一、简单二叉树
为什么需要树这种数据结构
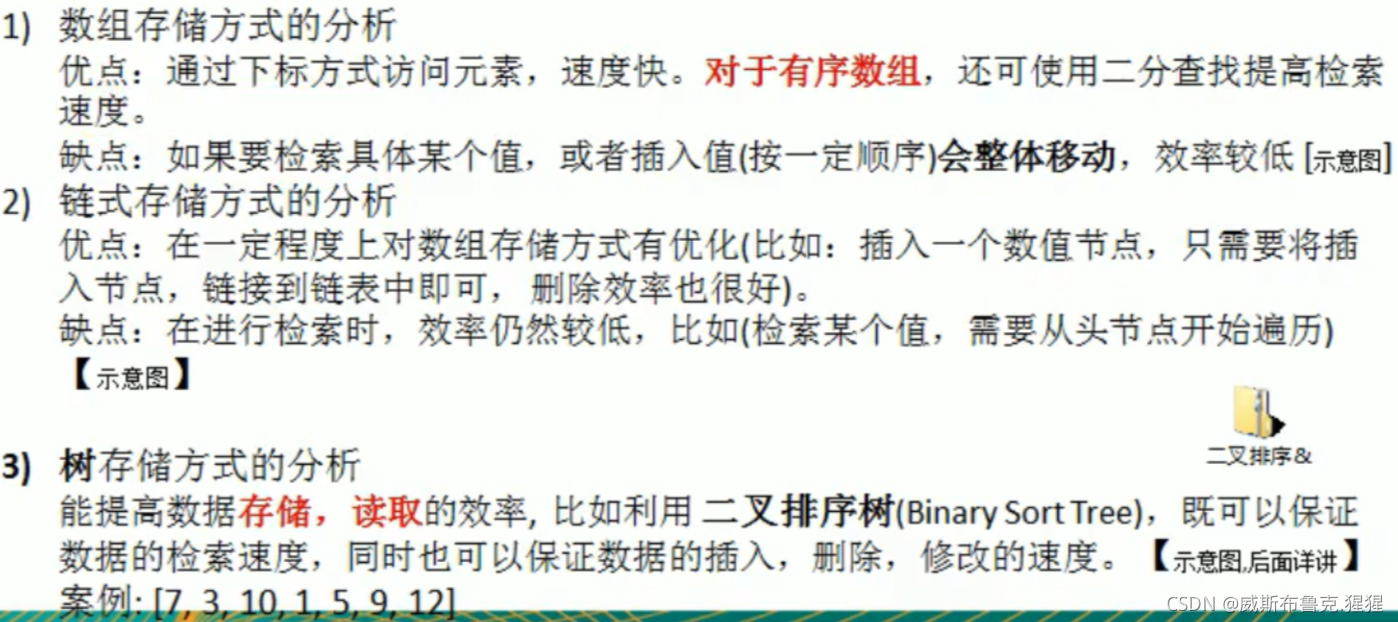
树的示意图?
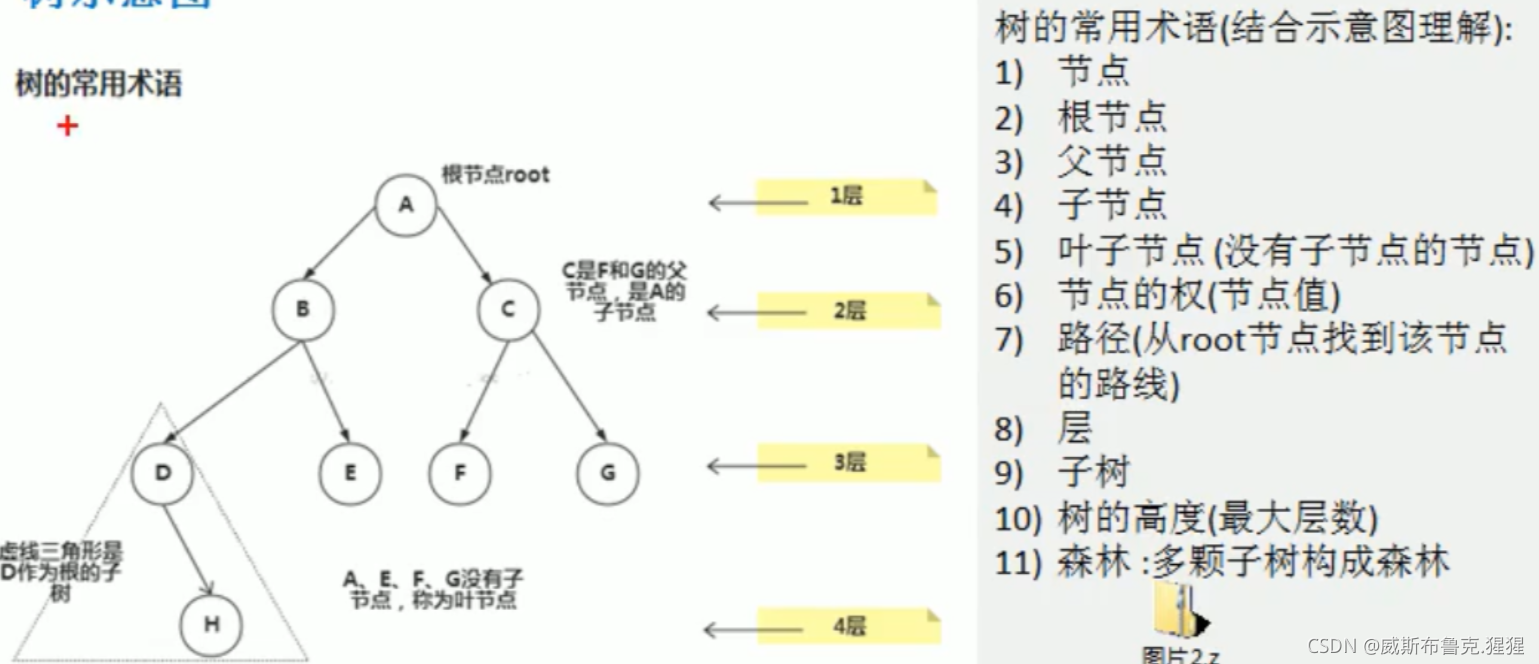
二叉树的概念
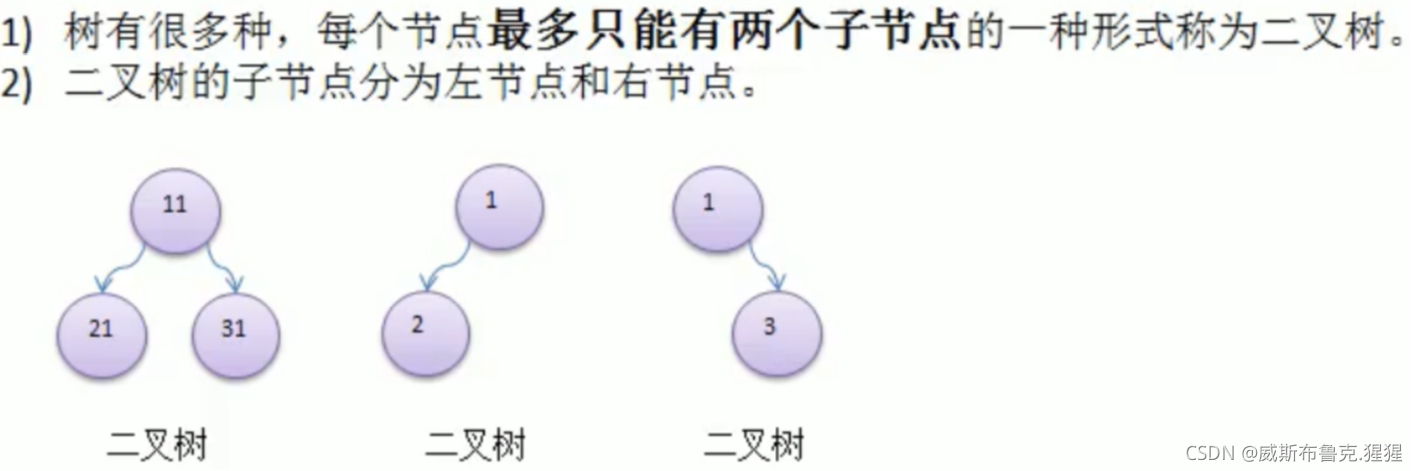
?二叉树遍历的说明
前序遍历:先输出父节点,在遍历左子树和右子树
中序遍历:先遍历左子树,再输出父节点,再遍历右子树
后序遍历:先遍历左子树,再遍历右子树,最后输出父节点
规律总结:看输出父节点的顺序,就确定是前序、中序还是后序
二叉树遍历应用实例(前序、中序、后序)
分析二叉树的前序,中序,后序的遍历步骤
1.创建一颗二叉树
2.前序遍历
2.1先输出当前节点(初始的时候是root节点)
2.2如果左子节点不为空,则递归继续前序遍历
2.3如果右子节点不为空,则递归继续前序遍历
3.中序遍历
3.1如果当前节点的左子节点不为空,则递归中序遍历
3.2输出当前节点
3.3如果当前节点的右子节点不为空,则递归中序遍历
4.后序遍历
4.1如果当前节点的左子节点不为空,则递归后序遍历
4.2如果当前节点的右子节点不为空,则递归后序遍历
4.3输出当前节点
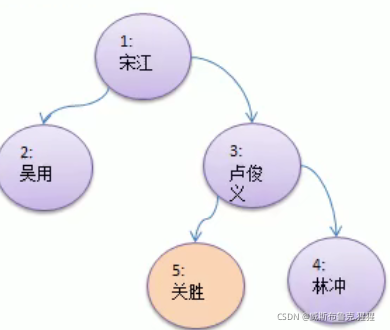
二叉树查找指定节点
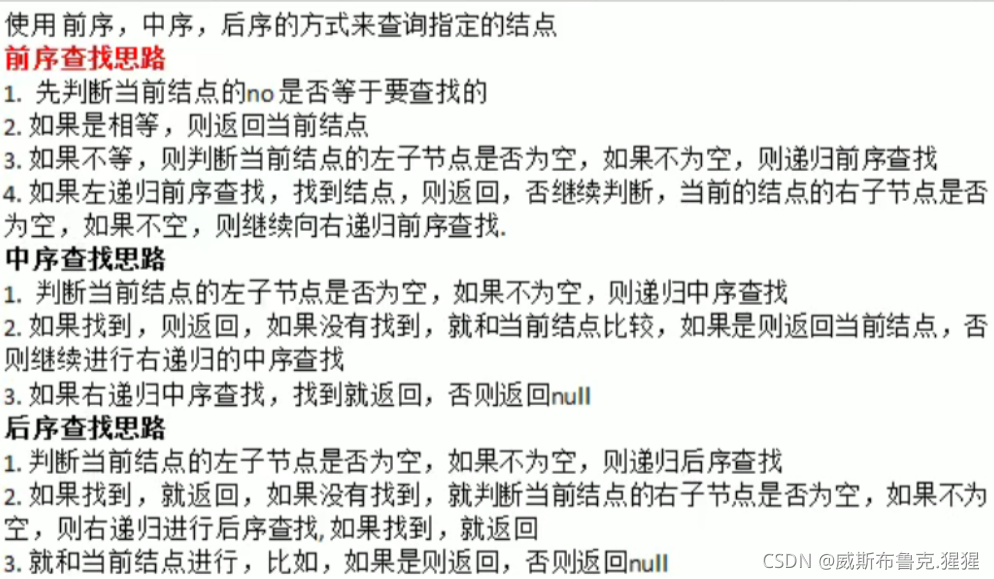
public static void main(String[] args) {
// 先需要创建一棵二叉树
BinaryTree binaryTree = new BinaryTree();
// 创建需要的节点
HeroNode root = new HeroNode(1, "宋江");
HeroNode node2 = new HeroNode(2, "吴用");
HeroNode node3 = new HeroNode(3, "卢俊义");
HeroNode node4 = new HeroNode(4, "林冲");
HeroNode node5 = new HeroNode(5, "关胜");
// 说明,我们先手动创建该二叉树,后面我们学习递归的方式创建二叉树
// 方式一:
root.setLeft(node2);
// 方式二:因为设置left的属性时,权限为private,所以这里不能使用这种方式
// root.left = node2;
root.setRight(node3);
node3.setRight(node4);
node3.setLeft(node5);
binaryTree.setRoot(root);
// 测试
System.out.println("前序遍历");// 1,2,3,5,4
binaryTree.preOrder();
System.out.println("中序遍历");// 2,1,5,3,4
binaryTree.midOrder();
System.out.println("后序遍历");// 2,5,4,3,1
binaryTree.postOrder();
// 前序遍历查找
// System.out.println("前序遍历方式~~~");
