#include <iostream>
using namespace std;
char pause;
typedef int T;
template <class T>
class SqList
{
private:
T *elem;
int length;
int listsize;
public:
SqList(int m);
~SqList();
void CreateList(int n);
void Insert(int i, T e);
T Delete(int i);
T GetElem(int i);
int Locate(T e);
void Clear();
int Empty();
int Full();
int Length();
void ListDisp();
};
template <class T>
SqList<T>::SqList(int m)
{
elem = new T[m];
length = 0;
listsize = m;
}
template <class T>
SqList<T>::~SqList()
{
delete[] elem;
length = 0;
listsize = 0;
}
template <class T>
void SqList<T>::CreateList(int n)
{
if (n > listsize)
throw "参数非法";
cout << "请依次输入" << n << "个元素:" << endl;
for (int i = 1; i <= n; i++)
cin >> elem[i - 1];
length = n;
}
template <class T>
void SqList<T>::Insert(int i, T e)
{
if (length > listsize)
throw "上溢";
if (i < 1 || i > length + 1)
throw "插入位置异常";
for (int j = length; j >= i; j--)
elem[j] = elem[j - 1];
elem[i - 1] = e;
length++;
}
template <class T>
T SqList<T>::Delete(int i)
{
T x;
if (length == 0)
throw "下溢";
if (i < 1 || i > length)
throw "删除位置异常";
x = elem[i - 1];
for (int j = i; j < length; j++)
elem[j - 1] = elem[j];
length--;
return x;
}
template <class T>
int SqList<T>::Locate(T e)
{
for (int i = 0; i < length - 1; i++)
if (elem[i] == e)
return i + 1;
return 0;
}
template <class T>
void SqList<T>::Clear()
{
length = 0;
}
template <class T>
T SqList<T>::GetElem(int i)
{
T e;
if (i < 1 || i > length)
throw "位置不合法";
e = elem[i - 1];
return 0;
}
template <class T>
int SqList<T>::Empty()
{
if (length == 0)
return 1;
return 0;
}
template <class T>
int SqList<T>::Full()
{
if (length == listsize)
return 1;
return 0;
}
template <class T>
int SqList<T>::Length()
{
return length;
}
template <class T>
void SqList<T>::ListDisp()
{
for (int i = 0; i < length; i++)
{
cout << i + 1 << "\t";
cout << elem[i] << endl;
}
}
int main()
{
int i;
T e;
SqList<int> L(20);
system("cls");
int choice;
do
{
cout << "1-创建顺序\n";
cout << "2-在链表第i个位置插入元素\n";
cout << "3-删除顺序表中第i个位置的元素\n";
cout << "4-返回第i个元素的值\n";
cout << "5-元素定位\n";
cout << "6-清空表\n";
cout << "7-测表空\n";
cout << "8-测表满\n";
cout << "9-测表长\n";
cout << "10-显示表\n";
cout << "11-退出\n";
cout << "Enter choice:";
cin >> choice;
switch (choice)
{
case 1:
cout << "请输入要创建的顺序表中元素的个数:";
cin >> i;
cout << endl;
L.CreateList(i);
break;
case 2:
cout << "请输入插入位置:";
cin >> i;
cout << endl;
cout << "请输入插入元素的值:";
cin >> e;
cout << endl;
L.Insert(i, e);
break;
case 3:
cout << "请输入删除位置:";
cin >> i;
cout << endl;
L.Delete(i);
cin.get(pause);
system("pause");
break;
case 4:
cout << "请输入要查询的元素位置";
cin >> i;
e = L.GetElem(i);
cout << "第" << i << "个元素值为:" << e << endl;
cin.get(pause);
system("pause");
case 5:
cout << "请输入要查询的元素值:";
cin >> e;
i = L.Locate(e);
cout << "查询元素" << e << "位于在表中的位置为:" << i << endl;
cin.get(pause);
system("pause");
break;
case 6:
L.Clear();
cout << "表已清空" << endl;
cin.get(pause);
system("pause");
break;
case 7:
i = L.Empty();
if (i)
cout << "表空" << endl;
else
cout << "表不空" << endl;
cin.get(pause);
system("pause");
break;
case 8:
i = L.Full();
if (i)
cout << "表满" << endl;
else
cout << "表未满" << endl;
cin.get(pause);
system("pause");
break;
case 9:
i = L.Length();
cout << "表长为:" << i << endl;
cin.get(pause);
system("pause");
break;
case 10:
L.ListDisp();
cout << endl;
cin.get(pause);
system("pause");
break;
case 11:
break;
default:
cout << "Invalid choice\n";
break;
}
} while (choice != 11);
return 0;
}
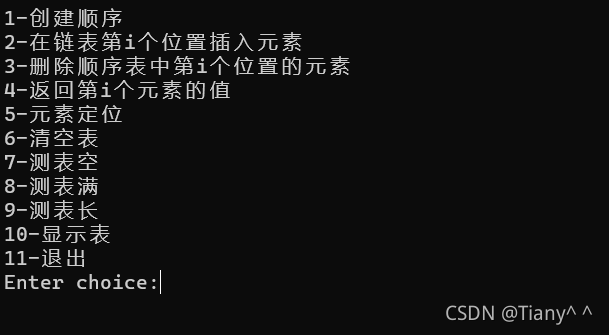
|