一、CMatrix类的代码实现
1. main.cpp
#include <iostream>
#include "CComplex.h"
#include <stdio.h>
#include "CMatrix.h"
using namespace std;
int main(int argc, char** argv) {
CMatrix m;
cin >> m;
cout << "m = " << m << endl;
double pData[10] = { 1,2,3,4,5 };
CMatrix m1(2, 5, pData);
CMatrix m2("1.txt");
cout << "m2 = \n" << m2 << endl;
CMatrix m3(m1);
CMatrix m4;
m4 = m1;
m1.Set(0, 1, 20);
cout << "m3 = \n" << m3 << endl;
cout << "m1 = \n" << m1 << endl;
cout << "m4 = \n" << m4 << endl;
m4 = m4;
cout << "new m4 = \n" << m4 << endl;
cout << "m4[8] = " << m4[8] << endl;
m4(1, 1) = 10;
cout << "m4(1,1) = " << m4(1, 1) << endl;
if (m4 == m1) {
cout << "Error!" << endl;
}
CMatrix m5;
m5 = m1 + m4;
cout << "m5 = \n" << m5 << endl;
double d = m5;
cout << "sum of m5 = " << d << endl;
return 0;
}
2. CMatrix.h
#pragma once
#ifndef CMATRIX_H
#define CMATRIX_H
#include <iostream>
using namespace std;
class CMatrix {
public:
CMatrix();
CMatrix(int nRow, int nCol, double* pData = NULL);
CMatrix(const CMatrix& m);
CMatrix(const char* strPath);
~CMatrix();
bool Create(int nRow, int nCol, double* pData = NULL);
void Set(int nRow, int nCol, double dVal);
void Release();
friend istream& operator>>(istream& is, CMatrix& m);
friend ostream& operator<<(ostream& os, const CMatrix& m);
CMatrix& operator=(const CMatrix& m);
CMatrix& operator+=(const CMatrix& m);
double& operator[](int nIndex);
double& operator()(int nRow, int nCol);
bool operator ==(const CMatrix& m);
bool operator !=(const CMatrix& m);
operator double();
private:
int m_nRow;
int m_nCol;
double* m_pData;
};
CMatrix operator+(const CMatrix& m1, const CMatrix& m2);
inline void CMatrix::Set(int nRow, int nCol, double dVal) {
m_pData[nRow * m_nCol + nCol] = dVal;
}
#endif
3. CMatrix.cpp
#include "CMatrix.h"
#include <fstream>
#include <assert.h>
CMatrix::CMatrix() :m_nRow(0), m_nCol(0), m_pData(NULL){
}
CMatrix::CMatrix(int nRow, int nCol, double* pData) : m_pData(NULL) {
Create(nRow, nCol, pData);
}
CMatrix::CMatrix(const CMatrix& m) : m_pData(NULL) {
*this = m;
}
CMatrix::CMatrix(const char* strPath) {
m_pData = NULL;
m_nRow = m_nCol = 0;
ifstream is(strPath);
is >> *this;
}
CMatrix::~CMatrix() {
Release();
}
bool CMatrix::Create(int nRow, int nCol, double* pData) {
Release();
m_nRow = nRow;
m_nCol = nCol;
m_pData = new double[nRow * nCol];
if (m_pData != NULL) {
if (pData!=NULL) {
memcpy(m_pData, pData, nRow * nCol * sizeof(double));
}
}
else {
return false;
}
return true;
}
void CMatrix::Release() {
if (m_pData!=NULL) {
delete []m_pData;
m_pData = NULL;
}
m_nRow = m_nCol = 0;
}
CMatrix& CMatrix::operator=(const CMatrix& m) {
if (this != &m) {
Create(m.m_nRow, m.m_nCol, m.m_pData);
}
return *this;
}
CMatrix& CMatrix::operator+=(const CMatrix& m) {