// HeroNode resNode = binaryTree.preOrderSearch(5);
// if (resNode != null) {
// System.out.printf("找到了,信息为no=%d name=%s", resNode.getNo(), resNode.getName());
// } else {
// System.out.printf("没有找到 no = %d 的英雄", 5);
// }
// 中序遍历查找
// System.out.println("中序遍历方式~~~");
// HeroNode resNode = binaryTree.midOrderSearch(5);
// if (resNode != null) {
// System.out.printf("找到了,信息为no=%d name=%s", resNode.getNo(), resNode.getName());
// } else {
// System.out.printf("没有找到 no = %d 的英雄", 5);
// }
System.out.println("后序遍历方式~~~");
HeroNode resNode = binaryTree.postOrderSearch(5);
if (resNode != null) {
System.out.printf("找到了,信息为no=%d name=%s", resNode.getNo(), resNode.getName());
} else {
System.out.printf("没有找到 no = %d 的英雄", 5);
}
}
}
//定义BinaryTree二叉树
class BinaryTree {
private HeroNode root;
public void setRoot(HeroNode root) {
this.root = root;
}
// 前序遍历
public void preOrder() {
if (this.root != null) {
this.root.preOrder();
} else {
System.out.println("二叉树为空,无法遍历");
}
}
// 中序遍历
public void midOrder() {
if (this.root != null) {
this.root.midOrder();
} else {
System.out.println("二叉树为空,无法遍历");
}
}
// 后续遍历
public void postOrder() {
if (this.root != null) {
this.root.postOrder();
} else {
System.out.println("二叉树为空,无法遍历");
}
}
// 前序遍历查找
public HeroNode preOrderSearch(int no) {
if (root != null) {
return root.preOrderSearch(no);
} else {
return null;
}
}
// 中序遍历查找
public HeroNode midOrderSearch(int no) {
