链表有很多好处,相比较顺序表而言可以节省空间
以下实现单链表的基本操作
import java.util.List;
class ListNode{
public int val;
public ListNode next;
public ListNode(int val){
this.val = val;
}
}
public class MyLinkedList1 {
public ListNode head;
//打印单链表
public void show(){
ListNode cur = this.head;
while(cur!=null){
System.out.print(cur.val+" ");
cur = cur.next;
}
System.out.println();
}
//计算单链表的长度
public int getSize(){
ListNode cur = this.head;
int count = 0;
while(cur!=null){
count++;
cur = cur.next;
}
return count;
}
//头插法
public void addFirst(int data){
//将给定的数据节点化 val值为给定数据,次时next为null
ListNode node = new ListNode(data);
//将node.next存头结点的地址
node.next = this.head;
//头结点的引用指向node
this.head = node;
}
//尾插法
public void addLast(int data){
ListNode node = new ListNode(data);
if(this.head==null){//判断单链表是否为空
this.head=node;//空 直接插入
return;
}
ListNode cur = this.head;
while(cur.next!=null){
cur = cur.next;
}//循环:查找尾节点
cur.next=node;
}
//查找是否包含关键字key是否在单链表当中
public boolean contains(int key){
if (this.head==null){
System.out.println("该链表为空!");
return false;
}
ListNode cur = this.head;
while(cur!=null){
if (cur.val==key){
return true;
}
cur = cur.next;
}
return false;
}
//任意位置插入,第一个数据节点为0号下标
public void addIndex(int index,int data){
if (index<0||this.head==null){
System.out.println("index位置不合法");
return;
}
ListNode node = new ListNode(data);//数据节点化
if (index==0){
addFirst(data);//头插法
}
if (index==getSize()){
addLast(data);//尾插法
}else{
ListNode cur = indexSubOne1(index);//找到要插入节点的前一节点
node.next = cur.next;//先连接后一节点
cur.next = node;//在连接前一节点
}
}
//找到index-1的节点 为 任意位置插入,第一个数据节点为0号下标 方法服务
public ListNode indexSubOne1(int index){
ListNode cur = this.head;
while(index-1!=0) {
cur = cur.next;
index--;
}
return cur;
}
//删除第一次出现的key节点
public void remove(int key){
if (this.head==null){
System.out.println("该链表为空!");
return;
}
if (this.head.val==key){//头结点为要删除节点
this.head = this.head.next;
}
ListNode prev = searchPrev(key);
if (prev==null){
System.out.println("没有你要删的节点");
return;
}
prev.next = prev.next.next;//删除节点
}
//找要删除节点的前一节点
private ListNode searchPrev(int key){
ListNode cur = this.head;
while(cur.next!=null){
if (cur.next.val==key) {
return cur;
}
cur = cur.next;
}
return null;
}
//删除所有key节点
public void removeAllKey(int key){
ListNode cur = this.head.next;
ListNode prev = this.head;
while(cur!=null){
if (cur.val==key){
prev.next = cur.next;//删节点
cur = cur.next;//遍历下一个节点
}else{
prev = cur;//移动
cur = cur.next;
}
}
if (this.head.val==key){
this.head = this.head.next;
}
}
//清空一个链表
public void clear(){ //稍微粗暴
this.head = null;
System.out.println("清空链表");//若打印 则clear调用成功
}
}
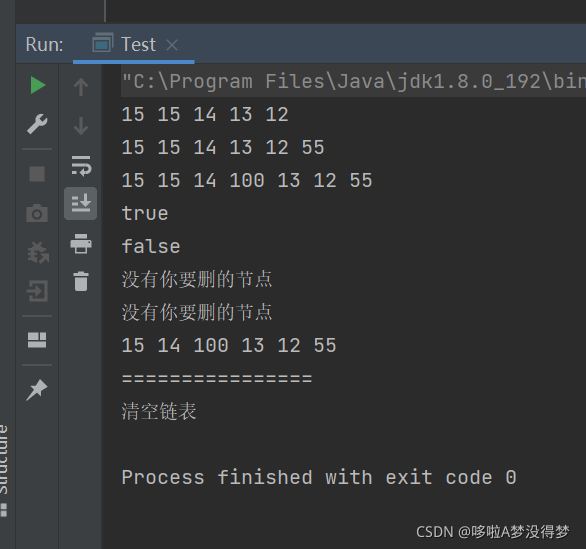
|