1. 题目
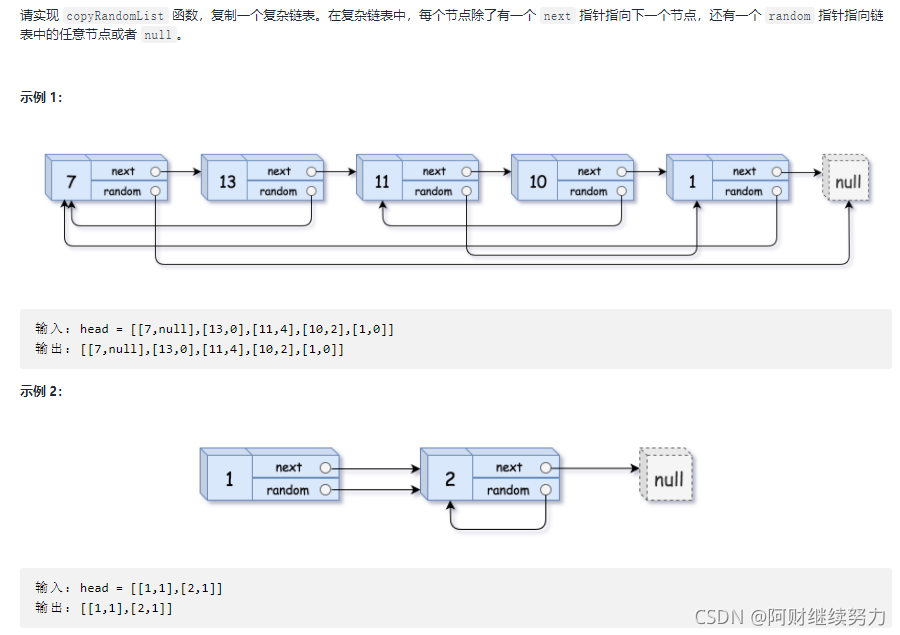 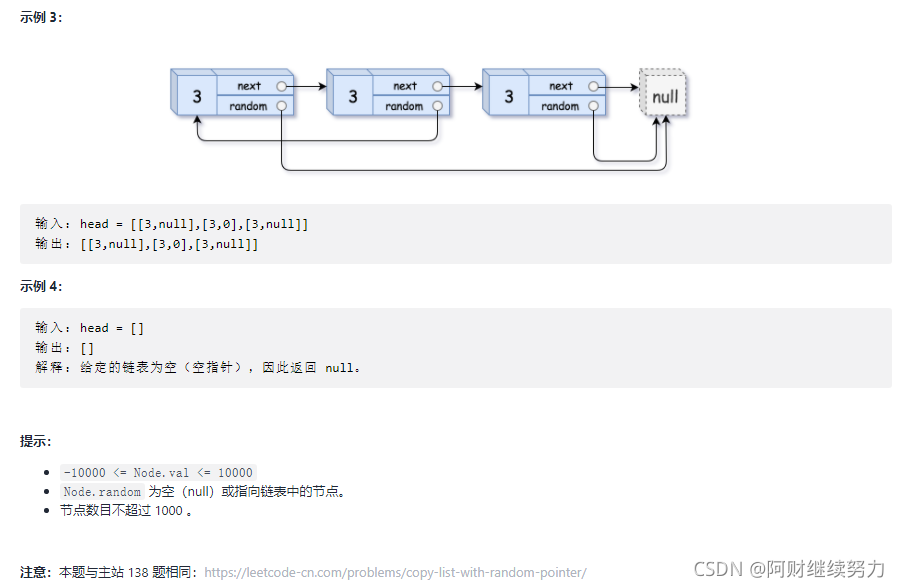
2. 思路
(1) 递归+HashMap
- 利用HashMap存储已创建的结点与原结点的映射关系。
- 递归得到每个结点的next和random,若HashMap中包含该结点对应的新结点,则直接指向新结点即可,否则创建新结点并存入HashMap中。
(2) 迭代
- 首先遍历链表,创建的新结点插入原结点的后面,然后再遍历链表,根据原结点的random修改新结点的random,最后拆分两个链表,返回新链表即可。
3. 代码
import java.util.HashMap;
import java.util.Map;
public class Test {
public static void main(String[] args) {
}
}
class Node {
int val;
Node next;
Node random;
public Node(int val) {
this.val = val;
this.next = null;
this.random = null;
}
}
class Solution {
private Map<Node, Node> map = new HashMap<>();
public Node copyRandomList(Node head) {
if (head == null) {
return null;
}
if (map.containsKey(head)) {
return map.get(head);
}
Node newHead = new Node(head.val);
map.put(head, newHead);
newHead.next = copyRandomList(head.next);
newHead.random = copyRandomList(head.random);
return newHead;
}
}
class Solution1 {
public Node copyRandomList(Node head) {
if (head == null) {
return null;
}
Node pre = head;
Node cur;
while (pre != null) {
cur = new Node(pre.val);
cur.next = pre.next;
pre.next = cur;
pre = cur.next;
}
pre = head;
while (pre != null) {
cur = pre.next;
if (pre.random != null) {
cur.random = pre.random.next;
}
pre = cur.next;
}
Node newHead = head.next;
pre = head;
while (pre != null) {
cur = pre.next;
pre.next = cur.next;
if (pre.next != null) {
cur.next = pre.next.next;
} else {
cur.next = null;
}
pre = pre.next;
}
return newHead;
}
}
|