栈和队列常考面试题
面试题1:有效的括号(括号匹配问题)
LeetCode-20有效的括号
解题思路分析如图:
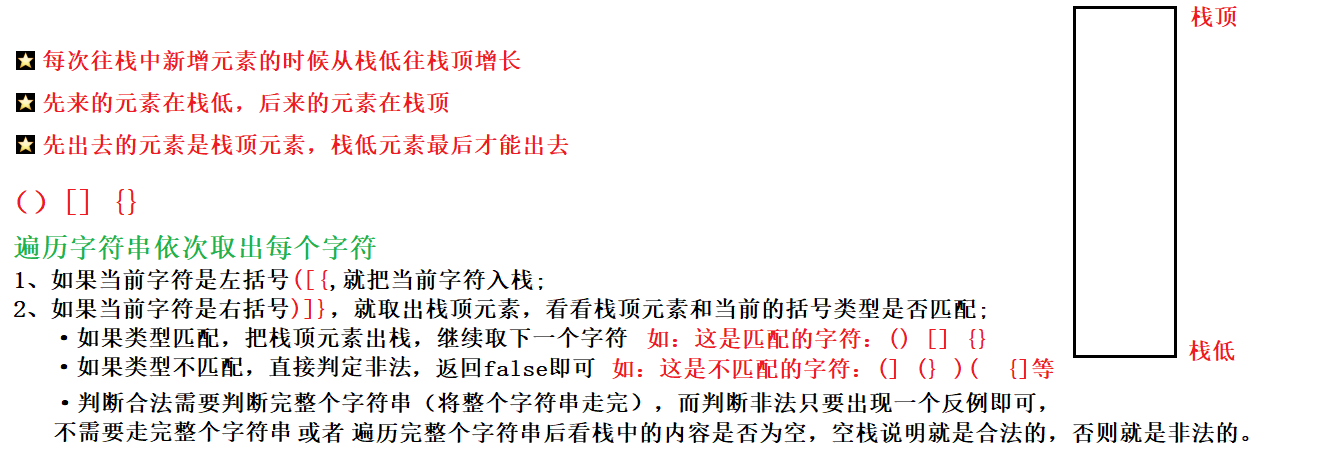
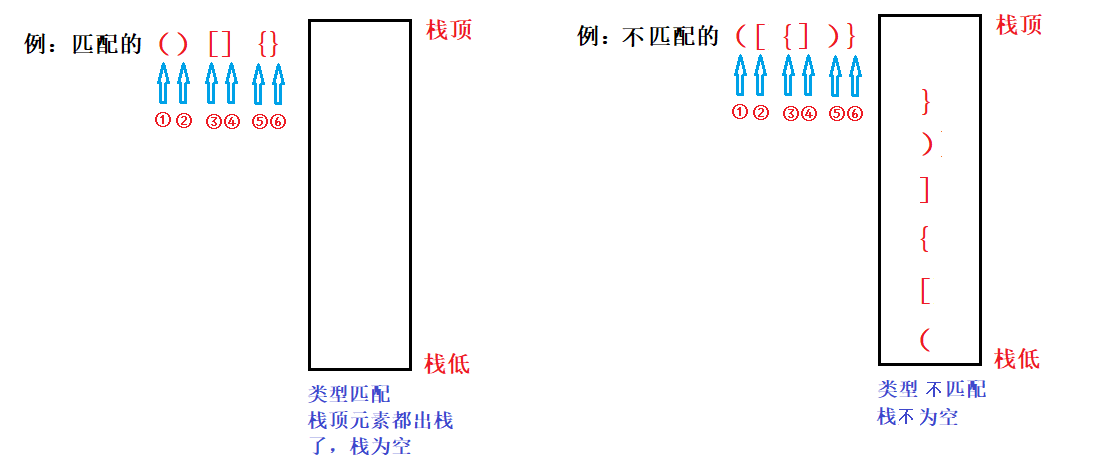
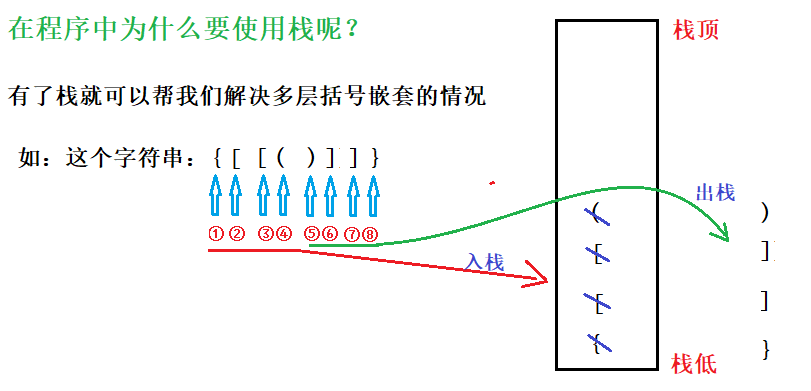
代码示例1:
package java2021_1004;
import java.util.Stack;
public class StackAndQueueInterviewQuestion1 {
public boolean isValid(String s) {
Stack<Character> stack=new Stack<>();
for(int i=0;i<s.length();i++) {
char c = s.charAt(i);
if (c == '(' || c == '[' || c == '{') {
stack.push(c);
continue;
}
if(stack.empty()){
return false;
}
Character top=stack.pop();
if(top=='(' && c==')'){
continue;
}
if(top=='[' && c==']'){
continue;
}
if(top=='{' && c=='}'){
continue;
}
return false;
}
if(stack.empty()){
return true;
}
return false;
}
}
代码示例2:将代码示例1中的合法情况1、2、3改成使用Map
package java2021_1004;
import java.util.*;
public class UseMap {
public boolean isValid(String s) {
Map<Character,Character> map=new HashMap<>();
map.put('(',')');
map.put('[',']');
map.put('{','}');
Stack<Character> stack=new Stack<>();
