using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace 重载函数
{
class Program
{
static void Matrix_Mulation(ref double[,] a, ref double[,] b,ref double [,] ab)
{
int m1 = a.GetLength(0);
int n1 = a.GetLength(1);
int m2 = b.GetLength(0);
int n2 = b.GetLength(1);
ab = new double[m1, n2];
if (n1 == m2)
{
for (int i = 0; i < m1; i++)
{
for (int j = 0; j < n2; j++)
{
for (int k = 0; k < n1; k++)
{
ab[i, j] += a[i, k] * b[k, j];
}
}
}
}
else
{
Console.WriteLine("两数组无法相乘");
}
}
static void Matrix_Mulation(ref double n, ref double[,] A, ref double[,] nA)
{
nA = new double[A.GetLength(0), A.GetLength(1)];
for (int i = 0; i < A.GetLength(0); i++)
{
for (int j = 0; j < A.GetLength(1); j++)
{
nA[i, j] = n * A[i, j];
}
}
}
static void OutPut(ref double[,] M)
{
for (int i = 0; i < M.GetLength(0); i++)
{
for (int j = 0; j < M.GetLength(1); j++)
{
Console.Write("{0,6}", M[i, j]);
}
Console.WriteLine();
}
}
static void Main(string[] args)
{
double[,] a = { { 1, 2, 3 }, { 4, 5, 6 }, { 7, 8, 9 } };
double[,] b = { { 1, 2 }, { 4, 5 }, { 7, 8 } };
double[,] ab = { };
double [,] M={};
double n=-1;
double[,] A = { };
double[,] nA = { };
Matrix_Mulation(ref a, ref b, ref ab);
M = ab;
Console.WriteLine("------------两矩阵相乘结果为------------");
Console.WriteLine();
OutPut(ref M);
A = a;
Matrix_Mulation(ref n, ref A, ref nA);
Console.WriteLine("------------两矩阵相乘结果为------------");
Console.WriteLine();
M = nA;
OutPut(ref M);
}
}
}
运行结果如下:
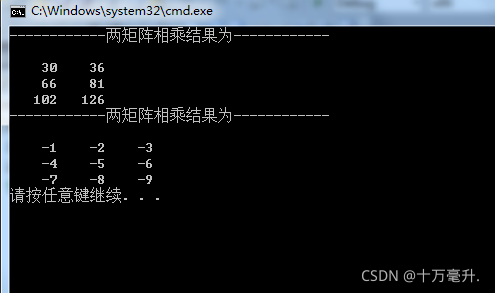
?
|