import numpy as np
import matplotlib.pyplot as plt
X = np.array([2.5, 0.5, 2.2, 1.9, 3.1, 2.3, 2.0, 1.0, 1.5, 1.1])
Y = np.array([2.4, 0.7, 2.9, 2.2, 3.0, 2.7, 1.6, 1.1, 1.6, 0.9])
X1 = sum(X) / len(X)
Y1 = sum(Y) / len(Y)
for i in range(len(X)):
X[i] = X[i] - X1
Y[i] = Y[i] - Y1
X = X[:, np.newaxis]
Y = Y[:, np.newaxis]
D = np.hstack((X, Y))
print("减去均值后的矩阵")
print(D)
cov_mat = np.cov(D.T)
print("协方差矩阵")
print(cov_mat)
eigen_vals, eigen_vecs = np.linalg.eig(cov_mat)
print("特征值")
print("eigen_vals = ", eigen_vals)
print("特征向量")
print("eigen_vecs = ", eigen_vecs)
Max_value = max(eigen_vals)
feature = np.array(eigen_vecs[:, 1])
finalData = D.dot(feature.transpose())
print("投影后的数据")
print(finalData)
运行结果
"D:\py project\exer\venv\Scripts\python.exe" "D:/py project/exer/PCA.py"
减去均值后的矩阵
[[ 0.69 0.49]
[-1.31 -1.21]
[ 0.39 0.99]
[ 0.09 0.29]
[ 1.29 1.09]
[ 0.49 0.79]
[ 0.19 -0.31]
[-0.81 -0.81]
[-0.31 -0.31]
[-0.71 -1.01]]
协方差矩阵
[[0.61655556 0.61544444]
[0.61544444 0.71655556]]
特征值
eigen_vals = [0.0490834 1.28402771]
特征向量
eigen_vecs = [[-0.73517866 -0.6778734 ]
[ 0.6778734 -0.73517866]]
投影后的数据
[-0.82797019 1.77758033 -0.99219749 -0.27421042 -1.67580142 -0.9129491
0.09910944 1.14457216 0.43804614 1.22382056]
Process finished with exit code 0
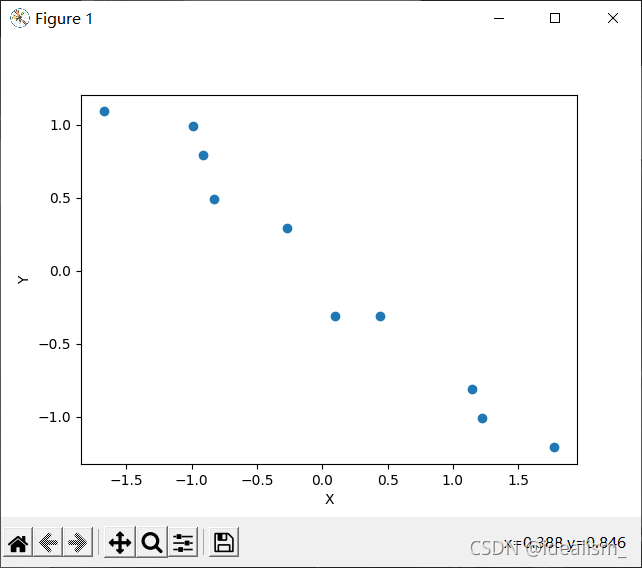
|