复习排序算法
1. 冒泡排序
ArrayList.prototype.bubbleSort = () => {
const len = this.data.length
for (let i = 0; i < len - 1; i++) {
for (let j = 0; j < len - 1 - i; j++) {
if (this.data[j] > this.data[j + 1]) {
const temp = this.data[j]
this.data[j] = this.data[j + 1]
this.data[j + 1] = temp
}
}
}
}
2. 选择排序
ArrayList.prototype.selectSort = () => {
const len = this.data.length
let min = 0
for (let i = 0; i < len - 1; i++) {
for (let j = i + 1; j < len; j++) {
if (this.data[j] < this.data[min]) {
min = j
}
}
const temp = this.data[min]
this.data[min] = this.data[i]
this.data[i] = temp
}
}
?
3. 插入排序
ArrayList.prototype.insertSort = () => {
const len = this.data.length
for (let i = 1; i < len; i++) {
const temp = this.data[i]
let j = i
while (j > 0 && this.data[j - 1] > temp) {
this.data[j] = this.data[j - 1]
j--
}
this.data[j] = temp
}
}
4.希尔排序
ArrayList.prototype.shellSort = () => {
const len = this.data.length
let interval = Math.floor(len / 2)
while (interval >= 1) {
for (let i = interval; i < len; i++) {
const temp = this.data[i]
let j = i
while (temp < this.data[j - interval] && (j - interval) >= 0) {
this.data[j] = this.data[j - interval]
j -= interval
}
this.data[j] = temp
}
interval = Math.floor(interval / 2)
}
}
?
5.快速排序
- 思路:首先取一个元素(一般取数组的第一个元素,或者是挑选数组的第一个元素、中间元素、最后一个元素三个数的中位数)作为 中心;将所有比中心小的元素全部放到中心的左边位置,比中心大的元素全部放到中心的右边位置,以中心元素为界就形成了 两个子序列,然后对每个子序列重新选择中心元素并按照以上规则执行,直到每个子序列的元素只剩下一个
- 基本操作:
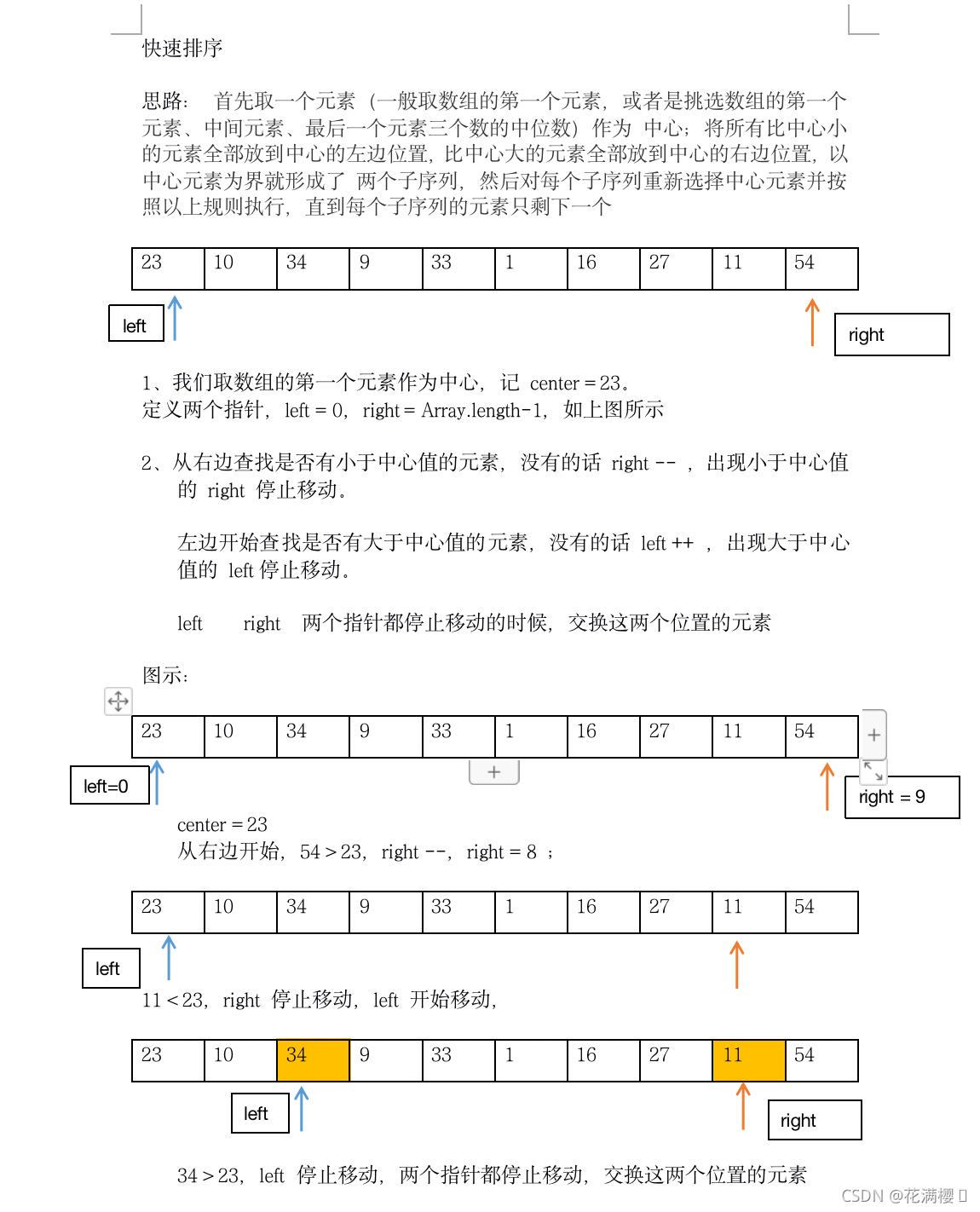
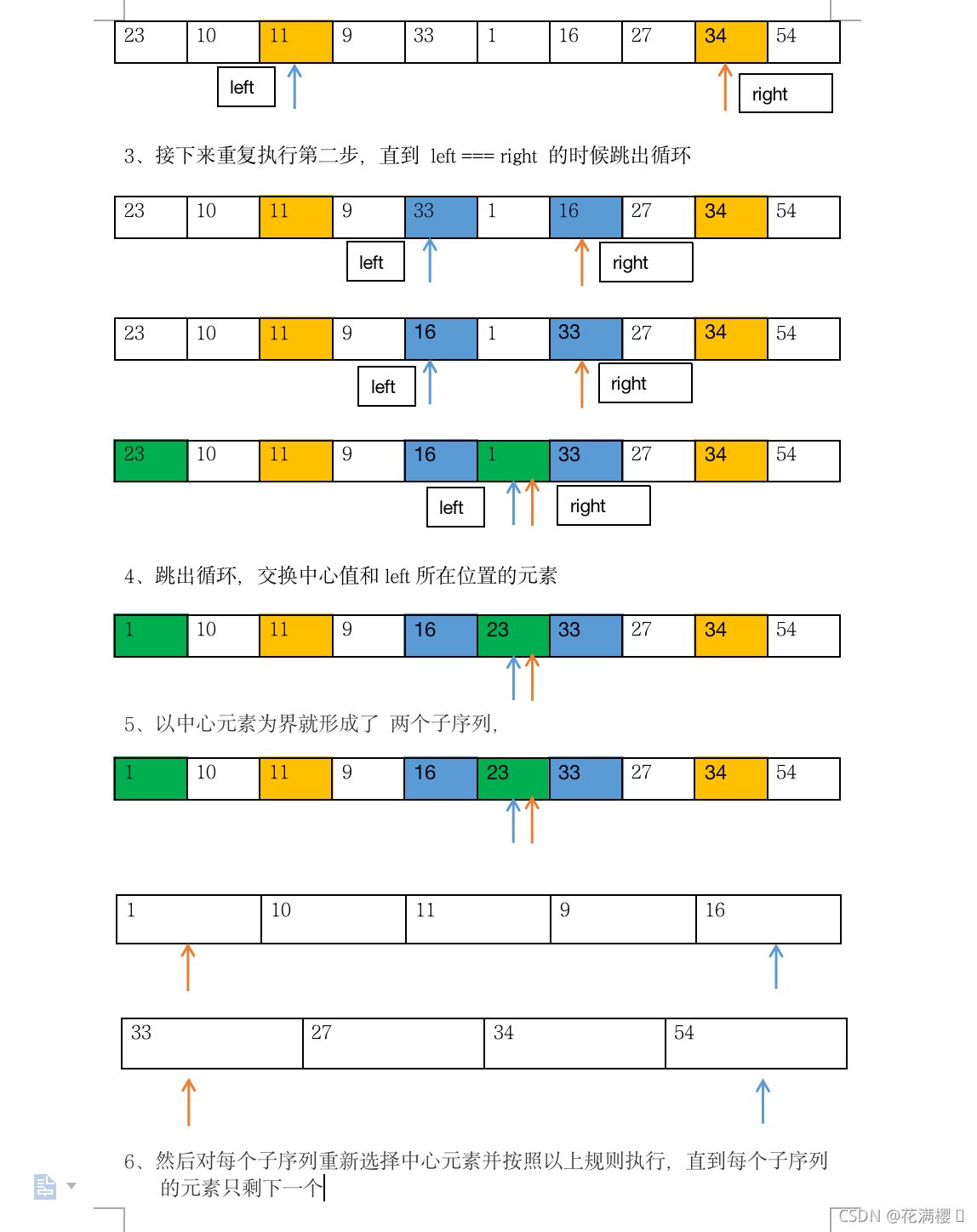
ArrayList.prototype.quickSort = () => {
const quickSort = (data, left, right) => {
if (left >= right) return
let l = left, r = right
const ele = data[l]
while (l < r) {
while (data[r] > ele && r > l) {
r--
}
while (data[l] <= ele && r > l) {
l++
}
const temp = data[l]
data[l] = data[r]
data[r] = temp
}
data[left] = data[l]
data[l] = ele
quickSort(data, left, l - 1)
quickSort(data, l + 1, right)
}
quickSort(this.data, 0, this.data.length - 1)
}
|