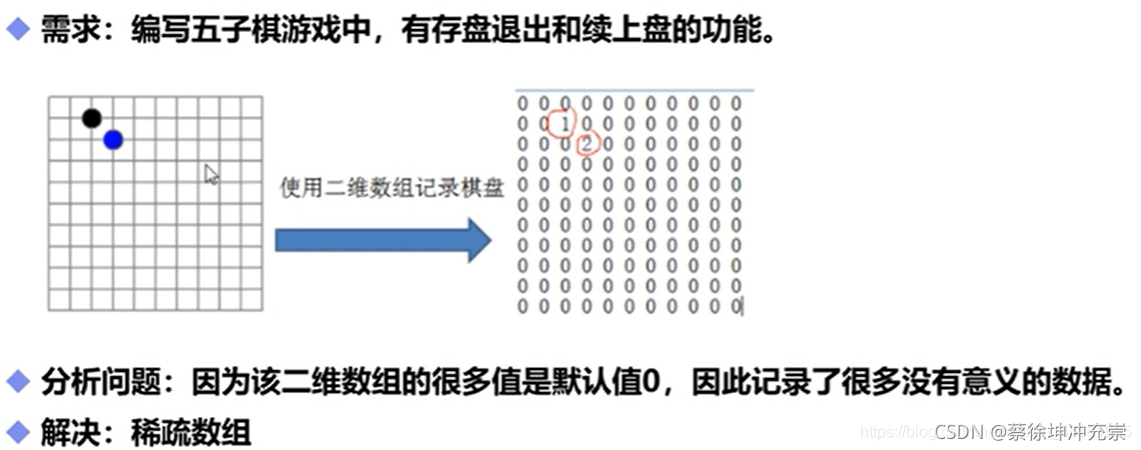
- 当一个数组中大部分元素为0,或者为同一值的数组时,可以使用稀疏数组来保存该数组。
- 稀疏数组的处理方式是:记录数组一共有几行几列,有多少个不同值;把具有不同值的元素和行列及值记录在一个小规模的数组中,从而缩小程序的规模
如下图:左边是原始数组,右边是稀疏数组 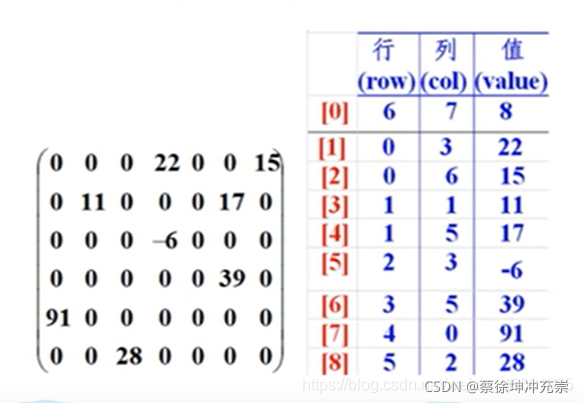
二维数组转稀疏数组思路
1)遍历原始的二维数组,得到有效数总数为sum 2)根据得到的sum就可以创建数组 parseArray int[sum+1][3] 3)将二维数组数据存进稀疏数组
稀疏数组转二维数组思路
1)先读取稀疏数组的第一行,根据第一行数据创建原始数组 chessArray=int[6][7] 2)读取稀疏数组的数据写进二维数组
代码实现:
import java.io.*;
public class SparseArray {
public static void main(String[] args) throws IOException {
int chessArr1[][] = new int[11][11];
chessArr1[1][2] = 1;
chessArr1[2][3] = 2;
chessArr1[1][1] = 3;
System.out.println("原始的二维数组为:");
for (int[] row : chessArr1) {
for (int data : row) {
System.out.print(data+"\t");
}
System.out.println();
}
System.out.println("=====================");
int sum = 0;
for (int i = 0; i < 11; i++) {
for (int j = 0; j < 11; j++) {
if (chessArr1[i][j] != 0){
sum++;
}
}
}
System.out.println("sum="+sum);
int sparseArray[][] = new int[sum+1][3];
sparseArray[0][0] = 11;
sparseArray[0][1] = 11;
sparseArray[0][2] = sum;
int count = 0;
for (int i=0;i<11;i++){
for (int j=0;j<11;j++){
if (chessArr1[i][j] != 0){
count++;
sparseArray[count][0] = i;
sparseArray[count][1] = j;
sparseArray[count][2] = chessArr1[i][j];
}
}
}
System.out.println("======================");
System.out.println("得到的稀疏数组:");
for (int[] row : sparseArray){
for (int data : row){
System.out.print(data+"\t");
}
System.out.println();
}
System.out.println("将数组写入文件==========");
writeFile(sparseArray);
System.out.println("从文件读取数据==========");
int arr[][] = readFile("/Users/liuben/Desktop/data/file/test.txt");
for (int[] row : arr){
for (int data : row){
System.out.print(data+"\t");
}
System.out.println();
}
System.out.println("=============================");
System.out.println("------------------------------------------");
System.out.println("还原数组:");
int chessArr2[][] = new int[sparseArray[0][0]][sparseArray[0][1]];
for (int i=1;i<sparseArray.length;i++){
chessArr2[sparseArray[i][0]][sparseArray[i][1]] = sparseArray[i][2];
}
for (int[] row : chessArr2){
for (int data : row){
System.out.print(data+"\t");
}
System.out.println();
}
}
public static void writeFile(int arr[][]) throws IOException {
File file = new File("/Users/liuben/Desktop/data/file/test.txt");
if (!file.exists()){
file.createNewFile();
}
OutputStream ops = new FileOutputStream("/Users/liuben/Desktop/data/file/test.txt");
OutputStreamWriter writer = new OutputStreamWriter(ops, "UTF-8");
for (int i=0;i<arr.length;i++){
for (int j=0;j<arr[i].length;j++){
writer.write(arr[i][j]+"\t");
}
writer.write("\n");
}
writer.flush();
writer.close();
}
public static int[][] readFile(String path) throws IOException {
File file = new File(path);
if (!file.exists()){
return new int[1][1];
}
FileInputStream fis = new FileInputStream(path);
InputStreamReader reader = new InputStreamReader(fis, "UTF-8");
BufferedReader bufferedReader = new BufferedReader(reader);
String[] strings = bufferedReader.readLine().split("\\s+");
int arr[][] = new int[Integer.parseInt(strings[0])][Integer.parseInt(strings[1])];
String lineText = null;
while ((lineText =bufferedReader.readLine()) != null){
String[] split = lineText.split("\\s+");
arr[Integer.parseInt(split[0])][Integer.parseInt(split[1])] = Integer.parseInt(split[2]);
}
reader.close();
return arr;
}
}
稀疏数组存入磁盘以及从磁盘读取文件(省略)
|