for(int i=0;i<s.length();i++) {
char c = s.charAt(i);
if (c == '(' || c == '[' || c == '{') {
stack.push(c);
continue;
}
if(stack.empty()){
return false;
}
Character top=stack.pop();
if(map.get(top)==c){
continue;
}
return false;
}
if(stack.empty()){
return true;
}
return false;
}
}
面试题2:用队列实现栈
LeetCode-225用队列实现栈
疑问:基于队列实现栈该如何实现呢?
基本过程分析:
可以使用两个队列来模拟实现一个栈的效果,因为,队列是先进先出,栈是先进后出,要想用队列实现栈需要实现先进后出的操作,在一个队列中是无法让先进来的后出去的,所以此时就需要一个辅助队列来帮助完成这样的操作,将A队列中除最后一个元素外都倒腾到B队列中,此时就可以让后进来的先出去了。
- 入栈:把新的元素往A队列中入队即可
- 出栈:把A队列中的元素往B队列中倒腾(即把A队列中的元素依次出队列,出队列之后入到B队列,直到当A中就剩一个元素的时候,就不用入到B队列中了,直接出队列即可,不需要计数了,此时就把最后进来的这个元素删掉了。)
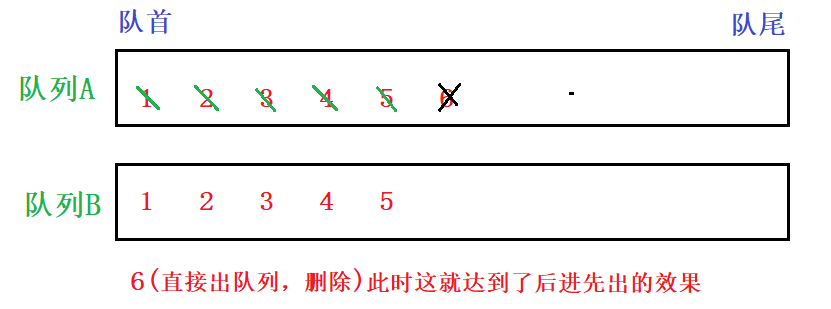
◇完成以上操作之后,交换A队列和B队列的身份,让A队列始终保持是入队列的(即保证新入栈的元素始终是往A中入)。 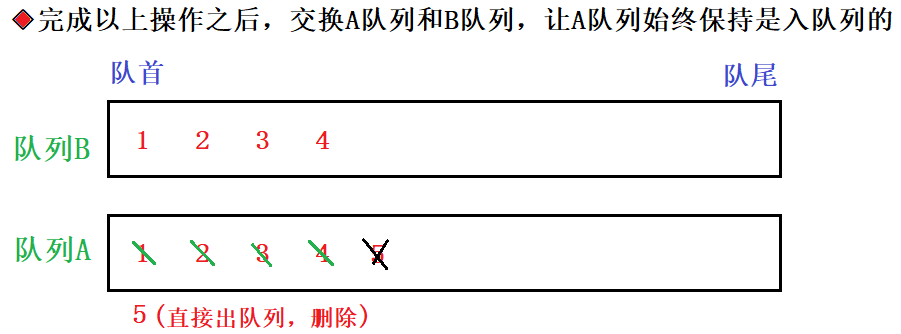 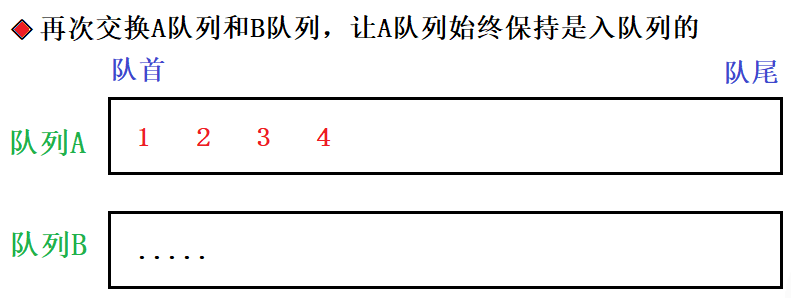
- 取栈顶元素:和出栈类似,但是出栈的时候是把最后一个元素给删掉,而取栈顶元素的最后一个元素是不能删的
- 判定为空:当A和B都为空,此时就表明这个栈为空。
package java2021_1004;
import java.util.Collection;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.Queue;
