基本概念
循环链表的定义:将单链表的最后一个数据元素的next指针指向第一个元素  循环链表拥有单链表的所有操作 创建链表 销毁链表 获取链表长度 清空链表 获取第pos个元素操作 插入元素到位置pos 删除位置pos处的元素
新增功能
游标 即在循环链表中可以定义一个“当前”指针,这个指针通常称为游标,可以通过这个游标遍历链表中所有的元素 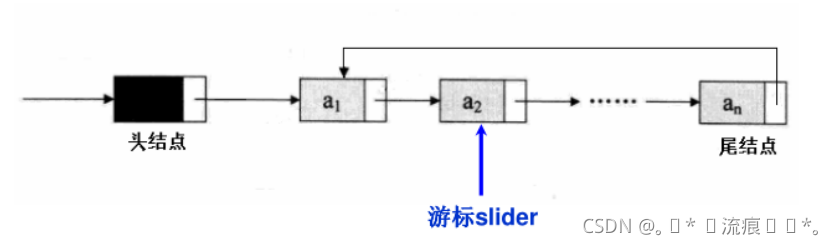
游标重置
CircleListNode* CircleList_Reset(CircleList* list);
获取当前游标指向的数据元素
CircleListNode* CircleList_Current(CircleList* list);
游标移动指向下一个数据元素
CircleListNode* CircleList_Next(CircleList* list);
指定删除链表的某个数据元素
CircleListNode* CircleLIst_DeleteNode(CircleList* list,CircleListNode* node);
特点
循环链表可以完全取代单链表的使用 循环链表的Next和Current操作可以高效的遍历链表中所有元素
提高了代码复杂度
循环链表插入结点
调用自定义函数
void insert_node (list_t *insert_node,list_t*prev_node,list_t* next_node)
{
insert_node->next = next_node;
insert_node->prev = prev_node;
next_node->prev = insert_node;
prev_node->next = insert_noed;
}
头插法
void insert_head(list_t*head,list_t* insert)
{
insert_node(insert,head,head->next);
}
尾插
void insert_tail(list_t* head,list_t*insert)
{
insert_node(insert,head->prev,head);
}
循环链表删除结点
void Delete(list **pNode,int place)
{
list *temp,*target;
int i;
temp=*pNode;
if(temp==NULL)
{
return ;
}
if(place==1)
{
for(target=*pNode;target->next!=*pNode;target=target->next);
temp=*pNode;
*pNode=(*pNoe)->next;
target->next=*pNode;
delete temp;
}
else
{
temp=target->next;
target->next=temp->next;
delete temp;
}
}
|