单链表类的定义
class Node(object):
def __init__(self, data):
self.data = data
self.next = None
头插法添加元素
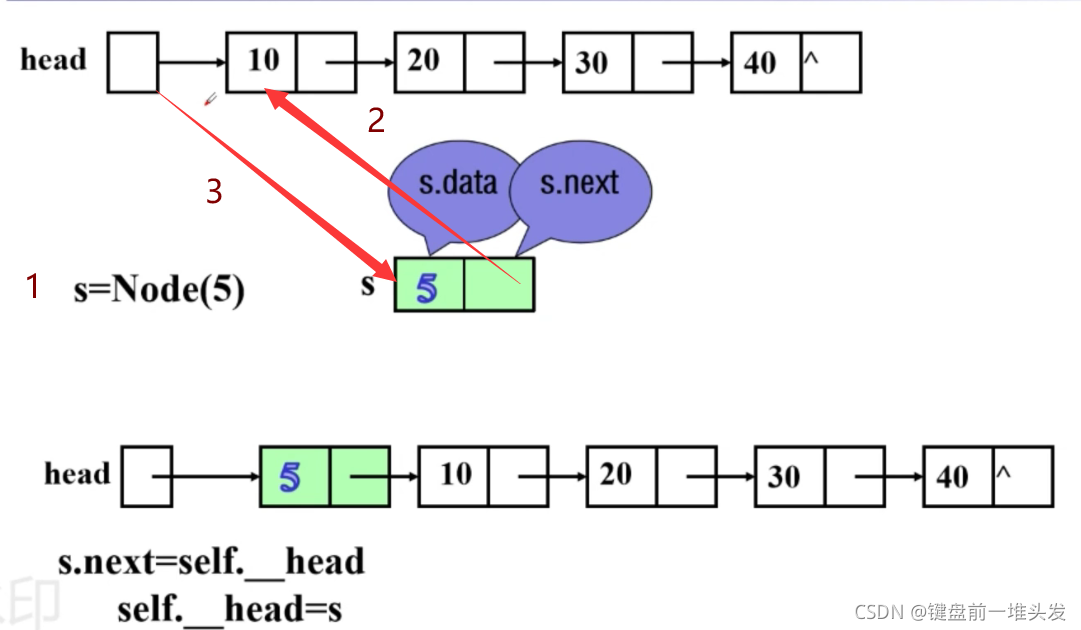
遍历单链表

class Node(object):
def __init__(self, data):
self.data = data
self.next = None
class List(object):
'''创建一个单链表类'''
def __init__(self):
'''初始化单链表'''
self.__head = None
def is_empty(self):
if self.__head:
return 1
else:
return 0
def add(self, item):
'''头插法'''
s = Node(item)
s.next = self.__head
self.__head = s
def travel(self):
'''遍历单恋表'''
p = self.__head
while p != None:
print(p.data, end='')
p = p.next
尾插法

- 先把指针移动到最后一个节点
- 创建一个节点
- 把新结点的地址放在最后一个节点的指针域里
指定位置插入元素insert(self, pos, x)
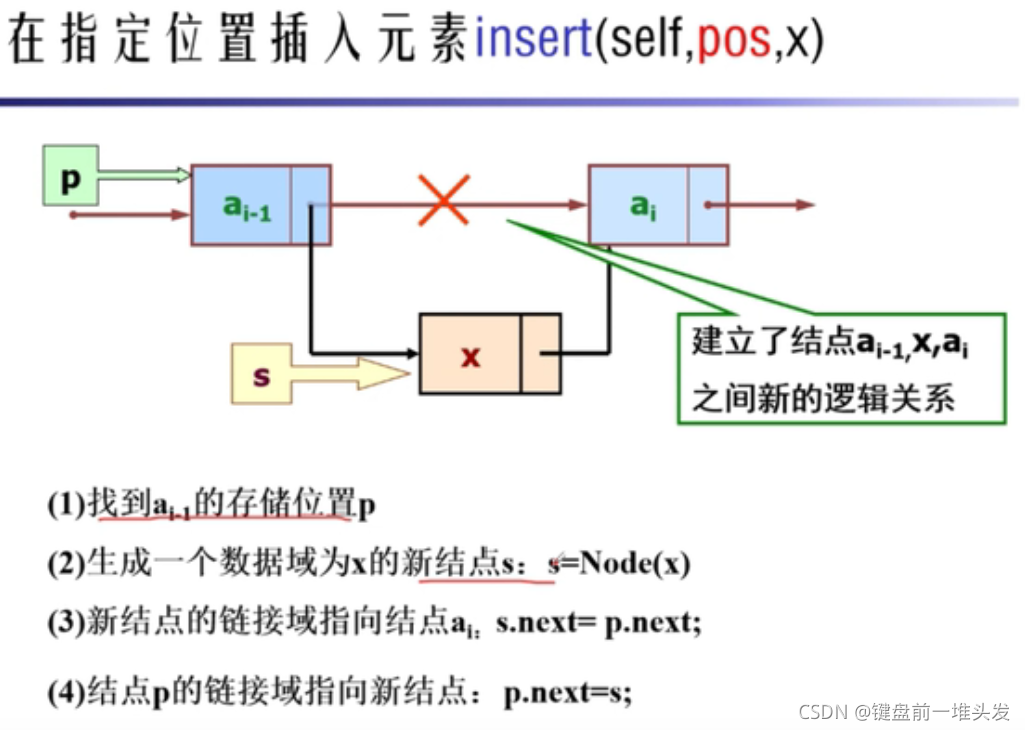
按值删除元素
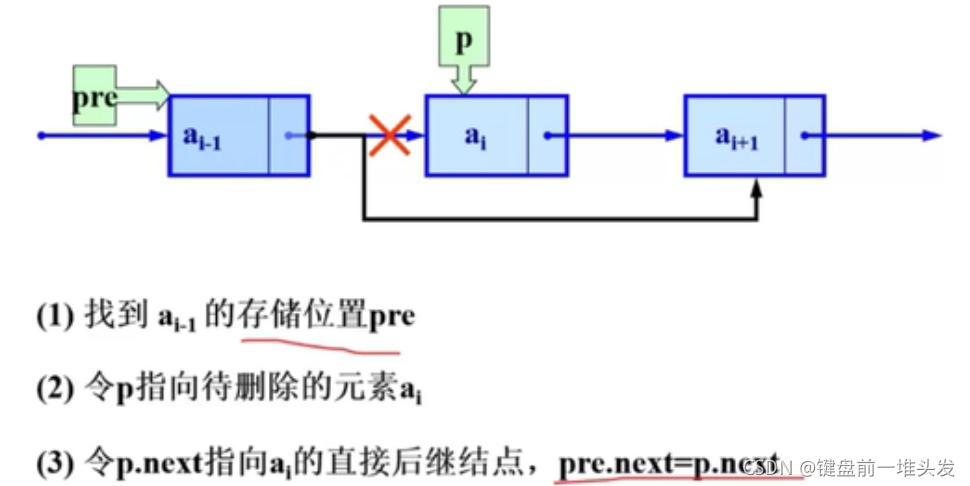
单链表增删查改完整代码python
class Node(object):
def __init__(self, data):
self.data = data
self.next = None
class List(object):
'''创建一个单链表类'''
def __init__(self):
'''初始化单链表'''
self.__head = None
def is_empty(self):
if self.__head:
return 1
else:
return 0
def add(self, item):
'''头插法'''
s = Node(item)
s.next = self.__head
self.__head = s
def add(self, item):
'''头插法'''
s = Node(item)
s.next = self.__head
self.__head = s
def add_end(self, x):
'''尾插法'''
s = Node(x)
p = self.__head
if self.__head == None:
self.__head = s
else:
while p.next != None:
p = p.next
p.next = s
def insert(self, pos, x):
'''指定位置插入元素'''
p = self.__head
count = 0
if pos >= self.length():
return -1
while count < pos:
p = p.next
count += 1
s = Node(x)
s.next = p.next
p.next = s
return 1
def remove(self, item):
p = self.__head
if p.data == item:
self.__head = p.next
else:
while p!=None and p.data!=item:
pre = p
p = p.next
pre.next = p.next
def travel(self):
'''遍历单恋表'''
p = self.__head
while p != None:
print(p.data, end=' ')
p = p.next
print('\n')
def length(self):
p = self.__head
count = 0
while p != None:
count +=1
p = p.next
return count
def search_pos(self, pos):
'''按序号查找,pos是待查找的位置'''
p = self.__head
count = 1
if pos < 1:
return None
else:
while p != None and count != pos:
p = p.next
count += 1
return p
def search_value(self, value):
'''search a item by value'''
p = self.__head
while p!=None and p.data!=value:
p = p.next
if p.data == value:
return p
elif p == None:
return None
|