单链表反转
题目: 给你单链表的头节点 head ,请你反转链表,并返回反转后的链表。 (这里我好迷,LeetCode上意思是head头结点也默认要存储一个数据,否则会错)
题目自定义好的节点类:
class ListNode {
int val;
ListNode next;
ListNode() {}
ListNode(int val) { this.val = val; }
ListNode(int val, ListNode next) { this.val = val; this.next = next; }
}
我的答案:
public class Solution2 {
public ListNode reverseList(ListNode head) {
Stack<Integer> s = new Stack<>();
ListNode n=head;
while (n!=null){
s.push(n.val);
n=n.next;
}
n=head;
while(s.size()>0){
n.val=s.pop();
n=n.next;
}
return head;
}
}
我个人是利用栈先进先出获取所有数据,然后再重新给节点一一赋值,貌似速度有点慢。这里复杂度时间复杂度是O(n),空间复杂度是O(1)。 后面再给出官方的写法对比下吧 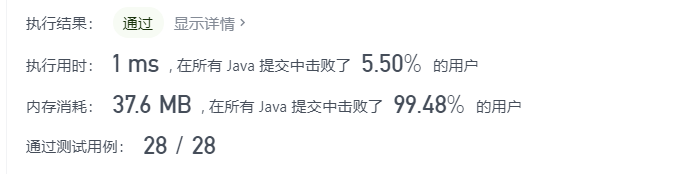
官方解答:
1、迭代:
class Solution {
public ListNode reverseList(ListNode head) {
ListNode prev = null;
ListNode curr = head;
while (curr != null) {
ListNode next = curr.next;
curr.next = prev;
prev = curr;
curr = next;
}
return prev;
}
}
2、递归:
class Solution {
public ListNode reverseList(ListNode head) {
if (head == null || head.next == null) {
return head;
}
ListNode newHead = reverseList(head.next);
head.next.next = head;
head.next = null;
return newHead;
}
}
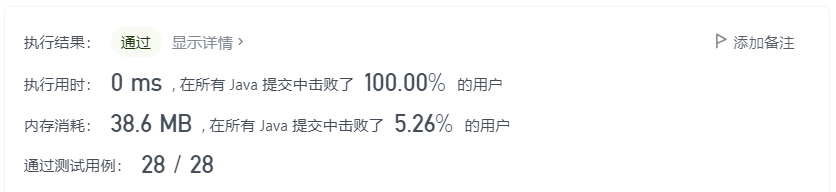
|