if (root != null) {
return root.midOrderSearch(no);
} else {
return null;
}
}
// 后序遍历查找
public HeroNode postOrderSearch(int no) {
if (root != null) {
return this.root.postOrderSearch(no);
} else {
return null;
}
}
}
//先创建HeroNode节点
class HeroNode {
private int no;
private String name;
private HeroNode left;// 默认为null
private HeroNode right;// 默认为null
public HeroNode(int no, String name) {
this.no = no;
this.name = name;
}
@Override
public String toString() {
return "HeroNode [no=" + no + ", name=" + name + "]";
}
public int getNo() {
return no;
}
public String getName() {
return name;
}
public HeroNode getLeft() {
return left;
}
public HeroNode getRight() {
return right;
}
public void setNo(int no) {
this.no = no;
}
public void setName(String name) {
this.name = name;
}
public void setLeft(HeroNode left) {
this.left = left;
}
public void setRight(HeroNode right) {
this.right = right;
}
// 前序遍历的方法
public void preOrder() {
System.out.println(this);// 先输出父节点
// 递归向左子树前序遍历
if (this.left != null) {
this.left.preOrder();
}
// 递归向右子树前序遍历
if (this.right != null) {
this.right.preOrder();
}
}
// 中序遍历
public void midOrder() {
// 递归向左子树中序遍历
if (this.left != null) {
this.left.midOrder();
}
// 输出父节点
System.out.println(this);
// 递归向右子树中序遍历
if (this.right != null) {
this.right.midOrder();
}
}
// 后序遍历
public void postOrder() {
if (this.left != null) {
this.left.postOrder();
}
if (this.right != null) {