public class StackAndQueueInterviewQuestion2 {
private Queue<Integer> A=new LinkedList<>();
private Queue<Integer> B=new LinkedList<>();
public void push(int x){
A.offer(x);
}
public Integer pop(){
if(empty()){
return null;
}
while(A.size()>1){
Integer front=A.poll();
B.offer(front);
}
int ret=A.poll();
swapAB();
return ret;
}
private void swapAB(){
Queue<Integer> tmp=A;
A=B;
B=tmp;
}
public Integer top(){
if(empty()){
return null;
}
while(A.size()>1){
Integer front=A.poll();
B.offer(front);
}
int ret=A.poll();
B.offer(ret);
swapAB();
return ret;
}
public boolean empty(){
return A.isEmpty();
}
}
面试题3:用栈实现队列
LeetCode-232用栈实现队列
疑问:基于栈实现队列该如何实现呢?
可以使用两个栈来模拟实现队列的效果,
1、实现入队列:先把B中的所有元素倒腾到A中,然后直接往A中入栈即可。
2、实现出队列:先把A中的所有元素都倒腾到B中,然后对B进行出栈操作即可
3、去队首元素,先把A中的所有元素都倒腾到B中,然后取B的栈顶元素就是队首元素。
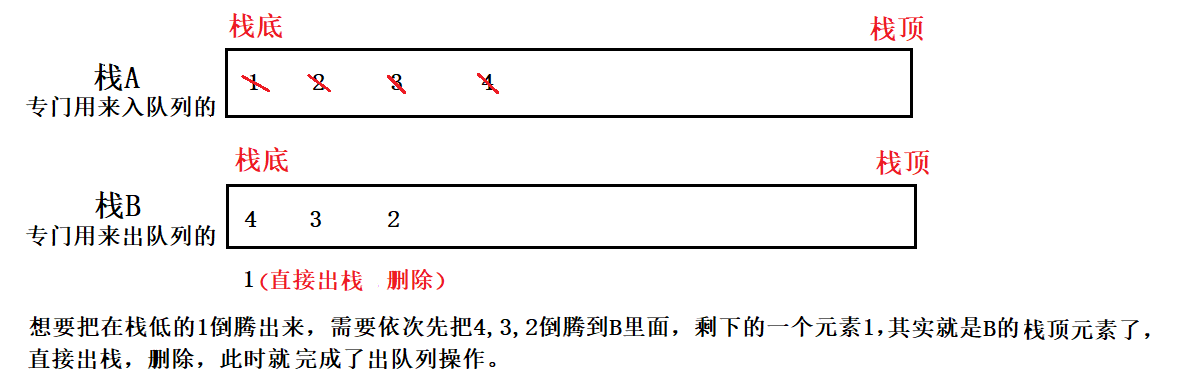
4、判空:A和B都为空,整个队列就是空了。
代码示例:
package java2021_1004;
import java.util.Stack;
public class StackAndQueueInterviewQuestion3 {