assert(m_nRow == m.m_nRow && m_nCol == m.m_nCol);
for (int i = 0; i < m_nRow*m_nCol; i++){
m_pData[i] += m.m_pData[i];
}
return *this;
}
CMatrix operator+(const CMatrix& m1, const CMatrix& m2) {
CMatrix m3(m1);
m3 += m2;
return m3;
}
double& CMatrix ::operator[](int nIndex) {
assert(nIndex < m_nRow* m_nCol);
return m_pData[nIndex];
}
double& CMatrix ::operator()(int nRow,int nCol) {
assert(nRow*m_nCol+nCol < m_nRow* m_nCol);
return m_pData[nRow * m_nCol + nCol];
}
bool CMatrix::operator==(const CMatrix& m) {
if (!(m_nRow == m.m_nRow && m_nCol == m.m_nCol)) {
return false;
}
for (int i = 0; i < m_nRow * m_nCol; i++) {
if (m_pData[i] != m.m_pData[i]) {
return false;
}
}
return true;
}
bool CMatrix::operator!=(const CMatrix& m) {
return !((*this) == m);
}
CMatrix::operator double() {
double dS = 0;
for (int i = 0; i < m_nRow * m_nCol; i++) {
dS += m_pData[i];
}
return dS;
}
istream& operator>>(istream& is, CMatrix& m) {
is >> m.m_nRow >> m.m_nCol;
m.Create(m.m_nRow, m.m_nCol);
for (int i = 0; i < m.m_nRow*m.m_nCol; i++) {
is >> m.m_pData[i];
}
return is;
}
ostream& operator<<(ostream& os, const CMatrix& m) {
os << m.m_nRow << " " << m.m_nCol << endl;
double* pData = m.m_pData;
for (int i = 0; i < m.m_nRow; i++) {
for (int j = 0; j < m.m_nCol; j++) {
os << *pData++<<" ";
}
os << endl;
}
return os;
}
二、运行结果
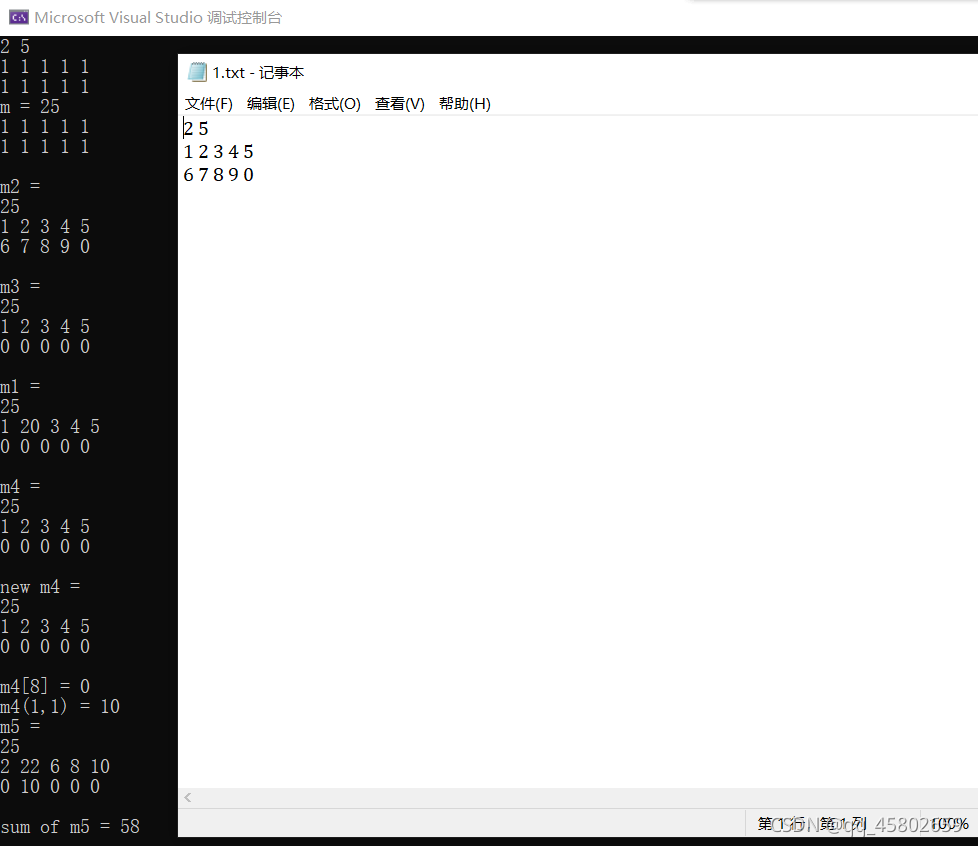
三、函数
1. 构造函数
CMatrix();
CMatrix(int nRow, int nCol, double* pData = NULL);
CMatrix(const CMatrix& m);
CMatrix(const char* strPath);
(1)分类
- 不带参数的构造函数
- 带有参数的构造函数
- 拷贝构造函数
- 带文件路径参数的构造函数
(2)特点
构造函数具有如下几个特点:
- 名字与类名相同,可以有参数,但是不能有返回值(void也不行);
- 作用是对对象进行初始化工作,如给成员变量赋值等;
- 如果定义类时没有写构造函数,系统会生成一个默认的无参构造函数,默认构造函数没有参数,不做任何工作;
- 如果定义了构造函数,系统不再生成默认的无参构造函数;
- 对象生成时构造函数自动调用,对象一旦生成,不能在其上再次执行构造函数
- 一个类可以有多个构造函数,为重载关系。
2. 析构函数
~CMatrix();
- 格式:在类名前加上~;且与构造函数不同的是,析构函数从来没有参数。
- 作用:当对象的生命周期结束时,撤销类对象时候会自动调用析构函数。 1.对象在生命期结束,撤销类对象时会自动调用析构函数。 2.如果用new运算动态地建立一个对象,那用delete运算符释放该对象时,才会调用析构函数。
3. 运算符重载
CMatrix& operator=(const CMatrix& m);
CMatrix& operator+=(const CMatrix& m);
double& operator[](int nIndex);
double& operator()(int nRow, int nCol);
bool operator ==(const CMatrix& m);
bool operator !=(const CMatrix& m);
C++预定义中的运算符的操作对象只局限于基本的内置数据类型,但是对于我们自定义的类型(类)是没有办法操作的。但是大多时候我们需要对我们定义的类型进行类似的运算,这个时候就需要我们对这么运算符进行重新定义,赋予其新的功能,以满足自身的需求。
4. 友元函数
friend istream& operator>>(istream& is, CMatrix& m);
friend ostream& operator<<(ostream& os, const CMatrix& m);
在实现类之间数据共享时,减少系统开销,提高效率。
5. 内联函数
以牺牲代码段空间为代价,提高程序的运行时间的效率(入栈与出栈操作)。
(1)显示
- 放在类外
inline void CMatrix::Set(int nRow, int nCol, double dVal) {
m_pData[nRow * m_nCol + nCol] = dVal;
}
(2)隐式
- 放在类内
void CMatrix::Set(int nRow, int nCol, double dVal) {
m_pData[nRow * m_nCol + nCol] = dVal;
}
|