this.right.postOrder();
}
System.out.println(this);
}
// 前序遍历查找
/**
* @param no 查找no
* @return 如果找到就返回该Node,如果没有找到返回null
*/
public HeroNode preOrderSearch(int no) {
// 比较当前节点是不是
if (this.no == no) {
return this;
}
// 1.则判断当前节点的左子节点是否为空,如果不为空,则递归前序查找
// 2.如果左递归前序查找,找到节点,则返回
HeroNode resNode = null;
if (this.left != null) {
resNode = this.left.preOrderSearch(no);
}
if (resNode != null) {// 说明左子树找到
return resNode;
}
// 1.左递归前序查找,找到节点,则返回,否则继续判断
// 2.当前的节点的右子节点是否为空,如果不空,则继续向右递归前序查找
if (this.right != null) {
resNode = this.right.preOrderSearch(no);
}
return resNode;
}
// 中序遍历查找
public HeroNode midOrderSearch(int no) {
// 判断当前节点的左子节点是否为空,如果不为空,则递归中序查找
HeroNode resNode = null;
if (this.left != null) {
resNode = this.left.midOrderSearch(no);
}
if (resNode != null) {
return resNode;
}
// 如果找到,则返回,如果没有找到,就和当前节点比较,如果是则返回当前节点
if (this.no == no) {
return this;
}
// 如果找到,则返回,如果没有找到,就和当前节点比较,如果是则返回当前节点
if (this.no == no) {
return this;
}
// 否则继续进行右递归的中序查找
if (this.right != null) {
resNode = this.right.midOrderSearch(no);
}
return resNode;
}
// 后序遍历查找
public HeroNode postOrderSearch(int no) {
// 判断当前节点的左子节点是否为空,如果不为空,则递归后序查找
HeroNode resNode = null;
if (this.left != null) {
resNode = this.left.postOrderSearch(no);
}
if (resNode != null) {// 说明在左子树找到
return resNode;
}
// 如果左子树没有找到,则向右子树递归进行后序遍历查找
if (this.right != null) {
resNode = this.right.postOrderSearch(no);
}
if (resNode != null) {
return resNode;
