思路:
将二叉树的叶子结点转换成单链表,并返回最左叶子结点的地址(链头))
即可用先序遍历 找到结点并且将其连接连接成链表就可以了
此处先序遍历建立的二叉树为下如所示:(ABD#G###CE##F##)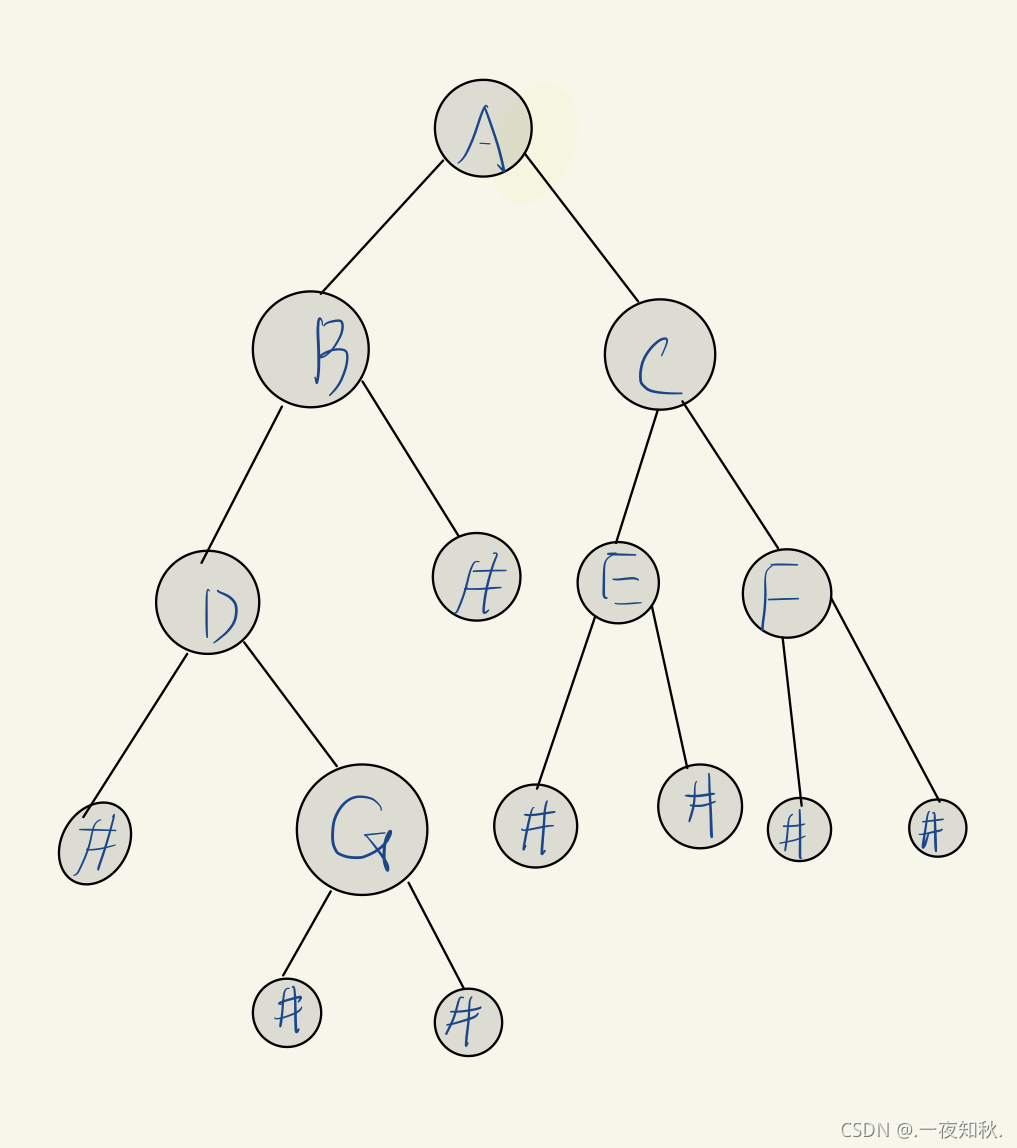
//---编写一个递归算法,利用叶结点中空的指针域,将空结点自左向右连接成一个链表---//
//二叉链表 存储表示
#include<stdio.h>
#include<stdlib.h>
typedef struct BiTNode
{
char data;
struct BiTNode *lchild,*rchild;
struct BiTNode *next;
}BiTNode,*BiTree;
void get_list(BiTree T,BiTree &L) //获得链表
{
if(!T) //判断T是否为空
{
return ;
}
else
{
if(T->lchild == NULL && T->rchild == NULL) //找到叶子结点
{
BiTree t=L;
while(t->next!=NULL) //找到链尾
{
t=t->next;
}
t->next = T; //连接链表
t->next->next=NULL;
return;
}
get_list(T->lchild,L); //遍历左子树
get_list(T->rchild,L); //遍历右子树
}
return ;
}
void PrintList(BiTree L) //打印链表
{
BiTree t=L->next;
while(t != NULL)
{
printf("%c",t->data);
t = t->next;
}
return ;
}
//创建二叉树 先序遍历 可以输入ABD#G###CE##F##
void CreateBiTree(BiTree &T)
{
char ch;
scanf("%c",&ch);
if(ch == '#')
{
T = NULL;
}
else{
T=(BiTree)malloc(sizeof(BiTNode));
T->data=ch;
CreateBiTree(T->lchild);
CreateBiTree(T->rchild);
}
return ;
}
int main()
{
BiTree L;
L=(BiTree)malloc(sizeof(BiTNode));
L->next =NULL;
BiTree T;
CreateBiTree(T);
get_list(T,L);
PrintList(L);
return 0;
}
|