private Stack<Integer> A=new Stack<>();
private Stack<Integer> B=new Stack<>();
public void push(int x){
while(!B.isEmpty()){
int tmp=B.pop();
A.push(tmp);
}
A.push(x);
}
public Integer pop(){
if(empty()){
return null;
}
while(!A.isEmpty()){
int tmp=A.pop();
B.push(tmp);
}
return B.pop();
}
public Integer peek(){
if(empty()){
return null;
}
while(!A.isEmpty()){
int tmp=A.pop();
B.push(tmp);
}
return B.peek();
}
public Boolean empty(){
return A.isEmpty() && B.isEmpty();
}
}
面试题4:实现一个最小栈
LeetCode-115最小栈
问题描述: 设计一个支持 push ,pop ,top 操作,并能在常数时间内检索到最小元素的栈。
push(x) —— 将元素 x 推入栈中。 pop() —— 删除栈顶的元素。 top() —— 获取栈顶元素。 getMin() —— 检索栈中的最小元素。
- 像最小栈这样的问题,要求O(1)获取最小值,只能用空间换时间。
- 创建两个栈一个叫栈A,一个叫栈B
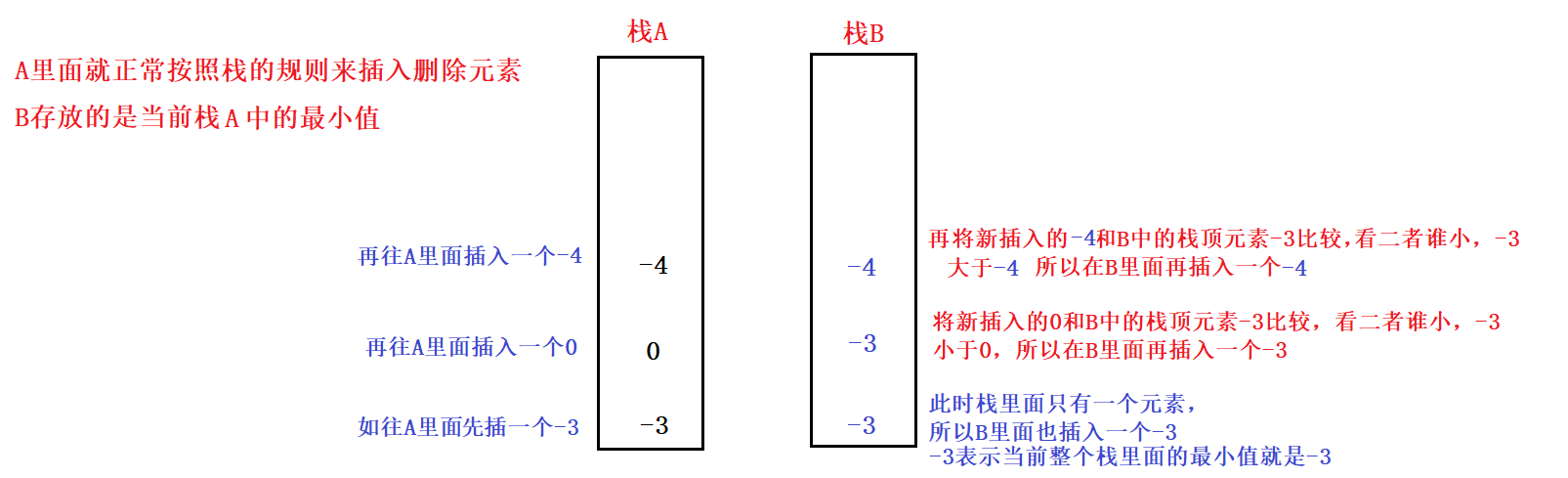

- 实现入栈操作:对于A,直接入栈,对于B,取当前元素和B的栈顶元素比较,把较小值入栈。
- 实现出栈操作:对于栈A和栈B同时出栈
- 实现取栈顶元素:直接取A的栈顶元素
- 实现取最小值:直接取B的栈顶元素
代码:
package java2021_1004;
import java.util.Stack;
public class StackAndQueueInterviewQuestion4 {
private Stack<Integer> A=new Stack<>();