}
// 如果左右子树没有找到,就比较当前节点是不是
if (this.no == no) {
return this;
}
return resNode;
}
}
二叉树 -->> 删除节点
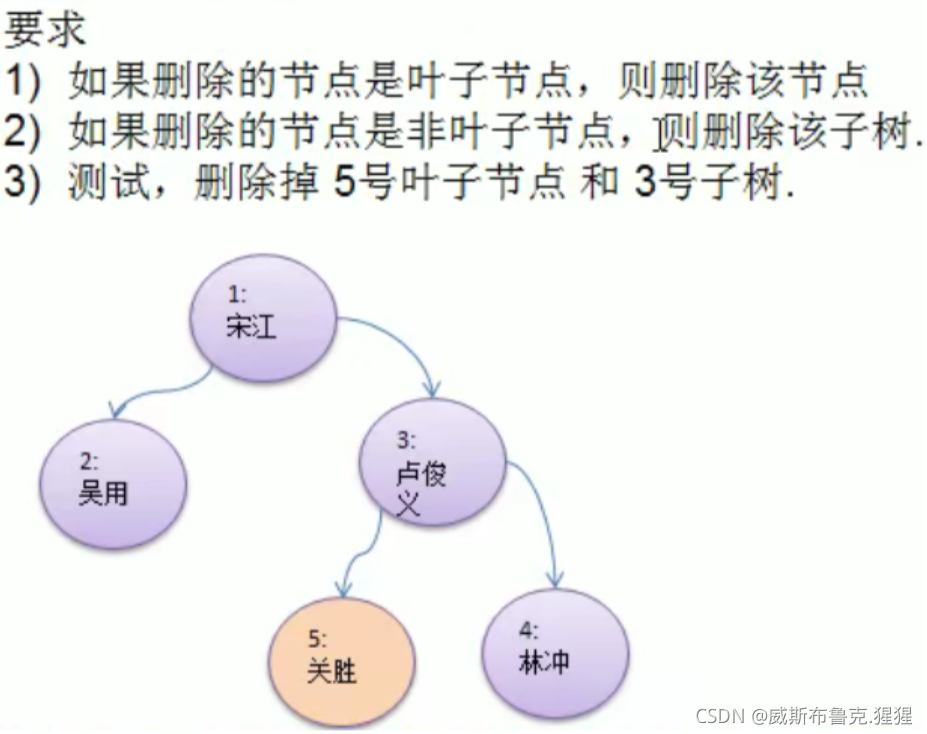
思路 :
首先先处理:考虑如果是空树root,如果只有一个root节点,则等价将二叉树置空;然后进行下面步骤。
1.因为二叉树是单向的,所以是判断当前节点的子节点是否需要删除节点, 而不能去判断当前这个节点是不是需要删除节点 2.如果当前节点的左子节点不为空,并且左子节点就是要删除节点,就将this.left = null; 并且就返回(结束递归删除) 3.如果当前节点的右子节点不为空,并且右子节点就是要删除节点,就将this.right = null; 并且就返回(结束递归删除) 4.如果第2步和第3步没有删除节点,那么就需要向左子树进行递归删除 5.如果第4步也没有删除节点,则应当向右子树进行递归删除。?
HeroNode类中增加了此方法
// 递归删除节点
// 1. 如果删除的节点是叶子节点,则删除该节点
// 2. 如果删除的节点是非叶子节点,则删除该子树
public void delNode(int no) {
// 思路
/*
* 1.因为二叉树是单向的,所以是判断当前节点的子节点是否需要删除节点, 而不能去判断当前这个节点是不是需要删除节点
* 2.如果当前节点的左子节点不为空,并且左子节点就是要删除节点,就将this.left = null; 并且就返回(结束递归删除)
* 3.如果当前节点的右子节点不为空,并且右子节点就是要删除节点,就将this.right = null; 并且就返回(结束递归删除)
* 4.如果第2步和第3步没有删除节点,那么就需要向左子树进行递归删除
* 5.如果第4步也没有删除节点,则应当向右子树进行递归删除。
*/
// 如果当前节点的左子节点不为空,并且左子节点就是要删除节点,就将this.left = null;并且就返回(结束递归删除)
if (this.left != null && this.left.no == no) {
this.left = null;
return;
}
// 如果当前节点的右子节点不为空,并且右子节点就是要删除节点,就将this.right = null;并且就返回(结束递归删除)
if (this.right != null && this.right.no == no) {
this.right = null;
return;
}
// 向左子树进行递归删除
if (this.left != null) {
this.left.delNode(no);
}
// 向右子树进行递归删除
if (this.right != null) {
this.right.delNode(no);
}
}
BinaryTree类中增加了方法
// 删除节点
public void delNode(int no) {
if (root != null) {
// 如果只有一个root节点,这里立即判断root是不是就是要删除节点
if (root.getNo() == no) {
root = null;
