反转链表 (Reverse Linked List)
题目描述
Given the head of a singly linked list, reverse the list, and return the reversed list.
Example 1: 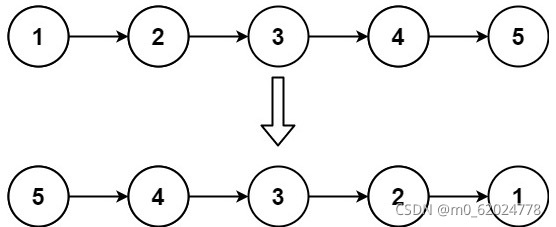
Input: head = [1,2,3,4,5] Output: [5,4,3,2,1]
Example 2:
Input: head = [1,2] Output: [2,1]
Example 3:
Input: head = [] Output: []
Constraints:
- The number of nodes in the list is the range [0, 5000].
- -5000 <= Node.val <= 5000
Follow up: A linked list can be reversed either iteratively or recursively. Could you implement both?
来源:力扣(LeetCode) 链接:https://leetcode-cn.com/problems/reverse-linked-list 著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
解题方法
迭代法
class Solution {
public:
ListNode* reverseList(ListNode* head) {
ListNode *tmp;
ListNode *cur = head;
ListNode *pre = nullptr;
while(cur) {
tmp = cur->next;
cur->next = pre;
pre = cur;
cur = tmp;
}
return pre;
}
};
递归法
class Solution {
public:
ListNode* reverseList(ListNode* head) {
if(head == nullptr || head->next == nullptr) return head;
ListNode *last = reverseList(head->next);
head->next->next = head;
head->next = nullptr;
return last;
}
};
可能出现的问题
runtime error: member access within null pointer of type ‘ListNode’ (solution.cpp)
错误原因:试图使用空指针。 解决方法:增加判断条件,排除对空指针的引用。
|