private Stack<Integer> B=new Stack<>();
public void push(int x){
A.push(x);
if(B.isEmpty()){
B.push(x);
return;
}
int min=B.peek();
if(x<min){
min=x;
}
B.push(min);
}
public Integer pop(){
if(A.isEmpty()){
return null;
}
B.pop();
return A.pop();
}
public Integer top(){
if(A.isEmpty()){
return null;
}
return A.peek();
}
public Integer getMin(){
if(B.isEmpty()){
return null;
}
return B.peek();
}
}
面试题5:设计循环队列
LeetCode-622设计循环队列
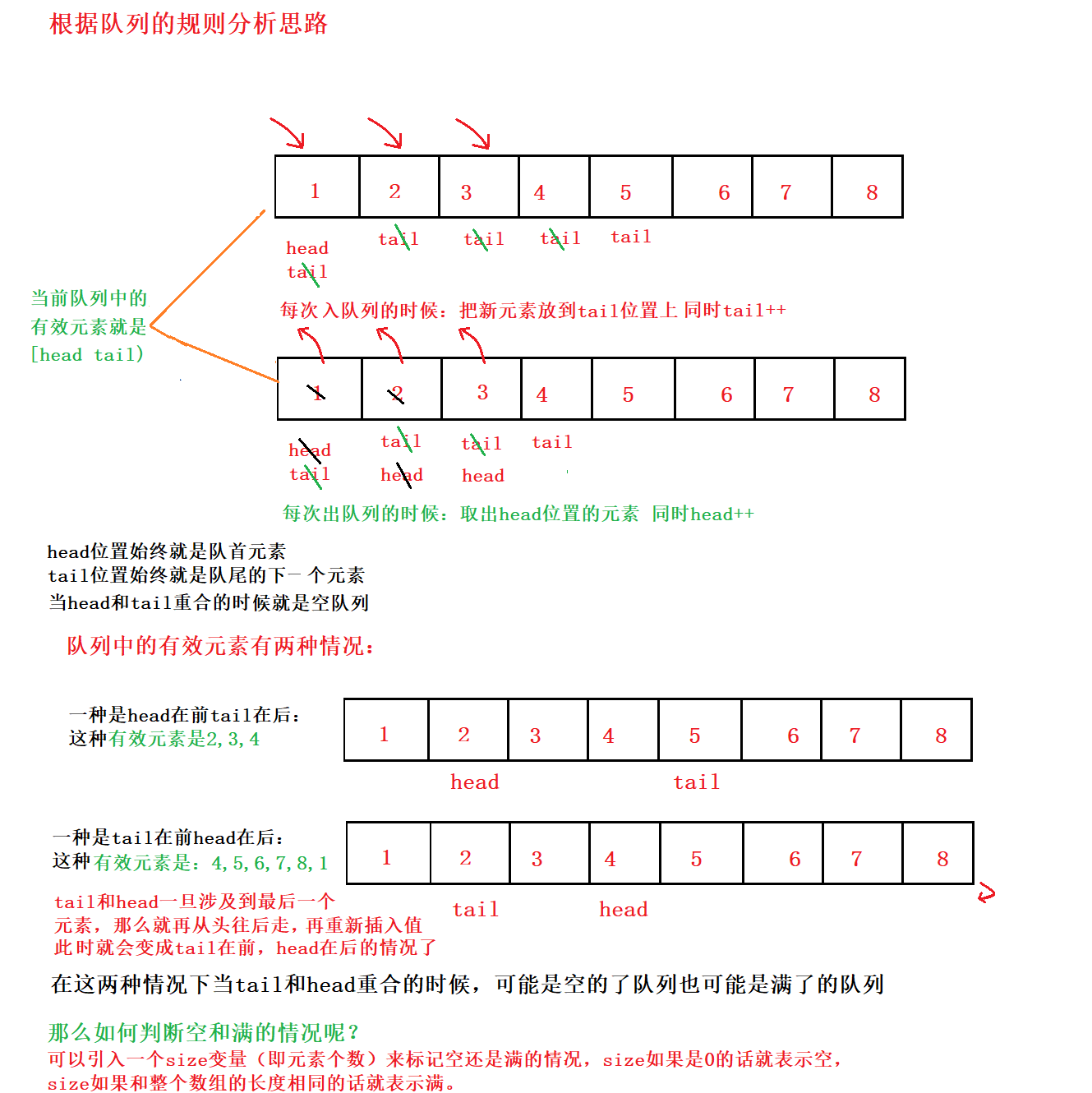
package java2021_1003;
public class MyQueueArray {
private int[] array=new int[100];
private int head=0;
private int tail=0;
private int size=0;
public void offer(int val){
if(size==array.length){
return;
}
array[tail]=val;
tail++;
if(tail>=array.length) {
tail=0;
}
size++;
}
public Integer poll(){
if(size==0){
return null;
}
Integer ret=array[head];
head++;
if(head>=array.length){
head=0;
}
size--;
return ret;
}
public Integer peek(){
if(size==0){
return null;
}
return array[head];
}
}
|