WC140 三个数的最大乘积
给定一个长度为 的无序数组 ,包含正数、负数和 0 ,请从中找出 3 个数,使得乘积最大,返回这个乘积。
public long solve (int[] A) {
// write code here
Arrays.sort(A);
return Math.max((long)A[0]*A[1]*A[A.length-1],(long)A[A.length-1]*A[A.length-2]*A[A.length-3]);//加上类型转换
}
CM8 翻转子串
给定2个字符串s1和s2,请判断s2是否为s1旋转而成,返回bool值。字符串中字符为英文字母和空格,区分大小写,字符串长度小于等于1000。
测试样例: “Hello world”,"worldhello " 返回:false “waterbottle”,“erbottlewat” 返回:true
public boolean checkReverseEqual(String s1, String s2) {
// write code here
String s=s1+s1;
if(s.indexOf(s2)>-1){
return true;
}
return false;
}
WC43 删除有序链表中重复的元素-I
删除给出链表中的重复元素(链表中元素从小到大有序),使链表中的所有元素都只出现一次 例如: 给出的链表为1\to1\to21→1→2,返回1 \to 21→2. 给出的链表为1\to1\to 2 \to 3 \to 31→1→2→3→3,返回1\to 2 \to 31→2→3.
public ListNode deleteDuplicates (ListNode head) {
ListNode next1=head;
ListNode cur=head;
if(head==null)
return head;
while(next1!=null){
if(head.val!=next1.val){
head.next=next1;
head=head.next;
}
next1=next1.next;
}
head.next=null;
return cur;
WC35 不同的二叉搜索树
给定一个值n,能构建出多少不同的值包含1…n的二叉搜索树(BST)? 例如 给定 n = 3, 有五种不同的二叉搜索树(BST) 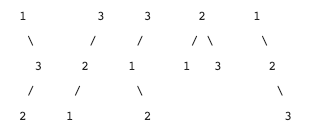 设值为n的情况下,可以组成f(n)个二叉搜索树,根据规律可知: f(0) = 1 f(1) = 1 f(n) += f(k-1)*f(n-k), 其中k=1,2,…n
public int numTrees (int n) {
// write code here
if(n==1 || n==2) {
return n;
}
int [] dp=new int[n+1];
dp[0]=1;
dp[1]=1;
for(int i=2;i<=n;i++) {
for(int j=1;j<=i;j++){
dp[i]+=dp[j-1]*dp[i-j];
}
}
return dp[n];
}
|