} else {
// 递归删除
root.delNode(no);
}
} else {
System.out.println("空树,不能删除~~");
}
}
顺序存储二叉树
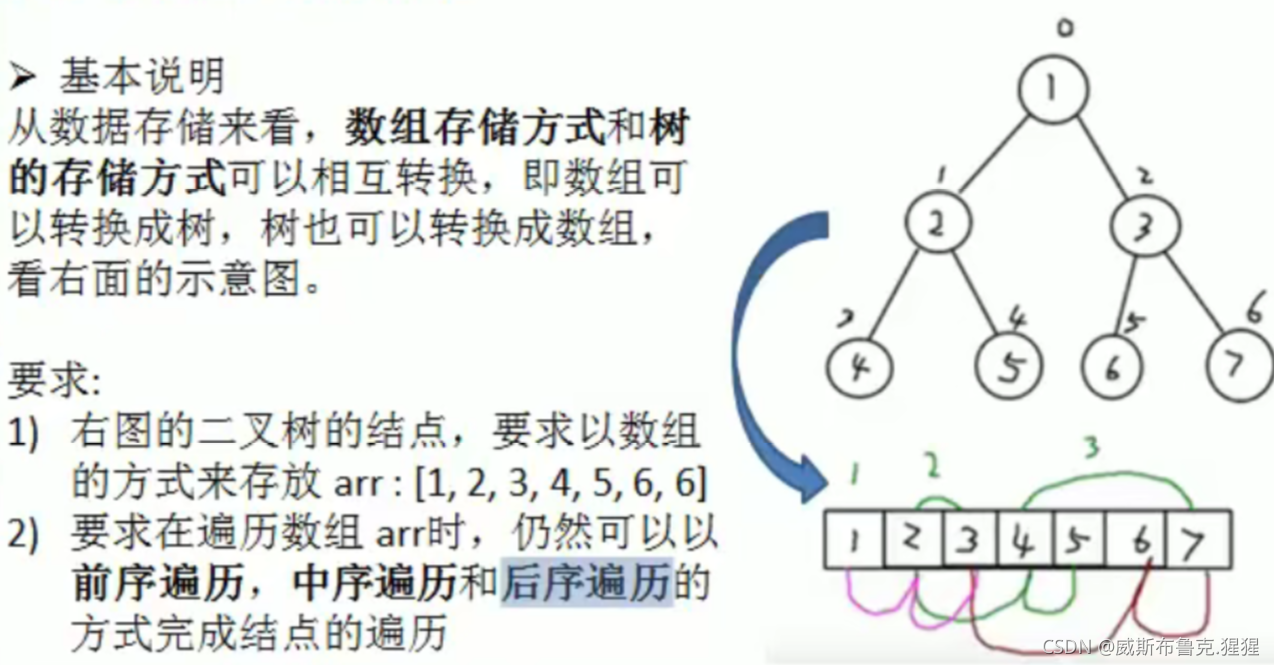
?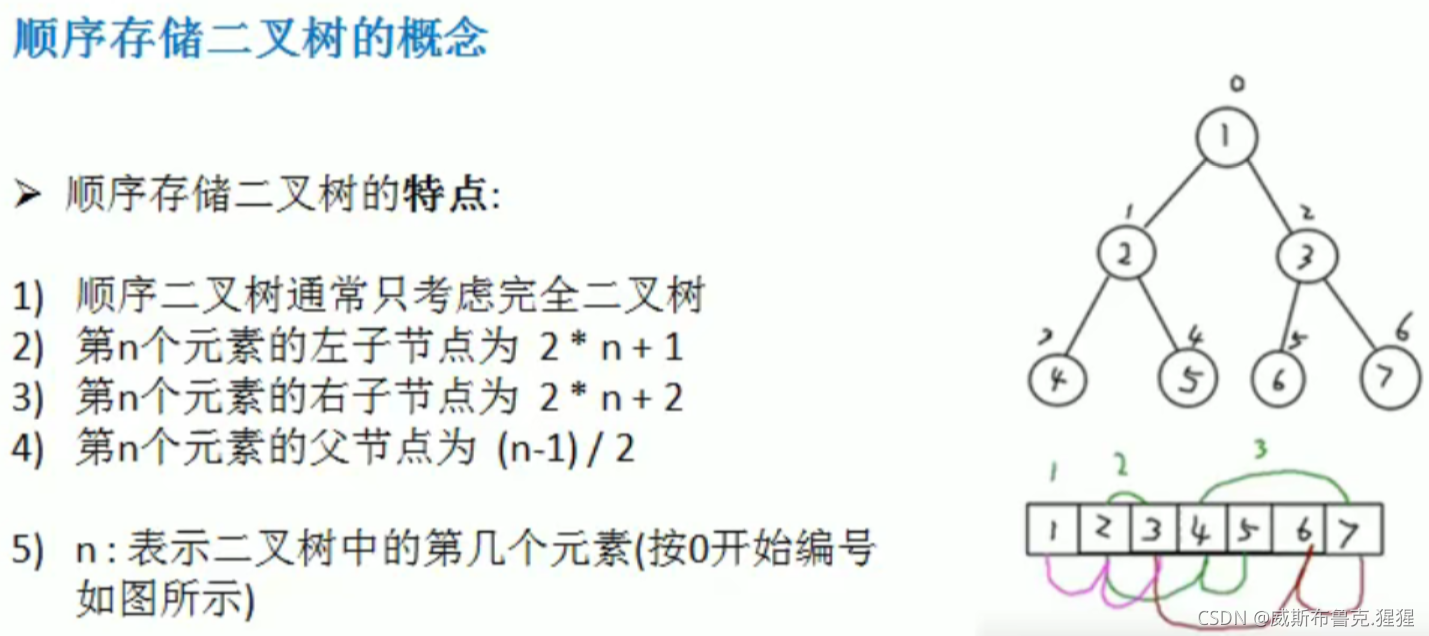
public class ArrayBinaryTreeDemo {
public static void main(String[] args) {
int[] arr = { 1, 2, 3, 4, 5, 6, 7 };
// 创建一个ArrBinaryTree
ArrBinaryTree arrBinaryTree = new ArrBinaryTree(arr);
arrBinaryTree.preOrder();// 1,2,4,5,3,6,7
}
}
//编写一个ArrayBinaryTree,实现顺序存储二叉树遍历
class ArrBinaryTree {
private int[] arr;// 存储数据节点的数组
public ArrBinaryTree(int[] arr) {
this.arr = arr;
}
// 重载preOrder
public void preOrder() {
this.preOrder(0);
}
// 编写一个方法,完成顺序存储二叉树的前序遍历
/**
* @param index 数组的下标
*/
public void preOrder(int index) {
// 如果数组为空,或者arr.length = 0
if (arr == null || arr.length == 0) {
System.out.println("数组为空,不能按照二叉树的前序遍历");
}
// 输出当前这个元素
System.out.println(arr[index]);
// 向左递归遍历
if ((index * 2 + 1) < arr.length) {
preOrder(2 * index + 1);
}
// 向右递归遍历
if ((index * 2 + 2) < arr.length) {
preOrder(2 * index + 2);
}
}
}
?顺序存储二叉树应用实例
八大排序算法中的堆排序中,应用了顺序存储排序二叉树
线索化二叉树
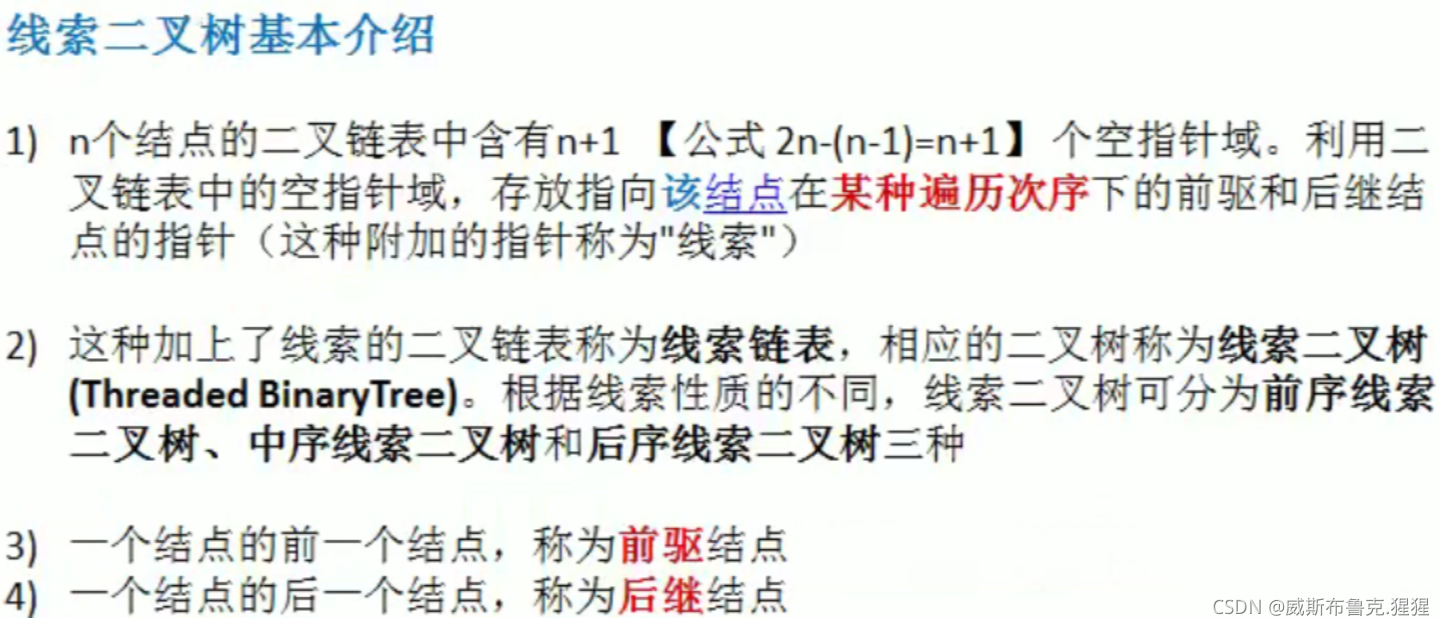
?
当对上面的二叉树进行中序遍历时,数列为{8,3,10,1,6,14}
以上面的二叉树为例(中序遍历时):节点8没有前驱节点,节点14没有后继节点;
节点3的前驱节点为8,后继节点为10.
线索二叉树应用实例
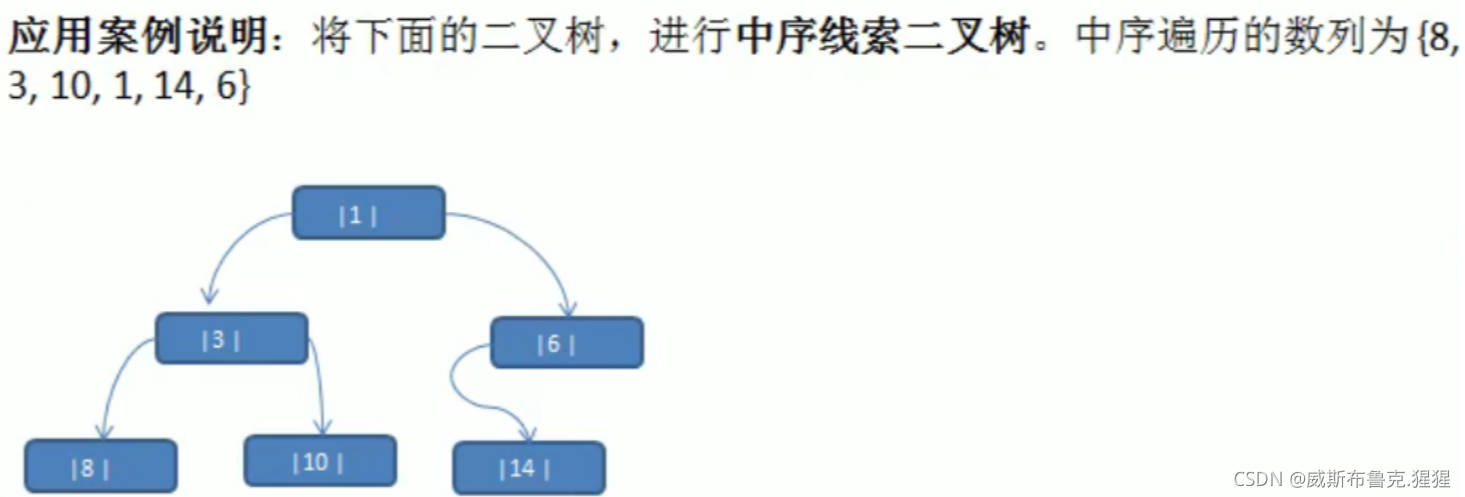
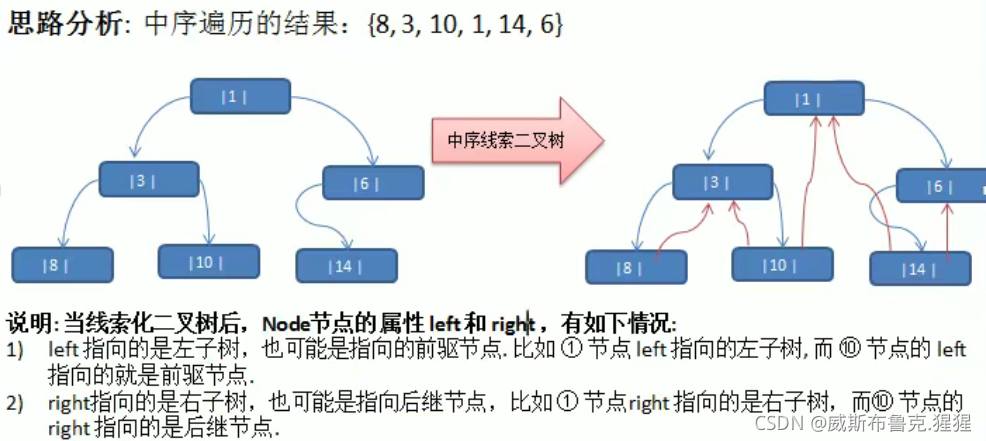
遍历线索化二叉树
说明:对前面的中序线索化的二叉树,进行遍历
分析:因为线索化后,各个节点指向有变化,因此原来的遍历方式不能使用,这时需要使用新的方式遍历线索化二叉树,各个节点可以通过线性方式遍历。因此无需使用递归方式,这样也提高了遍历的效率。遍历的次序应当和中序遍历保持一致。?
