题目
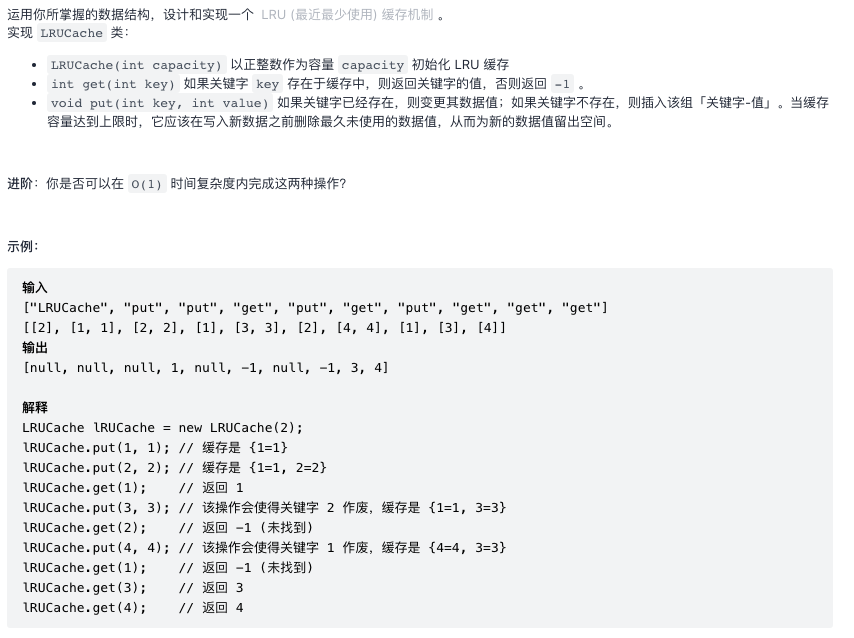 
解题:双向链表+散列表
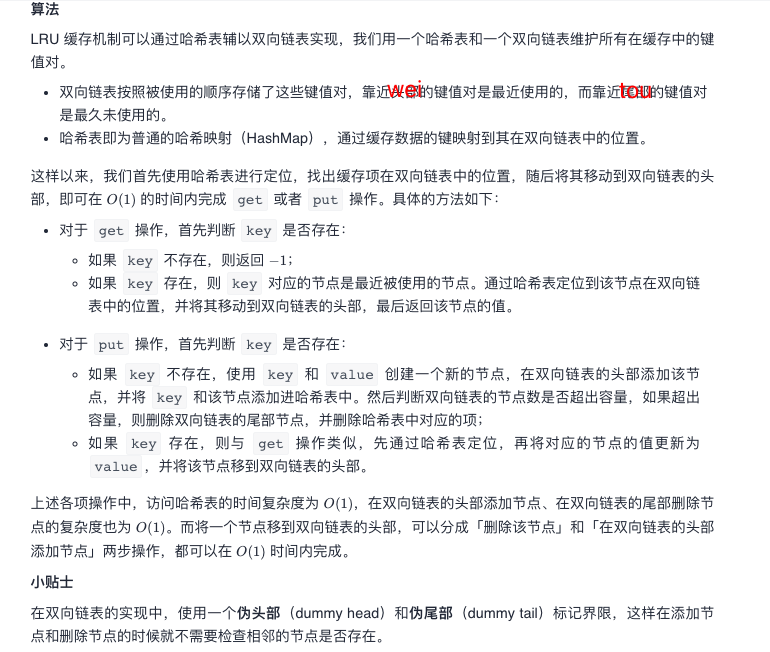
var DoubleLinkedListNode = function(key, value) {
this.key = key;
this.val = value;
this.prev = null;
this.next = null;
};
var LRUCache = function(capacity) {
this.cap = capacity;
this.usedSpace = 0;
this.cache = new Map();
this.head = new DoubleLinkedListNode(undefined, undefined);
this.tail = new DoubleLinkedListNode(undefined, undefined);
this.head.next = this.tail;
this.tail.prev = this.head;
};
LRUCache.prototype.removeNode = function(node) {
node.prev.next = node.next;
node.next.prev = node.prev;
node.prev = null;
node.next = null;
};
LRUCache.prototype.addToTail = function(node) {
this.tail.prev.next = node;
node.prev = this.tail.prev;
this.tail.prev = node;
node.next = this.tail;
};
LRUCache.prototype.get = function(key) {
if (this.cache.has(key) === true) {
let node = this.cache.get(key);
this.removeNode(node);
this.addToTail(node);
return node.val;
}
return -1;
};
LRUCache.prototype.put = function(key, value) {
if (this.get(key) !== -1) {
(this.cache.get(key)).val = value;
} else {
if (this.usedSpace >= this.cap) {
let delNode = this.head.next;
this.removeNode(delNode);
this.usedSpace--;
this.cache.delete(delNode.key);
}
let newNode = new DoubleLinkedListNode(key, value);
this.addToTail(newNode);
this.usedSpace++;
this.cache.set(key, newNode);
}
};
时间复杂度:对于 put 和 get 都是
O
(
1
)
O(1)
O(1)。
空间复杂度:
O
(
c
a
p
a
c
i
t
y
)
O(capacity)
O(capacity)。
|