先上结果图:
?
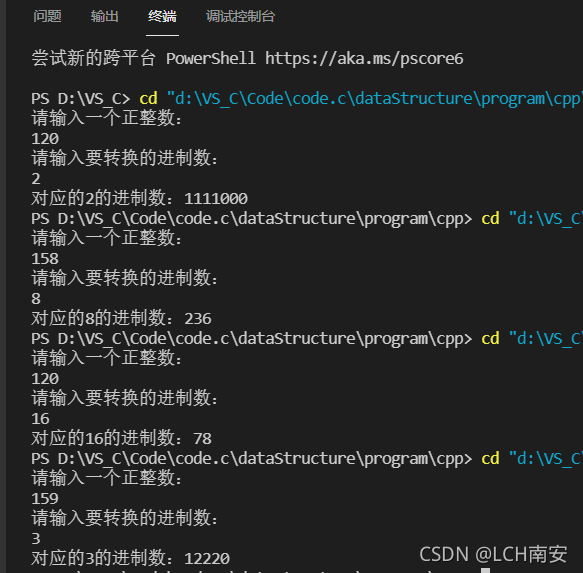
?代码部分:
/*
Author:LCH南安
Time:2021.10.25
*/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define STACK_INIT_SIZE 100
#define MaxSize 100; //可用最大容量
typedef char ElemType; //定义为char类型
typedef struct SqStack
{
ElemType *base; //栈底指针
int top; //栈顶
int stacksize; //栈可用最大容量
} SqStack;
//构造一个空的栈
void InitStack(SqStack &S)
{ //分配空间大小为STACK_INIT_SIZE的栈
S.base = (ElemType *)malloc(STACK_INIT_SIZE * sizeof(ElemType));
if (!S.base)
exit(0); // 存储分配失败
S.top = -1; //栈空
S.stacksize = MaxSize; //栈可用最大容量为100
}
//元素进栈
int Push(SqStack &S, ElemType e)
{
if (S.top >= S.stacksize - 1) //判断是否溢出
{
//S.base=(ElemType *)realloc(S.base,(S.stacksize+STACK_INIT_SIZE)*sizeof(ElemType));
//if(!S.base) exit();
//S.top=S.base+S.stacksize; //由于realloc可能不在原malloc分配的起始地址开始,
//可能从另一足够大分区开始重新分配内存,所以必须更新top;
//S.stacksize+=STACK_INIT_SIZE
return 0;
}
S.top++;
S.base[S.top] = e; //进栈
return 1;
}
//元素出栈
int Pop(SqStack &S, ElemType &e)
{
if (S.top == -1) //判断是否栈空
{
return 0;
}
else
{
e = S.base[S.top]; //出栈,e接收
S.top--; //栈顶降低
return 1;
}
}
//判断栈是否为空
int StackEmpty(SqStack S)
{
if (S.top == -1) //判断是否栈空
return 1;
else
return 0;
}
//销毁顺序栈
int DestroyStack(SqStack &S)
{
if (S.base)
{
delete S.base;
S.stacksize = 0; //最大容量变为0
S.top = -1; //栈顶标志
S.base = NULL; //栈底指针为NULL
}
return 1;
}
//进制转换
void trans(int d, int c, char b[])
{
SqStack S;
InitStack(S);
char ch;
int i = 0;
while (d != 0)
{
ch = '0' + d % c; //求余数并转为字符
Push(S, ch); //进栈
d /= c; //继续下一位
}
while (!StackEmpty(S)) //判栈空
{
Pop(S, ch); //出栈
b[i] = ch; //将出栈元素数组
i++;
}
b[i] = '\0'; //加入字符串结束标志
DestroyStack(S); //销毁S
}
int main()
{
int d, c;
char str[100];
do
{
printf("请输入一个正整数:\n"); //保证输入正整数
scanf("%d", &d);
printf("请输入要转换的进制数:\n");
scanf("%d", &c);
} while (d < 0);
trans(d, c, str); //传参
printf("对应的%d的进制数:%s\n", c, str);
return 0;
}
|