public class ThreadedBinaryTreeDemo {
public static void main(String[] args) {
// 测试中序线索二叉树的功能
HeroNode root = new HeroNode(1, "tom");
HeroNode node2 = new HeroNode(3, "jack");
HeroNode node3 = new HeroNode(6, "smith");
HeroNode node4 = new HeroNode(8, "mary");
HeroNode node5 = new HeroNode(10, "king");
HeroNode node6 = new HeroNode(14, "dim");
// 二叉树,后面递归创建,现在简答处理使用手动创建
root.setLeft(node2);
root.setRight(node3);
node2.setRight(node4);
node2.setRight(node5);
node3.setLeft(node6);
// 测试中序线索化
ThreadedBinaryTree threadedBinaryTree = new ThreadedBinaryTree();
threadedBinaryTree.setRoot(root);
threadedBinaryTree.threadedNodes();
// 测试:以10号节点测试
HeroNode leftNode = node5.getLeft();
HeroNode rightNode = node5.getRight();
System.out.println("10号节点的前驱节点是=" + leftNode);// 3
System.out.println("10号节点的后继节点是=" + rightNode);// 1
// 当线索化二叉树后,不能再使用原来的遍历方法
System.out.println("使用线索化的方式遍历线索化二叉树");
threadedBinaryTree.threadedList();// 8,3,10,1,14,6
}
}
//定义ThreadedBinaryTree 实现了线索化功能的二叉树
class ThreadedBinaryTree {
private HeroNode root;
// 为了实现线索化,需要创建一个指向当前节点的前驱节点的指针
// 在递归进行线索化时,pre总是保留前一个节点
private HeroNode pre = null;
public void setRoot(HeroNode root) {
this.root = root;
}
// 重载threadedNodes方法
public void threadedNodes() {
this.threadedNodes(root);
}
// 遍历线索化二叉树的方法
public void threadedList() {
// 定义一个变量,存储当前遍历的节点,从root开始
HeroNode node = root;
while (node != null) {
// 循环的找到leftType == 1的节点,第一个找到就是8节点
// 后面随着遍历而变化,因为当leftType == 1时,说明该节点时按照线索化
// 处理后的有效节点
while (node.getLeftType() == 0) {
node = node.getLeft();
}
// 打印当前这个节点
System.out.println(node);
// 如果当前节点的右指针指向的是后继节点,就一直输出
while (node.getRightType() == 1) {
// 获取到当前节点的后继节点
node = node.getRight();
System.out.println(node);
}
// 替换这个遍历的节点
node = node.getRight();
}
}
// 编写对二叉树进行中序线索化的方法
/**
* @param node 就是当前需要线索化的节点
*/
public void threadedNodes(HeroNode node) {
// 如果node == null,不能线索化
if (node == null) {
return;
}
// (一)先线索化左子树
threadedNodes(node.getLeft());
// (二)线索化当前节点【有难度】
// 处理当前节点的前驱节点
// 以8节点来理解
// 8节点的.left = null,8节点的.leftType = 1
if (node.getLeft() == null) {
// 让当前节点的左指针指向前驱节点
node.setLeft(pre);
// 修改当前节点的左指针的类型,指向前驱节点
node.setLeftType(1);
}
// 处理后继节点
if (pre != null && pre.getRight() == null) {
// 让前驱节点的右指针指向当前节点
pre.setRight(node);
// 修改前驱节点的右指针类型
pre.setRightType(1);
}
// !!!每处理一个节点后,让当前节点时下一个节点的前驱节点
pre = node;
// (三)线索化右子树
threadedNodes(node.getRight());
}
// 删除节点
public void delNode(int no) {
if (root != null) {
// 如果只有一个root节点,这里立即判断root是不是就是要删除节点
if (root.getNo() == no) {
root = null;
} else {
// 递归删除
root.delNode(no);
}
} else {
System.out.println("空树,不能删除~~");
}
}
// 前序遍历
public void preOrder() {
if (this.root != null) {
this.root.preOrder();
} else {
System.out.println("二叉树为空,无法遍历");
}
}
// 中序遍历
public void midOrder() {
if (this.root != null) {
this.root.midOrder();
} else {
System.out.println("二叉树为空,无法遍历");
}
}
// 后续遍历
public void postOrder() {
if (this.root != null) {
this.root.postOrder();
} else {
System.out.println("二叉树为空,无法遍历");
}
}
// 前序遍历查找
public HeroNode preOrderSearch(int no) {
if (root != null) {
return root.preOrderSearch(no);
} else {
return null;
}
}
// 中序遍历查找
public HeroNode midOrderSearch(int no) {
if (root != null) {
return root.midOrderSearch(no);
} else {
return null;
}
}
// 后序遍历查找
public HeroNode postOrderSearch(int no) {
if (root != null) {
return this.root.postOrderSearch(no);
} else {
return null;
}
}
}
//创建HeroNode
class HeroNode {
private int no;
private String name;
private HeroNode left;// 默认为null
private HeroNode right;// 默认为null
// 说明
// 1.如果leftType == 0表示指向的是左子树,如果1则表示指向前驱节点
// 2.如果rightType == 0表示指向是右子树,如果1表示指向后继节点
private int leftType;
