##2021.10.2 ? ?周六
算术运算符:
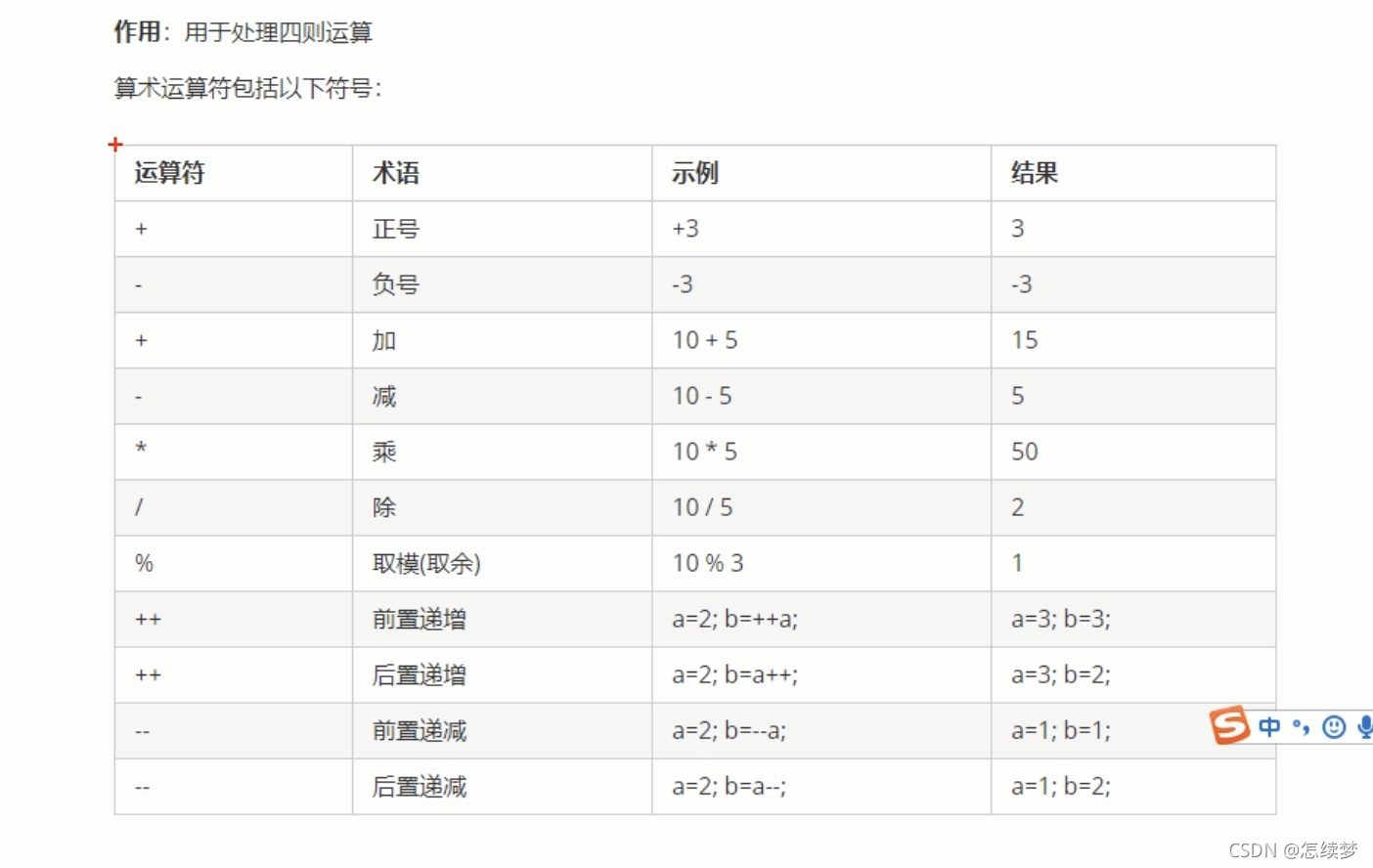
加减乘除运算:?+、-、*、/
#include <iostream>
using namespace std;
int main()
{
//两个数相加减
int a = 5;
int b = 3;
cout << a + b << endl;
cout << a - b << endl;
//两个数相乘除
cout << a * b << endl;
cout << a / b << endl;//两个整数相除结果依然是整数,将小数部分去除,直接去除,不会四舍五入
//除数(b)不能为零
//两个小数间的加减乘除
double c = 0.5;
double d = 0.25;
cout << c + d << endl;
cout << c - d << endl;
cout << c * d << endl;
cout << c / d << endl;//小数相除结果可以是整数也可以是小数
system(“pause“);
return 0;
}
取模(取余)运算符:%
#include <iostream>
using namespace std;
int main()
{
//取模运算的本质就是取余数
int a = 10;
int b = 3;
cout << a % b << endl;//输出结果为:1
int c = 10;
int d = 20;
cout << c % d << endl;
//输出结果为:10
//除数不能为零
//两个小数不可以做取模运算
system(“pause“);
return 0;
}
递增递减运算符:++、--
#include<iostream>
using namespace std;
int main()
{
//前置递增
int a = 10;
++a;//让变量+1
cout << a << endl;//输出结果为:11
//后置递增
int b = 10;
b++;//让变量+1
cout << b << endl;//输出结果为:11
/*二者区别:
前置:在使用变量之前让变量+、-1
后置:在使用变量之后让变量+、-1 */
int c = 10;
int d = ++c * 10;
cout << "c的值为" << c << endl;
cout << "d的值为" << d << endl;
//输出结果为:
//c的值为11
//d的值为110
int e = 10;
int f = e++ * 10;
cout << "e的值为" << e << endl;
cout << "f的值为" << f << endl;
//输出结果:
//e的值为11
//f的值为100
system("pause");
return 0;
}
END
|