以先序遍历为例创建二叉树以及求叶子节点的个数?
测试:ABD#G###CE##F##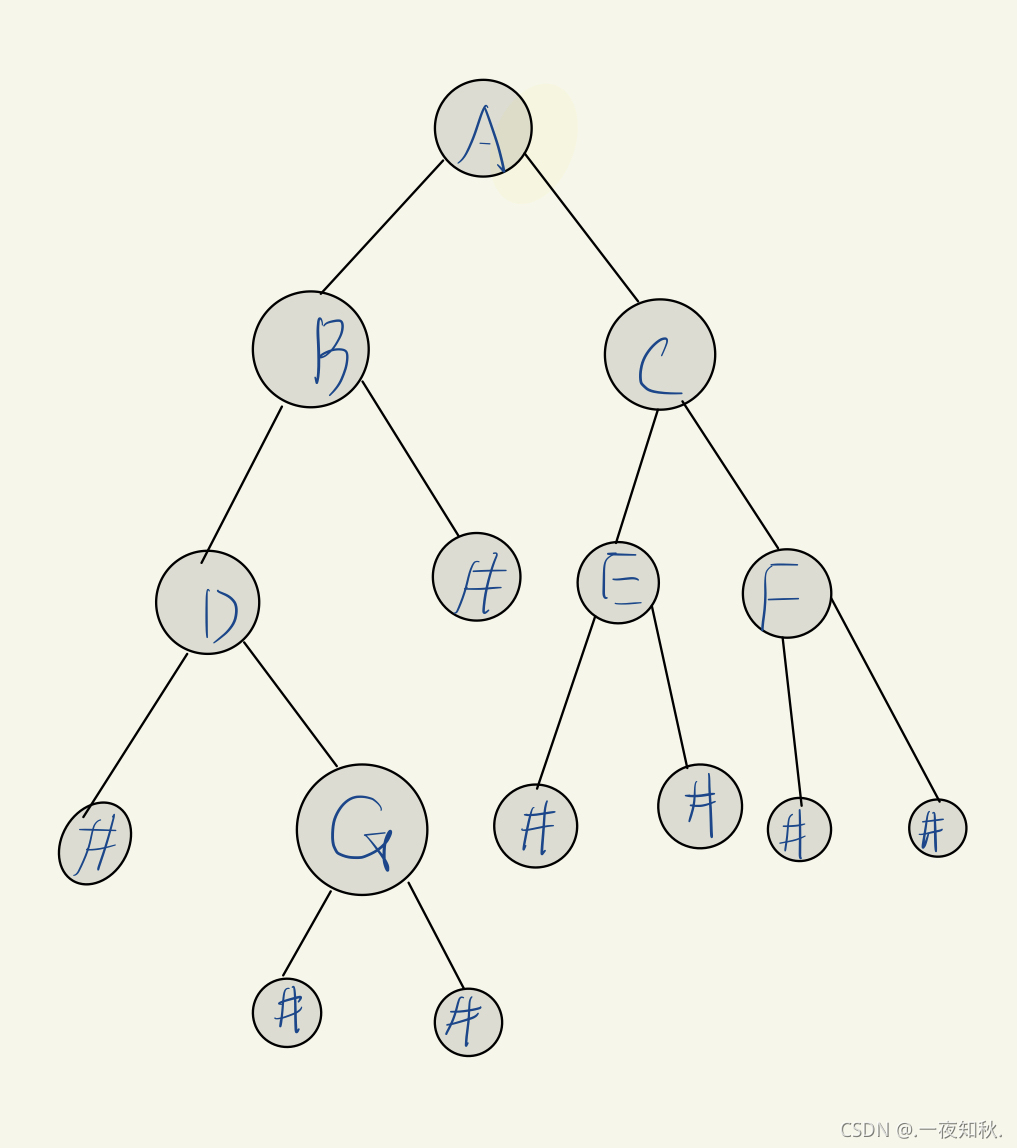
//编写非递归算法,求二叉树中叶子结点的个数
#include<stdio.h>
#include<stdlib.h>
#define MaxSize 1000
typedef struct BiTNode
{
char data;
struct BiTNode* lchild;
struct BiTNode* rchild;
}BiTNode,*BiTree;
//创建二叉树---先序创建二叉树 这里用的先序遍历树应该输入ABD#G###CE##F##
void CreateBiTree(BiTree &T)
{
char ch;
scanf("%c",&ch);
if(ch == '#')
{
T=NULL;
}
else{
T = (BiTree)malloc(sizeof(BiTNode));
T->data = ch;
CreateBiTree(T->lchild);
CreateBiTree(T->rchild);
}
return ;
}
//先序遍历算法---非递归求二叉树中叶子结点的个数
int Count_BiTree0(BiTree T)
{
int top=-1; //此时栈为空
int count =0;
BiTree S[MaxSize];
while(T!=NULL || top != -1)
{
while (T != NULL)
{
if(T->lchild == NULL && T->rchild == NULL)
{
count++;
}
S[++top] = T; //++top(top初始为-1),then将当前的根节点入栈
T = T->lchild; //访问当前根节点的左子树
}
if(top != -1)
{
T = S[top--]; //获取当前的根节点
T = T->rchild; //访问当前根节点的右子树
}
}
return count;
}
int main()
{
BiTree T;
CreateBiTree(T);
printf("the leaf Node 0 is:%d\n",Count_BiTree0(T));
return 0;
}
|