private int rightType;
public int getLeftType() {
return leftType;
}
public int getRightType() {
return rightType;
}
public void setLeftType(int leftType) {
this.leftType = leftType;
}
public void setRightType(int rightType) {
this.rightType = rightType;
}
public HeroNode(int no, String name) {
this.no = no;
this.name = name;
}
@Override
public String toString() {
return "HeroNode [no=" + no + ", name=" + name + "]";
}
public int getNo() {
return no;
}
public String getName() {
return name;
}
public HeroNode getLeft() {
return left;
}
public HeroNode getRight() {
return right;
}
public void setNo(int no) {
this.no = no;
}
public void setName(String name) {
this.name = name;
}
public void setLeft(HeroNode left) {
this.left = left;
}
public void setRight(HeroNode right) {
this.right = right;
}
// 递归删除节点
// 1. 如果删除的节点是叶子节点,则删除该节点
// 2. 如果删除的节点是非叶子节点,则删除该子树
public void delNode(int no) {
// 思路
/*
* 1.因为二叉树是单向的,所以是判断当前节点的子节点是否需要删除节点, 而不能去判断当前这个节点是不是需要删除节点
* 2.如果当前节点的左子节点不为空,并且左子节点就是要删除节点,就将this.left = null; 并且就返回(结束递归删除)
* 3.如果当前节点的右子节点不为空,并且右子节点就是要删除节点,就将this.right = null; 并且就返回(结束递归删除)
* 4.如果第2步和第3步没有删除节点,那么就需要向左子树进行递归删除 5.如果第4步也没有删除节点,则应当向右子树进行递归删除。
*/
// 如果当前节点的左子节点不为空,并且左子节点就是要删除节点,就将this.left = null;并且就返回(结束递归删除)
if (this.left != null && this.left.no == no) {
this.left = null;
return;
}
// 如果当前节点的右子节点不为空,并且右子节点就是要删除节点,就将this.right = null;并且就返回(结束递归删除)
if (this.right != null && this.right.no == no) {
this.right = null;
return;
}
// 向左子树进行递归删除
if (this.left != null) {
this.left.delNode(no);
}
// 向右子树进行递归删除
if (this.right != null) {
this.right.delNode(no);
}
}
// 前序遍历的方法
public void preOrder() {
System.out.println(this);// 先输出父节点
// 递归向左子树前序遍历
if (this.left != null) {
this.left.preOrder();
}
// 递归向右子树前序遍历
if (this.right != null) {
this.right.preOrder();
}
}
// 中序遍历
public void midOrder() {
// 递归向左子树中序遍历
if (this.left != null) {
this.left.midOrder();
}
// 输出父节点
System.out.println(this);
// 递归向右子树中序遍历
if (this.right != null) {
this.right.midOrder();
}
}
// 后序遍历
public void postOrder() {
if (this.left != null) {
this.left.postOrder();
}
if (this.right != null) {
this.right.postOrder();
}
System.out.println(this);
}
// 前序遍历查找
/**
* @param no 查找no
* @return 如果找到就返回该Node,如果没有找到返回null
*/
public HeroNode preOrderSearch(int no) {
// 比较当前节点是不是
if (this.no == no) {
return this;
}
// 1.则判断当前节点的左子节点是否为空,如果不为空,则递归前序查找
// 2.如果左递归前序查找,找到节点,则返回
HeroNode resNode = null;
if (this.left != null) {
resNode = this.left.preOrderSearch(no);
}
if (resNode != null) {// 说明左子树找到
return resNode;
}
// 1.左递归前序查找,找到节点,则返回,否则继续判断
// 2.当前的节点的右子节点是否为空,如果不空,则继续向右递归前序查找
if (this.right != null) {
resNode = this.right.preOrderSearch(no);
}
return resNode;
}
// 中序遍历查找
public HeroNode midOrderSearch(int no) {
// 判断当前节点的左子节点是否为空,如果不为空,则递归中序查找
HeroNode resNode = null;
if (this.left != null) {
resNode = this.left.midOrderSearch(no);
}
if (resNode != null) {
return resNode;
}
// 如果找到,则返回,如果没有找到,就和当前节点比较,如果是则返回当前节点
if (this.no == no) {
return this;
}
// 如果找到,则返回,如果没有找到,就和当前节点比较,如果是则返回当前节点
if (this.no == no) {
return this;
}
// 否则继续进行右递归的中序查找
if (this.right != null) {
resNode = this.right.midOrderSearch(no);
}
return resNode;
}
// 后序遍历查找
public HeroNode postOrderSearch(int no) {
// 判断当前节点的左子节点是否为空,如果不为空,则递归后序查找
HeroNode resNode = null;
if (this.left != null) {
resNode = this.left.postOrderSearch(no);
}
if (resNode != null) {// 说明在左子树找到
return resNode;
}
// 如果左子树没有找到,则向右子树递归进行后序遍历查找
if (this.right != null) {
resNode = this.right.postOrderSearch(no);
}
if (resNode != null) {
return resNode;
}
// 如果左右子树没有找到,就比较当前节点是不是
if (this.no == no) {
return this;
}
return resNode;
}
}
?
|